TypeScript for Large-Scale Applications
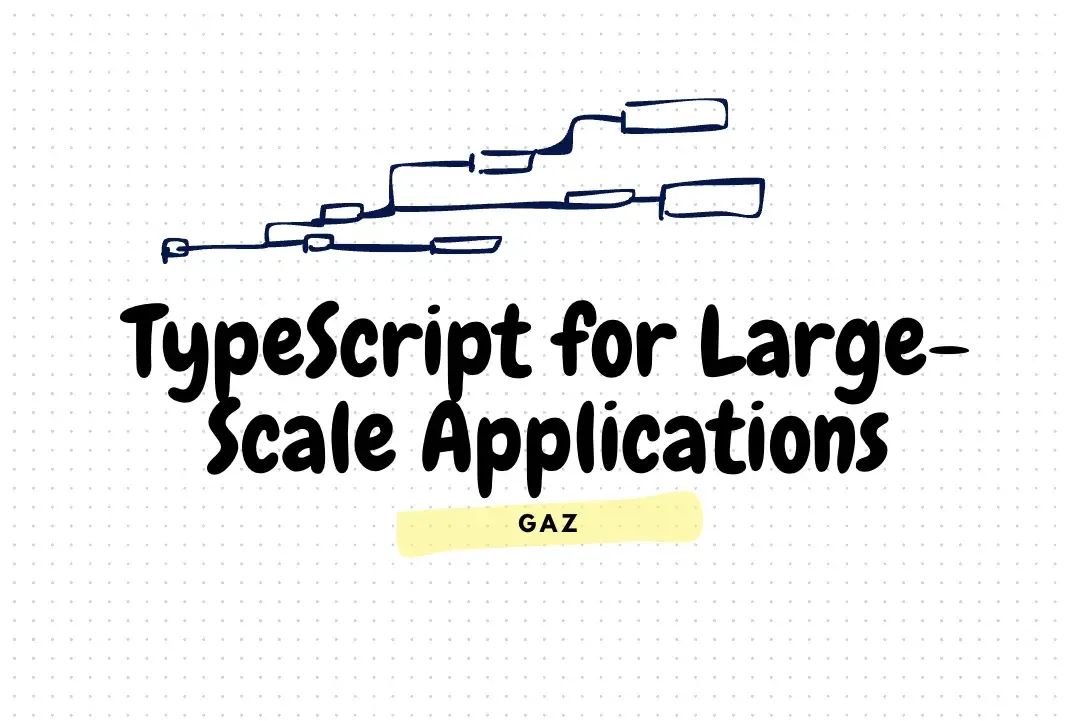
Developing and maintaining large-scale applications is a challenging endeavor. Over my years as a software developer, I’ve faced issues ranging from unwieldy codebases to elusive bugs. Enter TypeScript—a tool that transformed the way I approach these projects.
Why TypeScript?
JavaScript is an incredibly flexible language, which is both its strength and weakness. Flexibility often leads to unforeseen errors, especially in large applications with multiple developers. TypeScript adds a type system on top of JavaScript, enabling developers to:
- Catch errors at compile time rather than runtime.
- Improve code readability and maintainability.
- Facilitate better collaboration in teams by providing clear contracts.
1. Refactoring Without Fear
When working on a codebase with thousands of lines, refactoring can feel like walking through a minefield. TypeScript's static type-checking ensures that renaming a function, changing an interface, or restructuring modules doesn’t introduce bugs.
interface User {
id: number;
name: string;
email: string;
}
function getUserName(user: User): string {
return user.name;
}
// Refactor user to include a new property
interface User {
id: number;
name: string;
email: string;
isAdmin: boolean;
}
// TypeScript will notify us of any changes needed.
2. Managing Dependencies Between Modules
As projects grow, the interdependencies between modules increase. TypeScript’s module system and type declarations make these relationships explicit and manageable.
// auth.ts
export interface AuthToken {
token: string;
expiry: Date;
}
export function validateToken(token: AuthToken): boolean {
return token.expiry > new Date();
}
// app.ts
import { AuthToken, validateToken } from './auth';
const token: AuthToken = { token: 'abc123', expiry: new Date(Date.now() + 60000) };
console.log(validateToken(token));
3. Avoiding Common Bugs
TypeScript’s strict null checks and type inference help prevent common issues like undefined or null errors.
function greetUser(user: { name: string } | null): string {
if (!user) {
throw new Error('User not found');
}
return `Hello, ${user.name}!`;
}
const user = null;
console.log(greetUser(user)); // Compile-time error if strict null checks are enabled
4. Improved Onboarding for New Developers
In a large-scale project, onboarding new developers can be daunting. TypeScript’s type annotations and IntelliSense support provide a form of built-in documentation.
/**
* Calculates the area of a rectangle.
* @param width Width of the rectangle
* @param height Height of the rectangle
* @returns Area of the rectangle
*/
function calculateArea(width: number, height: number): number {
return width * height;
}
// IDEs show detailed information about the function usage.
Lessons Learned from Real-World Use
- Gradual Adoption: Migrating to TypeScript doesn’t have to be an all-or-nothing decision. Start with key modules and incrementally adopt it.
- Strict Configuration: Using strict mode (strict: true in tsconfig.json) helps enforce best practices.
- Leveraging Third-Party Type Definitions: Many popular JavaScript libraries provide TypeScript definitions, making integration seamless.
Conclusion
TypeScript has become an indispensable tool in my workflow for building and maintaining large-scale applications. Its ability to catch errors early, enhance developer productivity, and ensure code quality makes it a must-have for any serious project.
If you’re hesitant about adopting TypeScript, I’d recommend starting with a small project or module. The benefits become apparent quickly and can make a world of difference as your codebase grows.