Type Inference and Type Compatibility in TypeScript
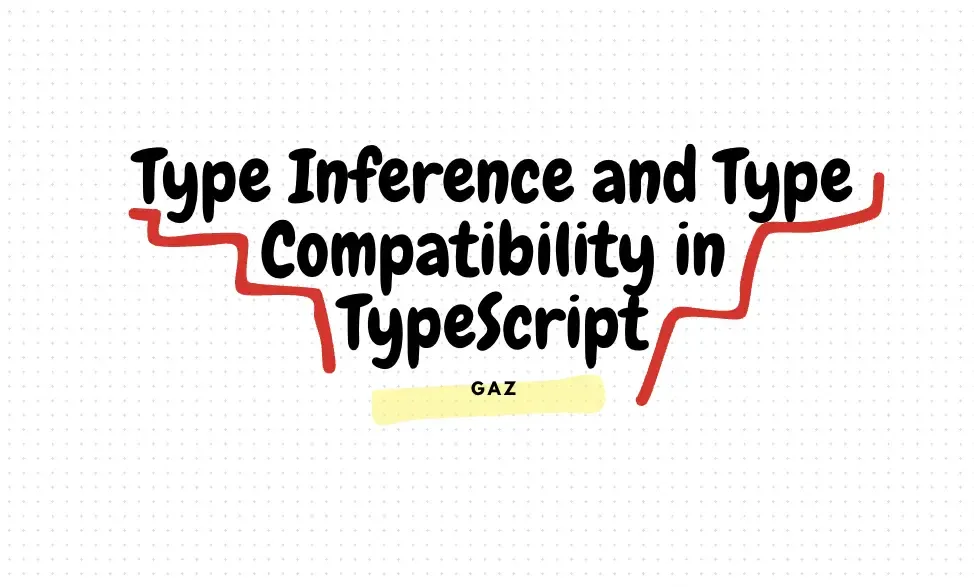
TypeScript, a superset of JavaScript, provides powerful type-checking and inference capabilities that enhance code safety and developer productivity. In this article, I will share my experience with TypeScript's type inference and type compatibility, showcasing how these features simplify development while ensuring type safety.
Type Inference
Type inference is the mechanism by which TypeScript automatically determines the type of a variable, parameter, or return value without explicit type annotations. This feature can save developers time and make the code more concise while maintaining strong typing.
Example 1: Variable Type Inference
let message = "Hello, TypeScript!"; // inferred as string
message = 42; // Error: Type 'number' is not assignable to type 'string'.
In the example above, TypeScript infers the type of message as string based on the initial assignment. Any subsequent assignment with a type mismatch will result in a compile-time error.
Example 2: Function Return Type Inference
function add(a: number, b: number) {
return a + b; // inferred as number
}
const result = add(5, 10); // result is inferred as number
Here, TypeScript infers the return type of the add function as number based on the types of the arguments and the operation.
Type Compatibility
TypeScript’s type compatibility system is structural, meaning it compares types based on their shape rather than their explicit declarations. This allows for greater flexibility while adhering to type safety.
Example 1: Structural Typing
TypeScript’s type compatibility system is structural, meaning it compares types based on their shape rather than their explicit declarations. This allows for greater flexibility while adhering to type safety.
type Point = {
x: number;
y: number;
};
type NamedPoint = {
x: number;
y: number;
name: string;
};
const point: Point = { x: 10, y: 20 };
const namedPoint: NamedPoint = { x: 15, y: 25, name: "Origin" };
// Structural compatibility: NamedPoint has all properties of Point
const anotherPoint: Point = namedPoint;
In this case, NamedPoint is compatible with Point because it has all the required properties of Point, plus additional ones. This is the essence of structural typing.
Example 2: Function Compatibility
type Log = (message: string) => void;
function logToConsole(msg: string) {
console.log(msg);
}
let logger: Log = logToConsole; // Compatible: Shapes match
logger("Hello, Logger!");
Here, the function logToConsole is compatible with the Log type because it has the same parameter list and return type.
Combining Inference and Compatibility
When type inference and compatibility come together, they create a seamless developer experience. For instance:
const points: Point[] = [
{ x: 1, y: 2 },
{ x: 3, y: 4 },
]; // Array elements inferred as Point
function printPoints(points: Point[]) {
points.forEach((point) => console.log(`(${point.x}, ${point.y})`));
}
printPoints(points); // Compatible as the inferred type matches the parameter type
Here, TypeScript infers the type of the points array as Point[], and its compatibility with the printPoints function ensures smooth integration without explicit annotations.
Best Practices
- Leverage Inference: Avoid unnecessary type annotations when TypeScript can infer types accurately.
- Validate Compatibility: Understand and utilize structural typing for flexible yet safe type relationships.
- Combine Features: Use inference and compatibility together to write clean and robust code.
Conclusion
Type inference and type compatibility are cornerstone features of TypeScript that simplify development while ensuring type safety. By leveraging these features, developers can write more concise and maintainable code without sacrificing robustness. As my experience with TypeScript has shown, mastering these concepts can significantly enhance productivity and reduce errors in large-scale projects.