Understanding Stack Data Structure in TypeScript: Implementation and Use Cases
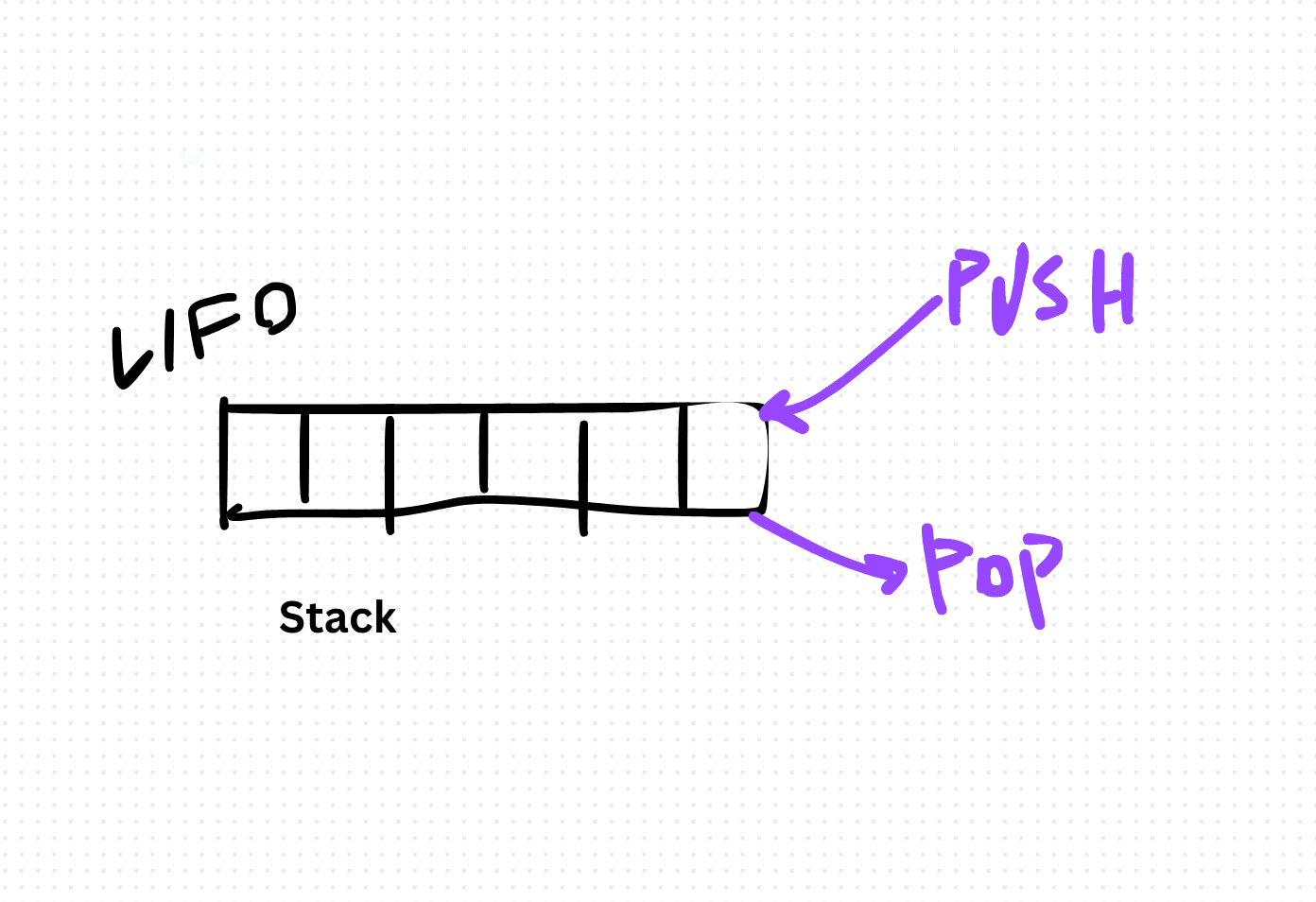
A stack is a linear data structure that stores a collection of elements with two primary operations: push and pop. The push operation adds an element to the top of the stack, while the pop operation removes and returns the top element of the stack. Additionally, stacks often support other operations such as peek (retrieve the top element without removing it) and isEmpty (check if the stack is empty).
class Stack<T> {
private items: T[];
constructor() {
this.items = [];
}
push(item: T): void {
this.items.push(item);
}
pop(): T | undefined {
return this.items.pop();
}
peek(): T | undefined {
return this.items[this.items.length - 1];
}
isEmpty(): boolean {
return this.items.length === 0;
}
size(): number {
return this.items.length;
}
clear(): void {
this.items = [];
}
}
Stacks are a versatile data structure with numerous applications in computer science and software development. By understanding how stacks work and leveraging their simplicity and efficiency, developers can build elegant solutions to a wide range of problems.