Palindrome Algorithm
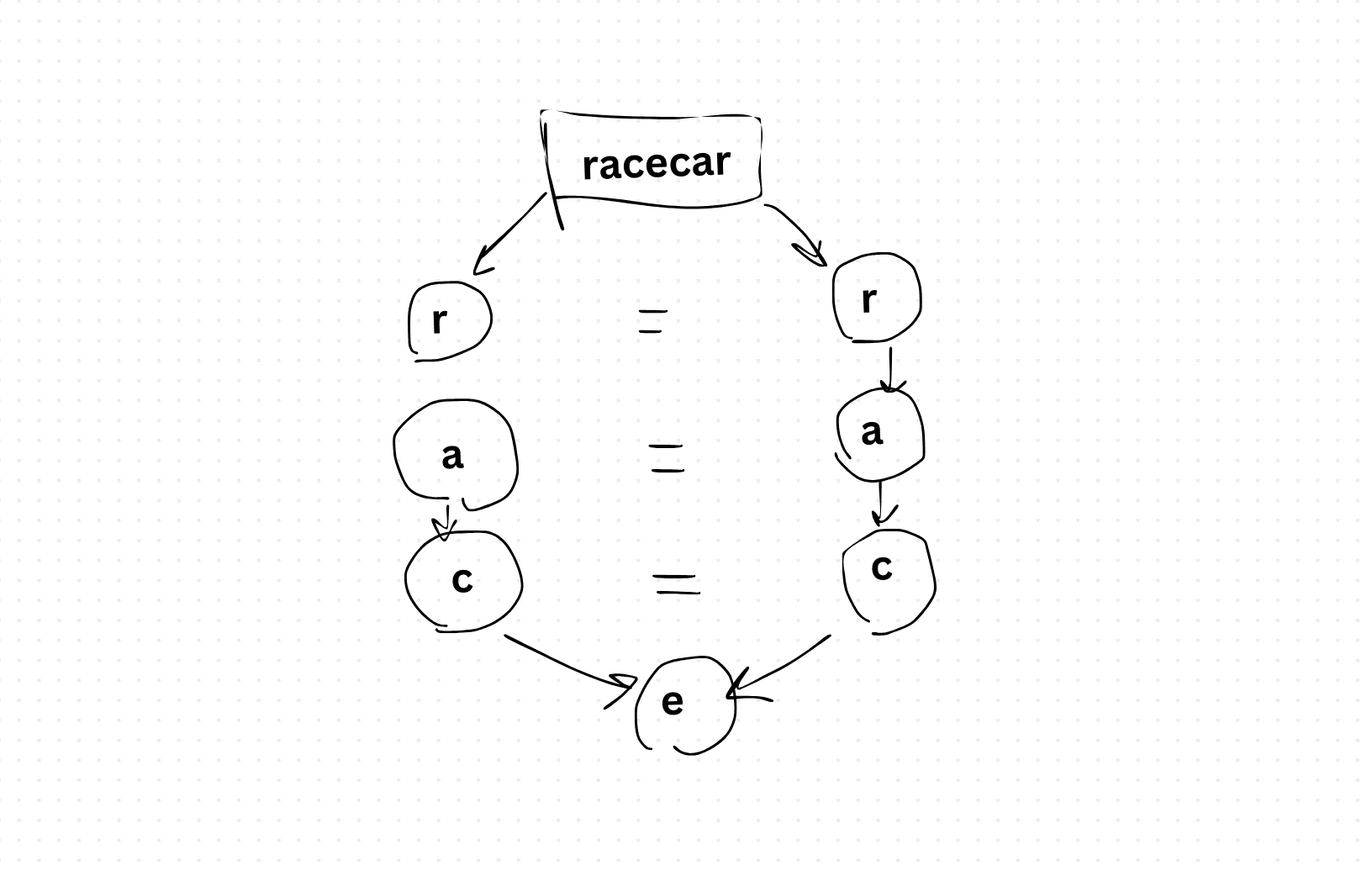
A palindrome is a sequence of characters that remains unchanged when read forwards or backwards, disregarding spaces, punctuation, and capitalization. Examples include "racecar," "madam," and "A man, a plan, a canal, Panama!" Understanding and detecting palindromes involves analyzing the structural symmetry of the sequence, often with an emphasis on efficient algorithmic approaches.
The Palindrome algorithm aims to determine whether a given string or sequence is a palindrome. Several techniques exist to solve this problem, each with its trade-offs in terms of time complexity, space complexity, and implementation complexity.
Brute-Force Method:
The simplest approach involves comparing the string with its reversed version. If the original and reversed strings are identical, the sequence is a palindrome. While intuitive, this method has a time complexity of O(n^2), where n is the length of the string, due to the overhead of string concatenation and comparison.
Ignoring Non-Alphanumeric Characters:
To simplify the comparison process, we can ignore non-alphanumeric characters and focus solely on alphanumeric content. This approach enhances the robustness of the algorithm and ensures consistent behaviour across different inputs.
Case-Insensitive Comparison:
To handle variations in capitalization, we can convert all characters to lowercase or uppercase before comparison. This ensures that the algorithm detects palindromes regardless of case differences.
Let's Code it Up!
const isPalindrome = (input) => {
const newInput = String(input).toLowerCase().replace(/[^a-z0-9]/g,"");
let leftIndex = 0;
let rightIndex = newInput.length - 1
while (leftIndex < rightIndex){
if (newInput[leftIndex] !== newInput[rightIndex]) {
return false;
}
leftIndex += 1;
rightIndex -= 1;
}
return true
}
isPalindrome('racecar');
isPalindrome('gazar');
isPalindrome(137283232);
isPalindrome(123321);
Conclusion:
The Palindrome algorithm, with its blend of simplicity and significance, embodies the essence of algorithmic problem-solving. By understanding its underlying principles, exploring implementation techniques, and optimizing detection strategies, developers can unlock a wealth of opportunities in various domains, from text processing and data validation to algorithmic puzzles and beyond. So, whether you're deciphering palindrome phrases or optimizing detection algorithms, embrace the challenge and let the power of palindromes guide your journey through the fascinating world of computer science.