Avoiding Overwriting Function Inputs: Best Practices in TypeScript
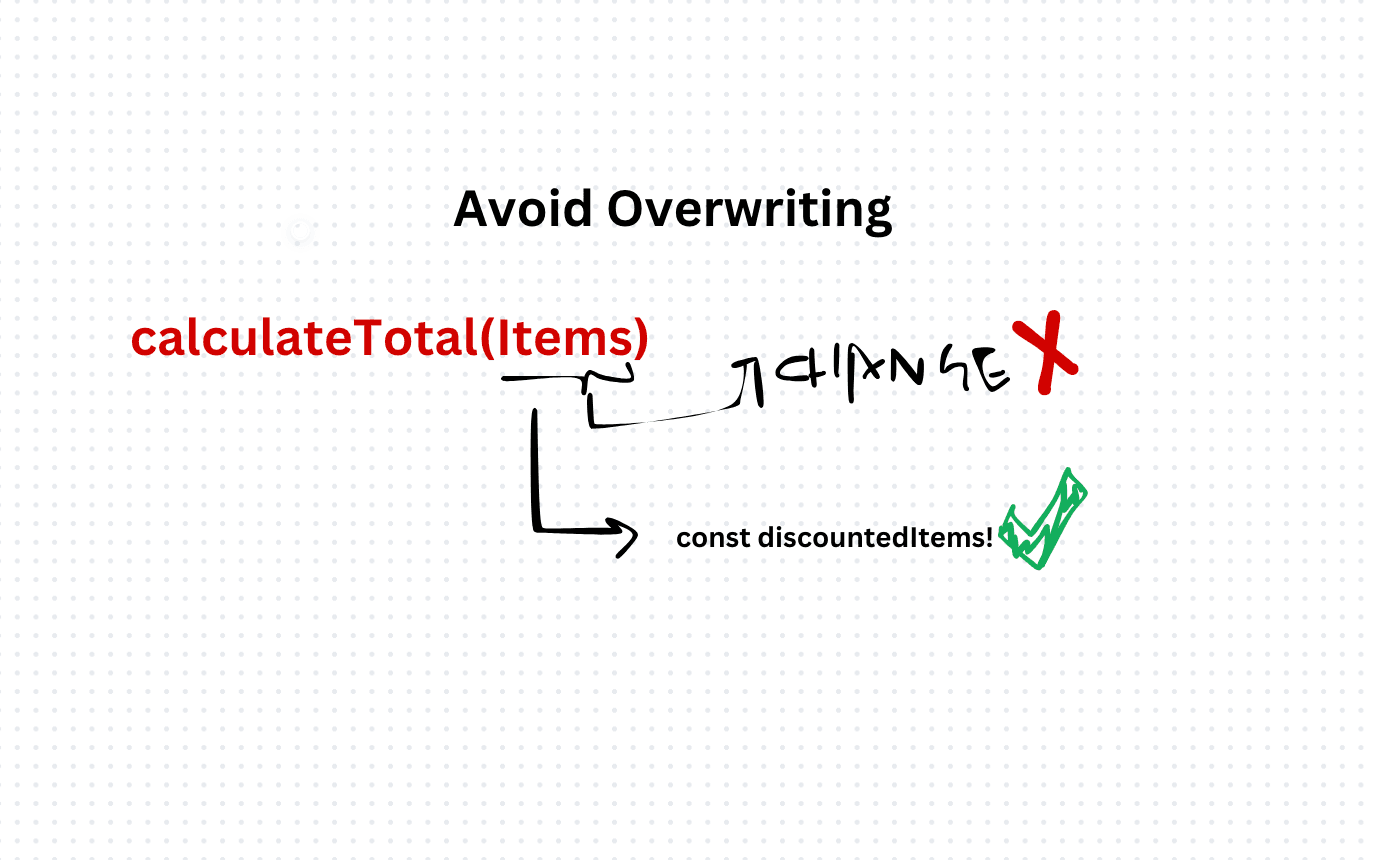
In TypeScript development, it's crucial to adopt practices that promote code stability, maintainability, and prevent unintended side effects. One such practice is avoiding the overwriting of function inputs, which can lead to unexpected behavior and make code harder to reason about. In this article, we'll explore the importance of this practice, its benefits, and provide practical examples in TypeScript to demonstrate how to adhere to it effectively.
Why Avoid Overwriting Function Inputs Matters:
Overwriting function inputs refers to the practice of modifying the values of function parameters within the function body. While it may seem convenient at first, it can lead to several issues:
- Unintended Side Effects: Modifying function inputs directly can introduce unintended side effects, making it difficult to predict the behavior of the function.
- Code Readability: Functions that modify their inputs can be harder to understand, as developers need to track changes made to function parameters throughout the codebase.
- Debugging Complexity: Overwritten function inputs can complicate debugging efforts, as it becomes challenging to trace the origin of a variable's value changes.
function calculateTotal(items: number[], discount: number): number {
for (let i = 0; i < items.length; i++) {
items[i] -= discount; // Overwriting input array elements
}
let total = 0;
for (const item of items) {
total += item;
}
return total;
}
// Improved Function Without Overwriting Inputs
function calculateTotal(items: readonly number[], discount: number): number {
const discountedItems = items.map(item => item - discount);
return discountedItems.reduce((acc, curr) => acc + curr, 0);
}
Avoiding the overwriting of function inputs is a best practice in TypeScript development that promotes code stability, readability, and maintainability. By treating function parameters as immutable values and adhering to functional programming principles, developers can create cleaner, more predictable code that is easier to understand, debug, and maintain.