Modifiers, Public, Protected, Private in Typescript
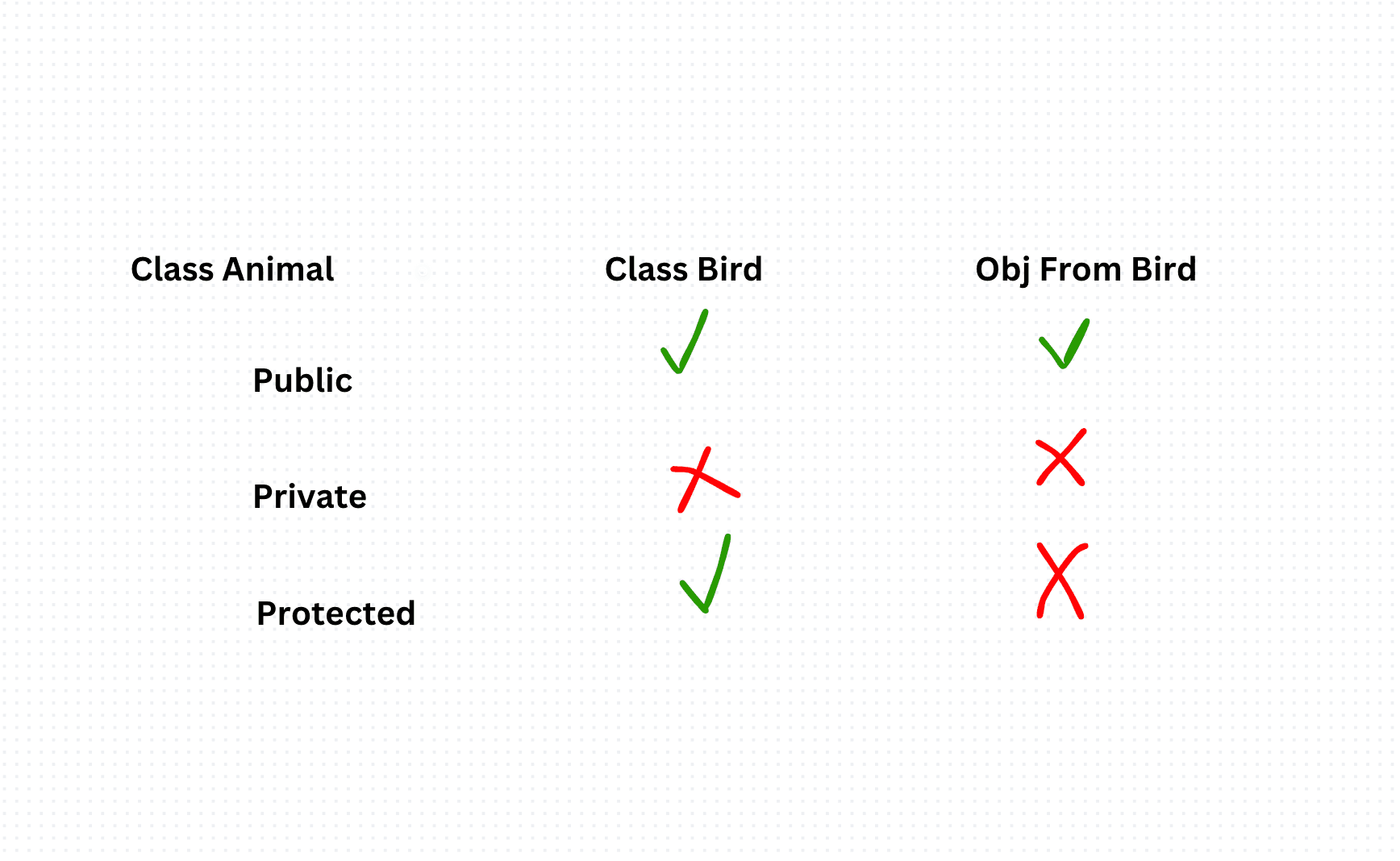
Access modifiers in TypeScript allow you to control the visibility of class members (properties and methods) from outside the class. There are three main access modifiers in TypeScript:
- Public: Public members are accessible from anywhere, both within the class and from external code. By default, class members in TypeScript are public if no access modifier is specified.
- Protected: Protected members are accessible within the class that defines them and within subclasses (inheritance), but not from external code.
- Private: Private members are accessible only within the class that defines them. They are not accessible from subclasses or external code.
class Person {
public name: string;
protected age: number;
private email: string;
constructor(name: string, age: number, email: string) {
this.name = name;
this.age = age;
this.email = email;
}
greet() {
console.log(`Hello, my name is ${this.name}.`);
}
}
So if you use this.age, is allowed and this.email is not
class Employee extends Person {
constructor(name: string, age: number, email: string) {
super(name, age, email);
}
showDetails() {
console.log(`Name: ${this.name}, Age: ${this.age}`); // Allowed
console.log(`Email: ${this.email}`); // Error: Property 'email' is private and only accessible within class 'Person'.
}
}
Also if you make an object
const person = new Person('Alice', 30, 'alice@example.com');
console.log(person.name); // Allowed
console.log(person.age); // Error: Property 'age' is protected
console.log(person.email); // Error: Property 'email' is protected
Understanding access modifiers in TypeScript classes is essential for writing well-structured and maintainable code. By choosing the appropriate access modifier for each class member, you can ensure proper encapsulation, data hiding, and inheritance in your TypeScript applications. Whether you're building small-scale projects or large-scale applications, mastering access modifiers will help you design cleaner and more robust codebases. Happy coding! 🚀