Exploring Sets Data Structure in TypeScript: A Comprehensive Guide
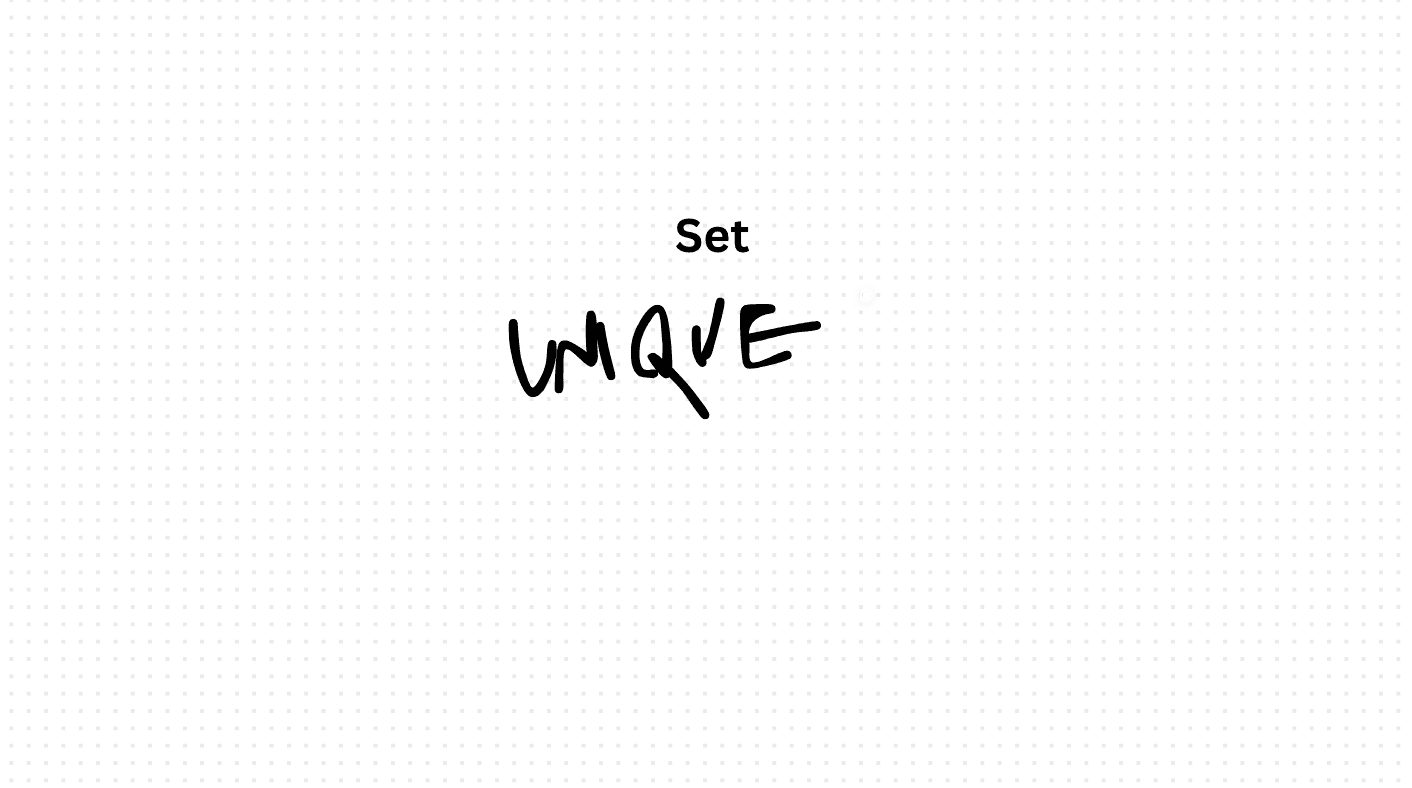
A Set in TypeScript is a collection of unique elements, where each element occurs only once. Unlike arrays, Sets do not maintain any specific order of elements, and they automatically eliminate duplicates.
Key Features of Sets:
- Uniqueness: Sets can only contain unique elements, ensuring that no duplicates are allowed.
- No Order: Elements in a Set are not stored in any specific order, and there is no guarantee of the order in which elements are iterated.
- Efficient Operations: Sets offer efficient methods for adding, removing, and checking the existence of elements, with constant-time complexity for most operations.
const set = new Set<number>();
set.add(1);
set.add(2);
set.add(3);
set.add(1); // IGNORED
set.add(2); // IGNORED
console.log("Size of Set:", set.size); // 3
console.log("Contains 2:", set.has(2)); // TRUE
console.log("Contains 4:", set.has(4)); // FALSE
set.delete(2);
for (let item of set) {
console.log(item);
}
And for objects
const set = new Set<number>();
const person = { id: 1, name:'Gaz'}
set.add(person);
set.add(person); // IGNORED
// however
set.add({ id: 1, name:'Gaz'}); // THIS WORKS! DUPLICATED
Sets data structure in TypeScript provides a convenient way to manage collections of unique elements with efficient operations. Whether you need to remove duplicates from a list of items, perform set operations such as union, intersection, or difference, Sets offer a simple and effective solution. By leveraging Sets in your TypeScript applications, you can streamline data management and improve performance in scenarios where uniqueness is essential.