Exploring Array Data Structure in TypeScript
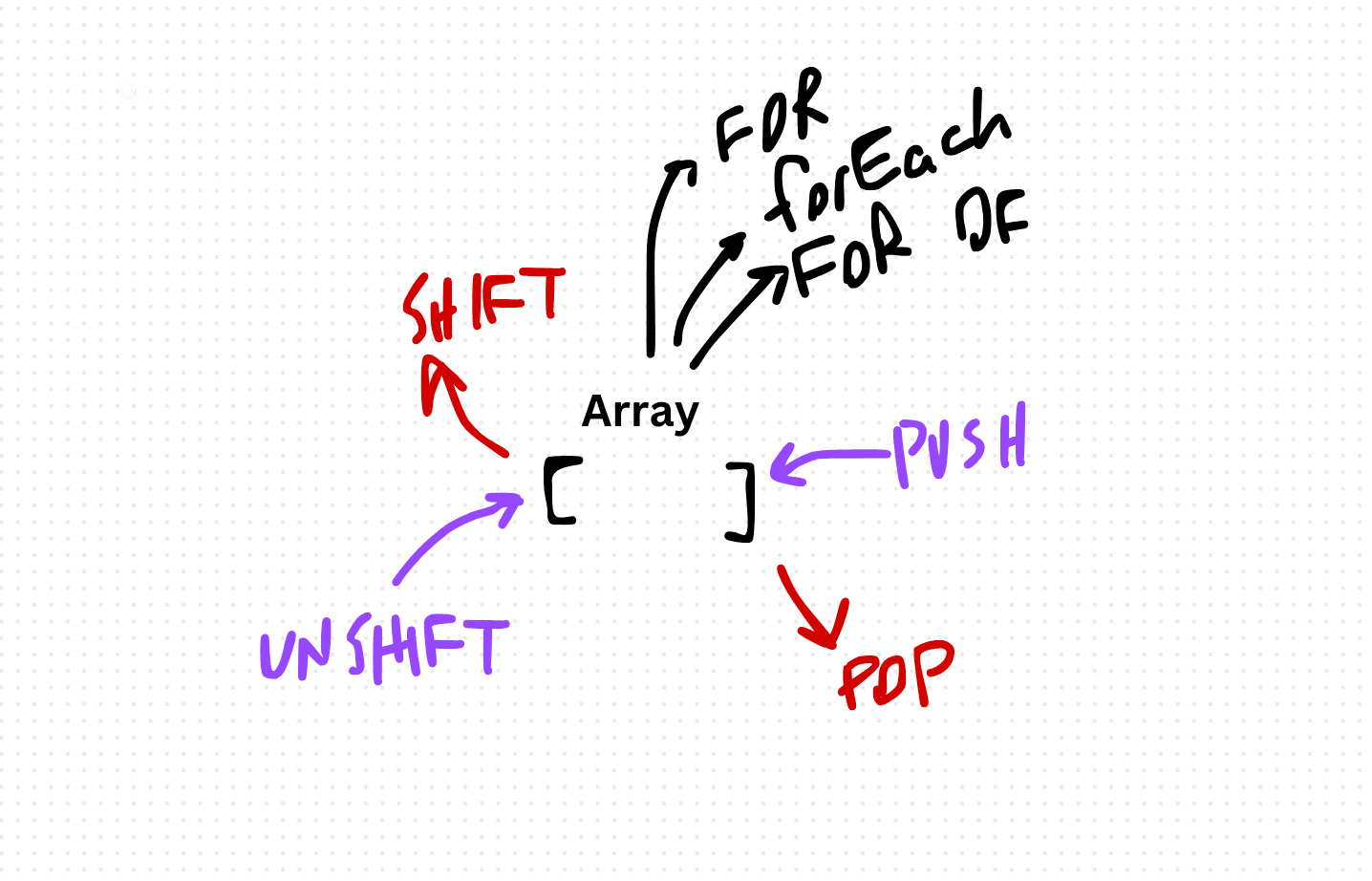
Arrays are one of the most fundamental and versatile data structures in programming. In TypeScript, arrays allow us to store and manipulate collections of elements efficiently. In this article, we'll dive deep into understanding arrays in TypeScript, exploring their features, operations, and best practices through code examples.
In TypeScript, arrays can be created using various methods. Here's how we can initialize arrays:
let numbers: number[] = [1, 2, 3, 4, 5];
let fruits: string[] = ['apple', 'banana', 'orange'];
let colors: Array<string> = new Array('red', 'green', 'blue');
Accessing Elements:
Array elements can be accessed using their index. The index starts from 0 for the first element and goes up to array.length - 1 for the last element.
console.log(numbers[0]); // Output: 1
console.log(fruits[1]);
Iterating Through Arrays:
We can iterate through arrays using loops like for loop, forEach method, or for...of loop.
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
fruits.forEach((fruit) => {
console.log(fruit);
});
for (let color of colors) {
console.log(color);
}
Adding and Removing Elements:
Arrays in TypeScript provide methods like push, pop, shift, and unshift for adding and removing elements.
// Adding elements to the end of the array
numbers.push(6, 7);
// Removing the last element from the array
numbers.pop();
// Adding elements to the beginning of the array
fruits.unshift('mango', 'grapes');
// Removing the first element from the array
fruits.shift();
Slice and Splice:
The slice method returns a shallow copy of a portion of an array, while the splice method changes the contents of an array by removing or replacing existing elements.
let slicedArray = numbers.slice(2, 4); // Returns elements from index 2 to 3
console.log(slicedArray); // Output: [3, 4]
numbers.splice(1, 2, 8, 9); // Removes 2 elements starting from index 1 and adds 8, 9
console.log(numbers); // Output: [1, 8, 9, 5, 6, 7]
Conclusion:
Arrays are essential data structures in TypeScript, offering powerful features for storing and manipulating collections of elements. By understanding array operations and best practices, developers can leverage arrays effectively in their TypeScript projects, facilitating efficient data management and processing.