What Developers Are Saying About React 19: Pros and Cons
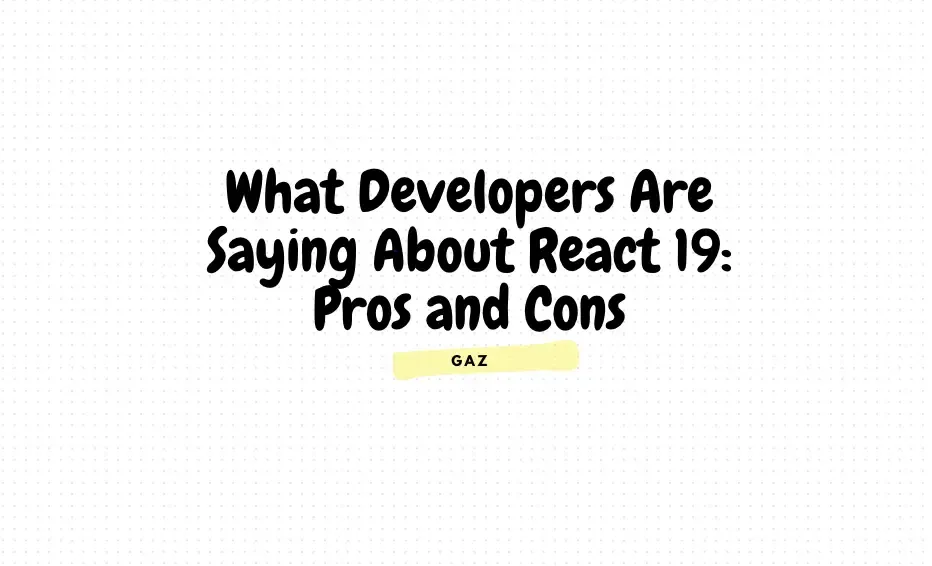
React 19 has been the talk of the development community since its release. With new features and optimizations, it promises to enhance the developer experience and application performance. Drawing from my experience and conversations with other developers, this article explores the pros and cons of React 19, complete with TypeScript examples.
What’s New in React 19?
Key highlights of React 19 include:
- Concurrent Rendering Enhancements: Improved support for rendering large components without blocking the main thread.
- Server Components: Seamless integration of server-rendered components with client-side interactivity.
- Enhanced Strict Mode: Provides better insights during development.
- Automatic Batching Updates: Minimizes re-renders, improving performance out of the box.
While these updates have been generally well-received, they also introduce complexities worth considering.
Pros of React 19
1. Improved Performance
React 19’s concurrent rendering makes it easier to handle complex UIs:
import React, { useState, Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
export default function App() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Concurrent Rendering Example</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent count={count} />
</Suspense>
</div>
);
}
2. Enhanced Developer Tools
React’s upgraded Strict Mode now catches more potential bugs, making debugging easier during development.
3. Better Resource Management
Server Components allow the backend to handle heavy lifting, reducing the client-side workload. This is especially useful for data-intensive applications:
Example: Using Server Components
// server.js
import { renderToPipeableStream } from 'react-dom/server';
import App from './App';
export default function handler(req, res) {
const { pipe } = renderToPipeableStream(<App />, {
onCompleteShell() {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/html');
pipe(res);
},
});
}
Cons of React 19
1. Steeper Learning Curve
Features like Server Components and concurrent rendering require a deeper understanding of React’s internals. Developers transitioning from earlier versions may face challenges.
2. Compatibility Issues
Certain libraries may not yet support React 19’s new features, necessitating workarounds or delays in adoption.
3. Potential Overhead
While automatic batching reduces re-renders, it can introduce unexpected behavior if not handled carefully.
Example: Automatic Batching Pitfall
import React, { useState } from 'react';
export default function Counter() {
const [count, setCount] = useState(0);
const [flag, setFlag] = useState(false);
function handleClick() {
setCount((prev) => prev + 1);
setFlag((prev) => !prev);
// Both updates are batched, but ensure side effects align with expectations
}
return (
<div>
<p>Count: {count}</p>
<button onClick={handleClick}>Update</button>
</div>
);
}
Should You Upgrade to React 19?
Consider upgrading if:
- Your project demands improved performance and scalability.
- You’re starting a new project and can take full advantage of the new features.
- However, for existing projects, evaluate compatibility and training needs before transitioning.
Conclusion
React 19 represents a significant step forward in front-end development. While its benefits are compelling, adopting it requires careful consideration and planning. By understanding its pros and cons, developers can make informed decisions about leveraging React 19 effectively.
I hope this analysis helps you navigate the landscape of React 19. Share your thoughts or questions below!