Exploring Linked List Data Structure in TypeScript
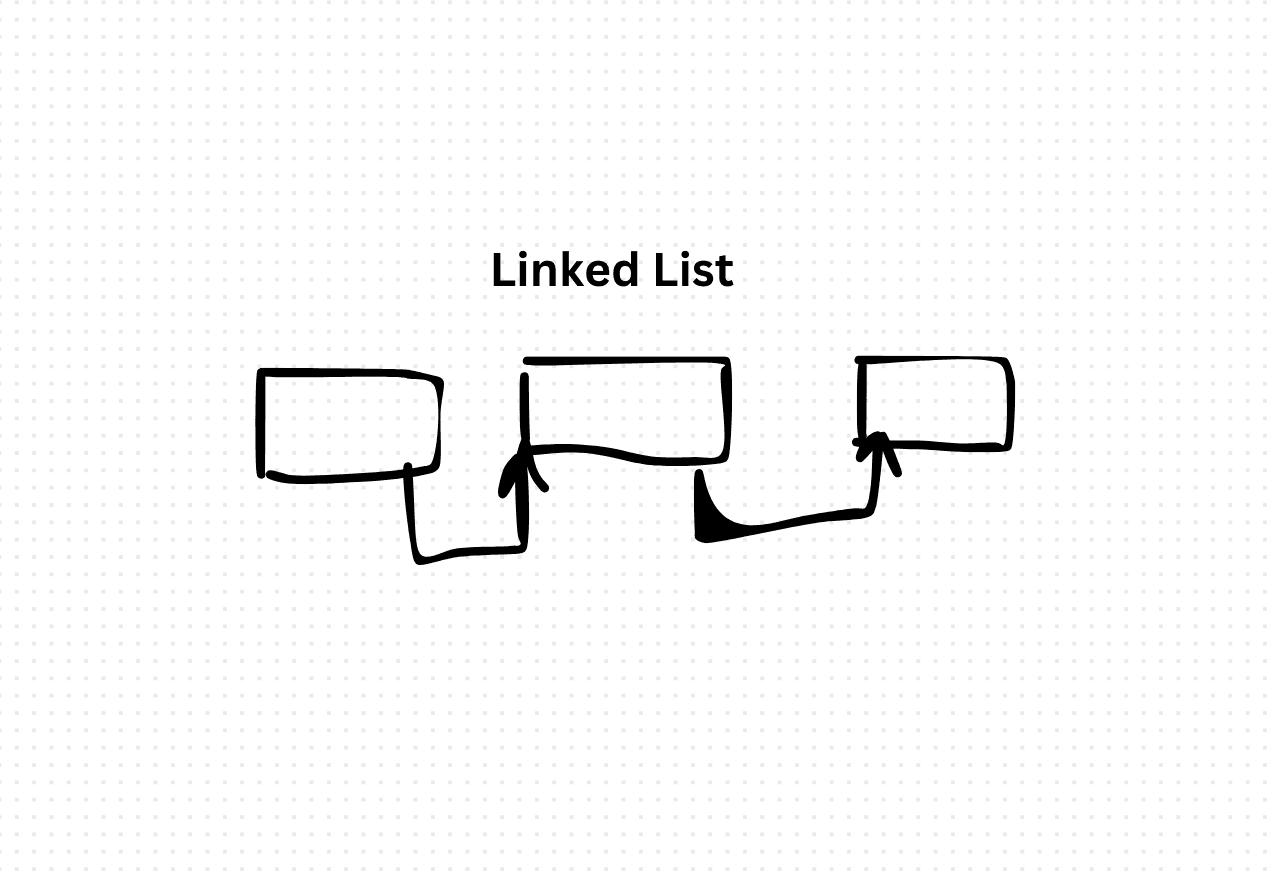
A linked list is a linear data structure consisting of a sequence of elements, known as nodes, where each node contains a data element and a reference (or pointer) to the next node in the sequence. The last node typically points to null, indicating the end of the list. Linked lists come in different variants, such as singly linked lists, doubly linked lists, and circular linked lists, each with its own characteristics and benefits.
class Node<T> {
data: T;
next: Node<T> | null;
constructor(data: T) {
this.data = data;
this.next = null;
}
}
class LinkedList<T> {
head: Node<T> | null;
constructor() {
this.head = null;
}
append(data: T): void {
const newNode = new Node(data);
if (!this.head) {
this.head = newNode;
return;
}
let current = this.head;
while (current.next !== null) {
current = current.next;
}
current.next = newNode;
}
}
Using the LinkedList class, we can perform operations like appending elements to the list, traversing the list, searching for elements, and more.
When to use Linked List?
Linked lists are particularly useful in the following scenarios:
- Dynamic Size Requirements: When the size of the data structure is not predetermined and needs to grow or shrink dynamically, linked lists offer a flexible solution. Unlike arrays, linked lists can easily accommodate changes in size without requiring reallocation of memory.
- Frequent Insertions and Deletions: Linked lists excel at insertion and deletion operations, especially when these operations occur frequently and at arbitrary positions within the list. Unlike arrays, which may require shifting elements to accommodate insertions or deletions, linked lists only require updating pointers, resulting in more efficient operations.
- Memory Efficiency: In scenarios where memory allocation is unpredictable or fragmented, linked lists provide a more memory-efficient option compared to arrays. Each node in a linked list can be allocated dynamically, allowing for optimal use of available memory.
- Implementing Stacks and Queues: Linked lists serve as the underlying data structure for implementing other abstract data types such as stacks and queues. In these cases, linked lists offer efficient push and pop operations for stacks and enqueue and dequeue operations for queues.
- Applications with Iterative Operations: Linked lists are well-suited for scenarios where iterative traversal and manipulation of elements are common. Algorithms such as searching, sorting, and merging can be implemented efficiently using linked lists due to their sequential nature and ease of traversal.
Linked lists are versatile data structures with various applications in computer science and software development. By understanding their principles and implementing them in TypeScript, developers can leverage the advantages of linked lists in building efficient and scalable solutions for diverse problems. Whether it's managing large datasets, implementing algorithms, or optimizing memory usage, linked lists offer a valuable tool in the developer's toolkit.