Hashing Algorithm
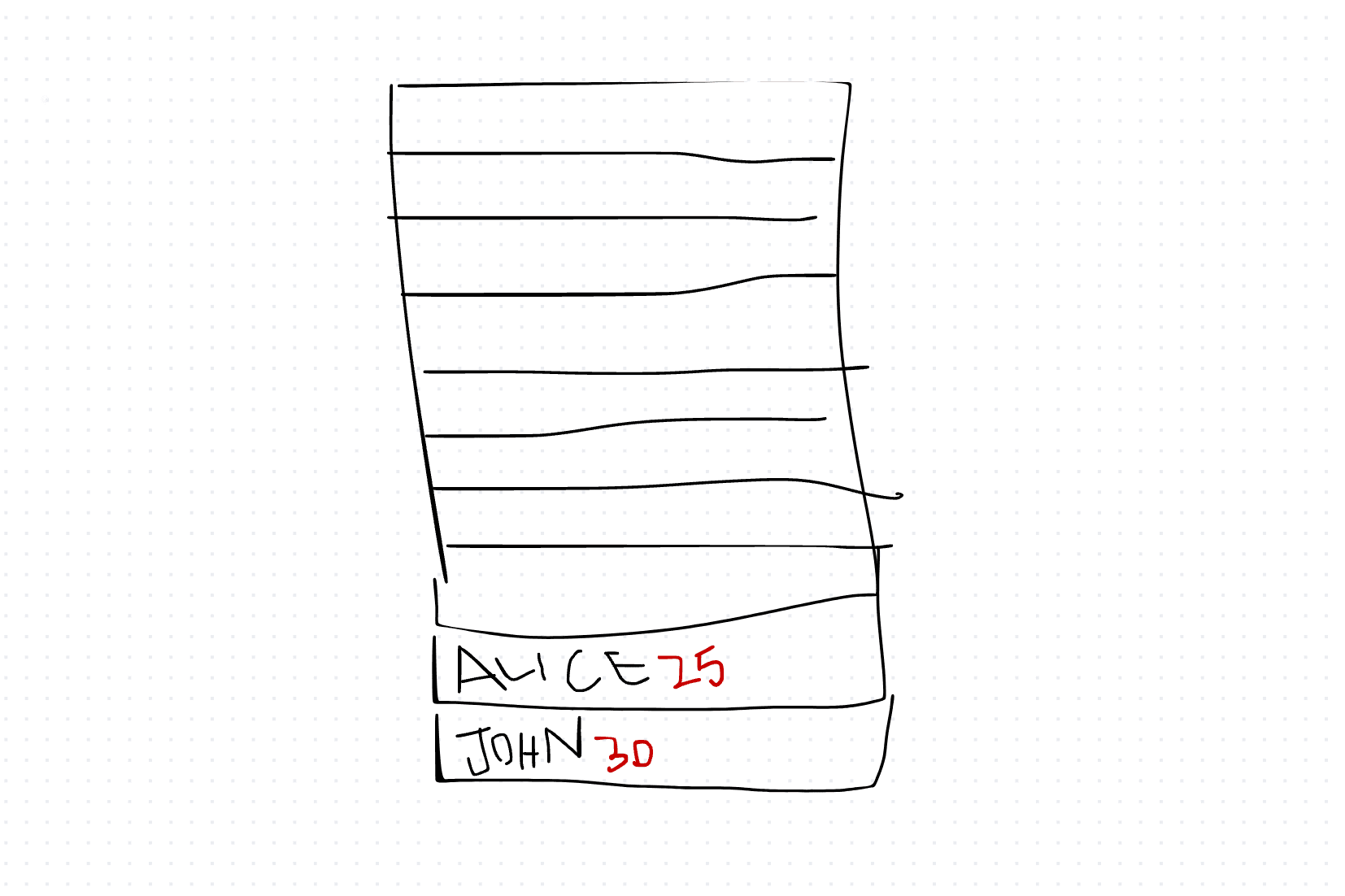
Hashing is a process of converting input data into a fixed-size value, typically a hash code or hash value, using a hash function. Hashing is widely used in various fields such as cryptography, data storage, and data retrieval due to its efficiency and effectiveness.
- Deterministic Mapping: A hash function should produce the same hash value for the same input consistently.
- Uniform Distribution: Ideally, hash values should be uniformly distributed across the hash space to minimize collisions.
- Collision Handling: Collisions occur when two different inputs produce the same hash value. Hashing algorithms employ techniques to handle collisions efficiently, such as chaining or open addressing.
// Simple Hash Function (Modulo)
function simpleHash(key, size) {
let hash = 0;
for (let i = 0; i < key.length; i++) {
hash = (hash + key.charCodeAt(i)) % size;
}
return hash;
}
// Hash Table Implementation
class HashTable {
constructor(size) {
this.size = size;
this.table = Array.from({ length: size }, () => []);
}
insert(key, value) {
const index = simpleHash(key, this.size);
this.table[index].push({ key, value });
}
get(key) {
const index = simpleHash(key, this.size);
const bucket = this.table[index];
for (const item of bucket) {
if (item.key === key) {
return item.value;
}
}
return undefined;
}
}
// Example usage:
const hashTable = new HashTable(10);
hashTable.insert("John", 25);
hashTable.insert("Alice", 30);
console.log("Value for key 'John':", hashTable.get("John"));
console.log("Value for key 'Alice':", hashTable.get("Alice"));