Method Chaining in TypeScript: Best Practices and Examples
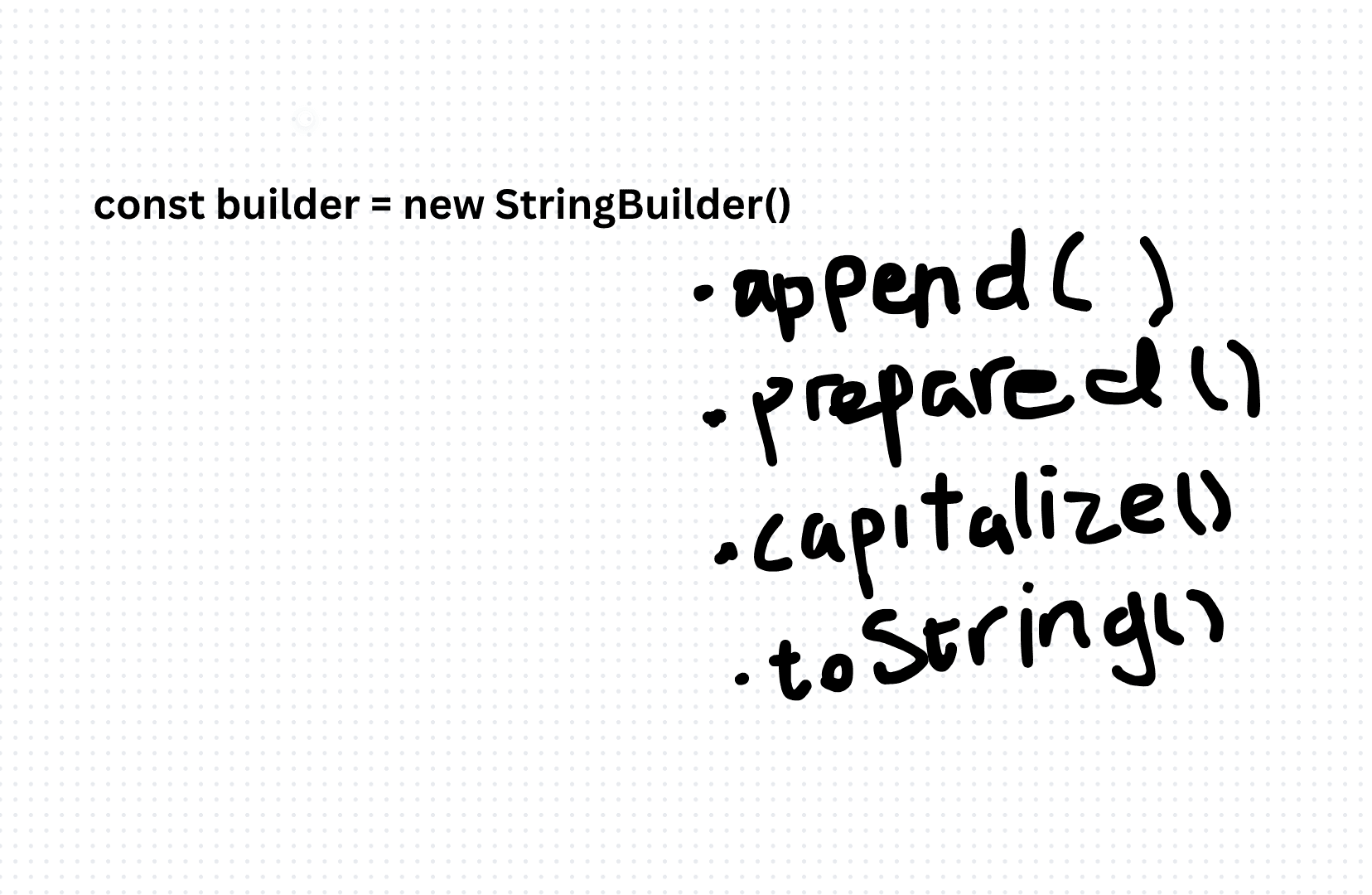
Method chaining is a powerful technique in object-oriented programming that allows consecutive method calls on an object in a fluent and concise manner. This pattern enhances code readability and maintainability by reducing the need for temporary variables and improving code expressiveness. In this article, we'll explore best practices for method chaining in TypeScript, along with examples to demonstrate its usage and benefits.
class StringBuilder {
private value: string;
constructor(value: string = '') {
this.value = value;
}
append(text: string): StringBuilder {
this.value += text;
return this;
}
prepend(text: string): StringBuilder {
this.value = text + this.value;
return this;
}
capitalize(): StringBuilder {
this.value = this.value.charAt(0).toUpperCase() + this.value.slice(1);
return this;
}
toString(): string {
return this.value;
}
}
const builder = new StringBuilder()
.append('hello, ')
.prepend('Hi, ')
.capitalize();
console.log(builder.toString());
Method chaining is a versatile technique for building fluent and expressive APIs in TypeScript. By adhering to best practices such as returning this, chaining immutable operations, documenting support, avoiding side effects, and using method chaining judiciously, developers can harness the power of method chaining to write clean, readable, and maintainable code.