Avoiding Side Effects in Functions: Best Practices with TypeScript
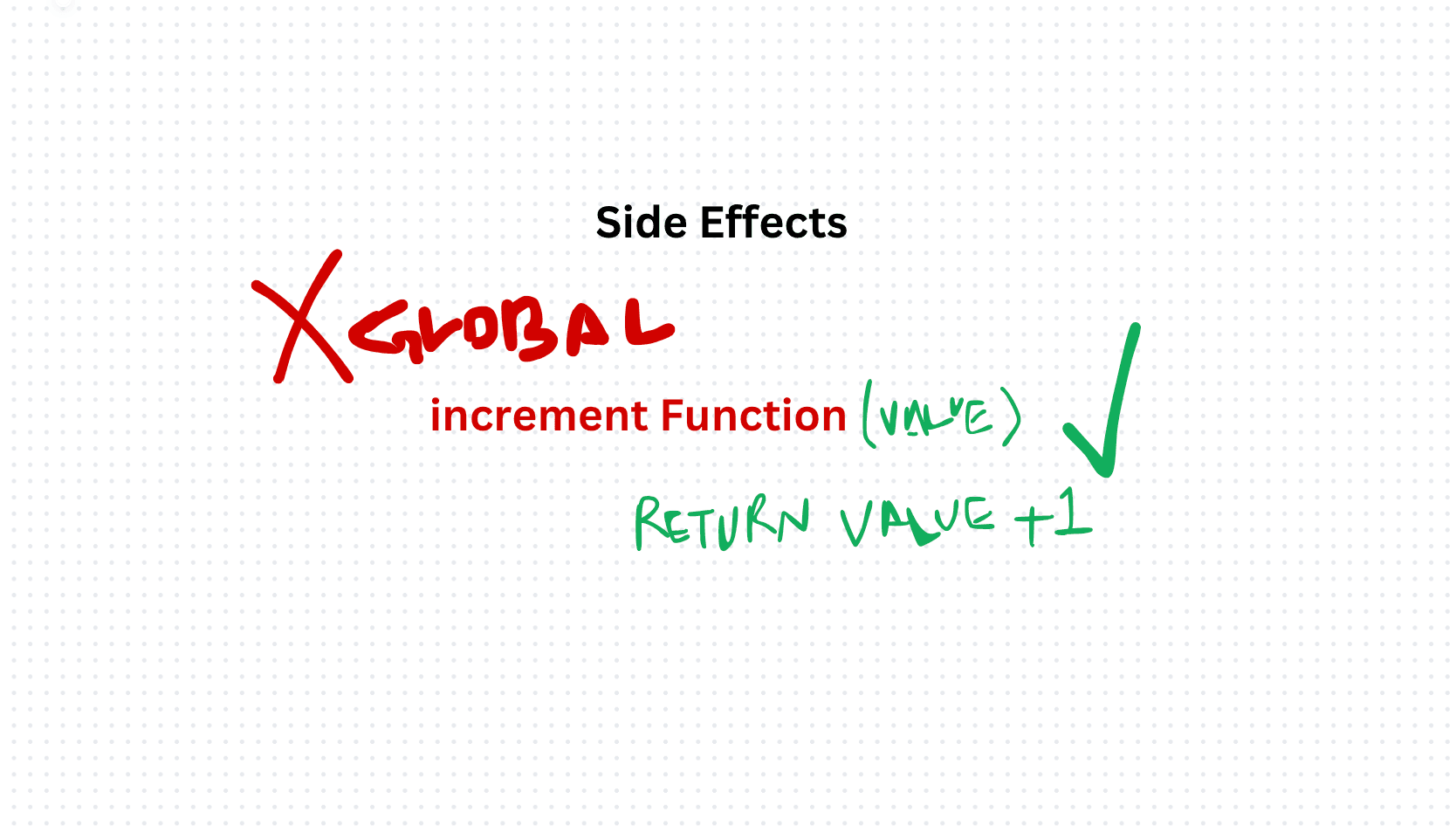
In TypeScript development, writing functions with minimal side effects is crucial for maintaining code reliability, predictability, and ease of debugging. Side effects occur when a function modifies state outside its scope, leading to unexpected behavior and making code harder to understand. In this article, we'll explore the importance of avoiding side effects in functions, discuss best practices for achieving this, and provide TypeScript examples to illustrate these concepts effectively.
Understanding Side Effects:
Side effects in functions can manifest in various forms, including:
- Modifying Global State: Functions that modify global variables, external objects, or mutable data structures introduce side effects.
let globalValue = 0;
function incrementGlobal(value: number): void {
globalValue += value;
}
// Improved Function without Side Effects
function increment(value: number): number {
return value + 1;
}
globalValue += increment(globalValue)
- I/O Operations: Functions that perform I/O operations such as reading from or writing to files, databases, or network requests can have side effects.
Functions that perform I/O operations are prone to side effects because they interact with external resources whose state can change. It's essential for developers to be aware of these potential side effects and design their functions carefully to minimize unintended behavior and maintain the integrity of the application's data and resources.
- Changing Function Inputs: Functions that modify their input parameters directly rather than returning a new value introduce side effects. (Avoiding Overwriting Function Inputs: Best Practices in TypeScript)
Avoiding side effects in functions is essential for writing clean, maintainable, and predictable code in TypeScript. By following best practices such as writing pure functions, embracing immutability, and using functional composition, developers can minimize the risk of unintended side effects and create more robust software systems.