Template Literal Types in TypeScript
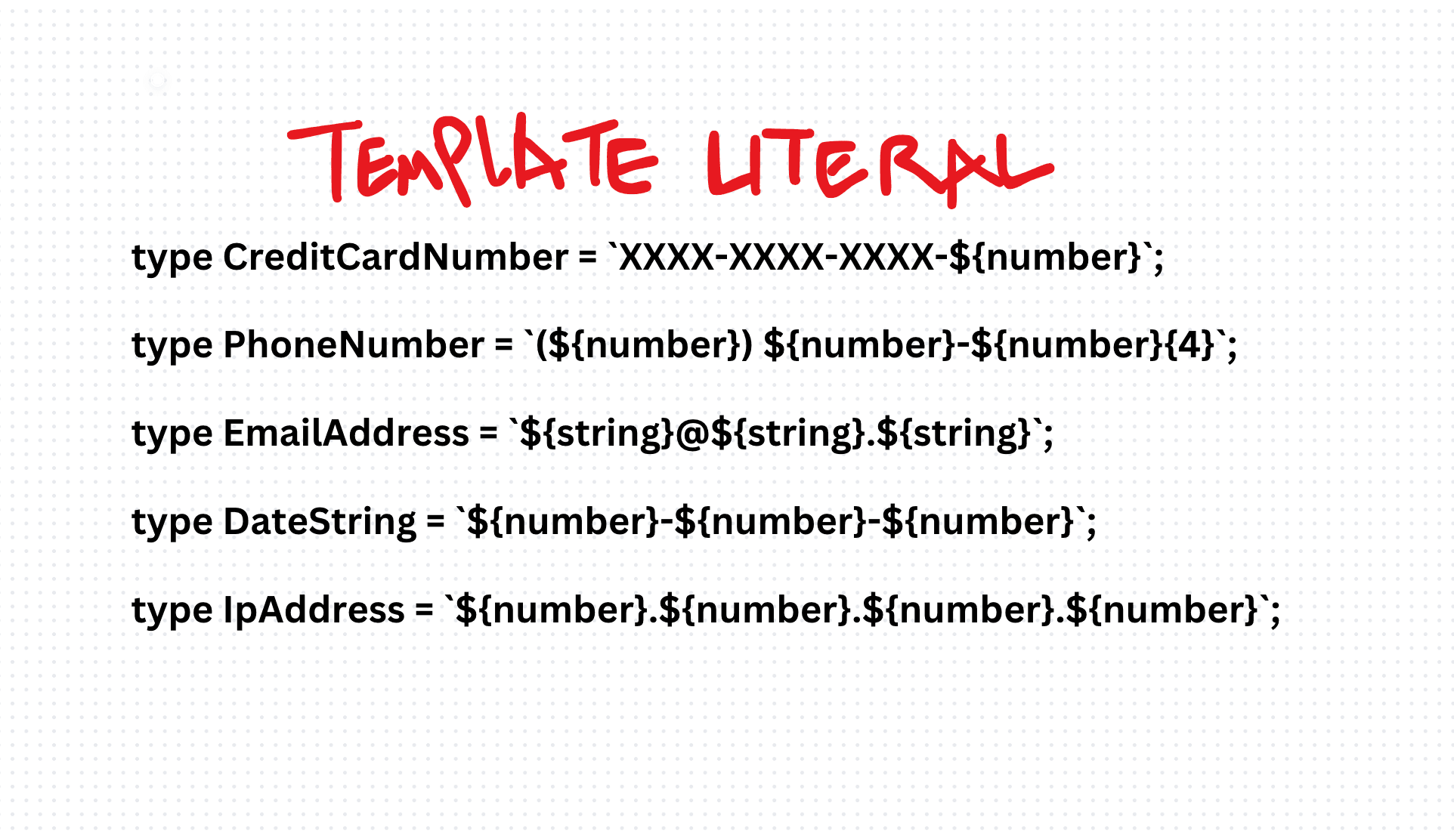
Template literal types are a feature in TypeScript that allows you to create a type that represents a string literal. This type can be used to enforce the structure of a string at compile-time. This means that you can define a type that ensures a string conforms to a specific format, such as a date or a URL.
Let's say we want to create a type that represents a URL. We can define a template literal type as follows:
type URL = `${string}://${string}`;
In this example, we're creating a type called URL that represents a string in the format scheme://host. The ${string}://${string} syntax is called a template literal type. It's a special syntax in TypeScript that allows us to create a type that represents a string literal.
More examples:
type CreditCardNumber = `XXXX-XXXX-XXXX-${number}`;
type PhoneNumber = `(${number}) ${number}-${number}{4}`;
type EmailAddress = `${string}@${string}.${string}`;
type DateString = `${number}-${number}-${number}`;
type IpAddress = `${number}.${number}.${number}.${number}`;
type Color = "red" | "green" | "blue";
type HexColor<T extends Color> = `#${string}`;
let myColor: HexColor<"blue"> = "#0000FF";
Template literal types are a powerful feature in TypeScript that can help you improve the type safety of your code. By defining a template literal type, you can enforce the structure of a string and catch errors early on in the development process. In this article, we've seen how to create a template literal type and use it to enforce the structure of a URL. With template literal types, you can write more maintainable, readable, and error-free code.