Toy Robot Simulator with Test-Driven Development (TDD) in TypeScript
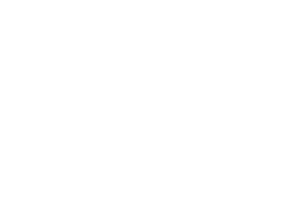
Before we begin, make sure you have Node.js and npm installed on your machine. Create a new directory for your project and initialize a new TypeScript.
You can clone my project here
git clone git@github.com:ehsangazar/toy-robot.git
Implementing the Robot Functionality:
Now that we have our initial test passing, let's implement the functionality of our Toy Robot Simulator. We'll start by adding basic functionality such as placing the robot on the table, moving it, rotating it, and checking its position.
You want to write the generic tests first:
import Board from "../Board";
import Cell from "../Cell";
import Robot, { Direction } from "../Robot";
describe("Cell", () => {
it("should return a function", () => {
expect(typeof Cell).toBeTruthy();
});
it("should return x and y", () => {
const cell = new Cell(0, 0);
const x = cell.getX();
const y = cell.getY();
expect(x).toBe(0);
expect(y).toBe(0);
});
it("should be able to occupy", () => {
const cell = new Cell(0, 0);
const board = new Board(10);
const robot = new Robot(0, 0, board, Direction.UP);
cell.occupy(robot);
expect(cell.getOccupant()).toBeTruthy();
});
it("should be able return if it is occupied", () => {
const cell = new Cell(0, 0);
const board = new Board(10);
const robot = new Robot(0, 0, board, Direction.UP);
cell.occupy(robot);
expect(cell.isItOccupied()).toBe(true);
});
it("should be able to free itself", () => {
const cell = new Cell(0, 0);
const board = new Board(10);
const robot = new Robot(0, 0, board, Direction.UP);
cell.occupy(robot);
cell.free();
expect(cell.isItOccupied()).toBe(false);
expect(cell.getOccupant()).toBe(null);
});
});
And After that you want to pass the tests:
import Robot from "../models/Robot";
class Cell {
private x: number;
private y: number;
private isOccupied: boolean = false;
private occupant: Robot | null = null;
constructor(x: number, y: number) {
this.x = x;
this.y = y;
}
getX() {
return this.x;
}
getY() {
return this.y;
}
occupy(newOccupant: Robot) {
this.isOccupied = true;
this.occupant = newOccupant;
}
getOccupant() {
return this.occupant;
}
free() {
this.isOccupied = false;
this.occupant = null;
}
isItOccupied() {
return this.isOccupied;
}
}
export default Cell;
Continuing with TDD:
As we implement each new feature, we'll follow the TDD cycle:
- Write a failing test that describes the desired behavior.
- Write the minimal amount of code necessary to make the test pass.
- Refactor the code as needed while keeping all tests passing.
Conclusion:
In this tutorial, we've built a Toy Robot Simulator using Test-Driven Development (TDD) in TypeScript. We've followed the TDD approach to iteratively develop the functionality of our robot, ensuring that each component is thoroughly tested before moving on to the next. By adopting TDD, we've created a robust and maintainable codebase with a comprehensive test suite. Happy coding!