Mastering Partial, Required, and Record in TypeScript: A Guide to Type Safety
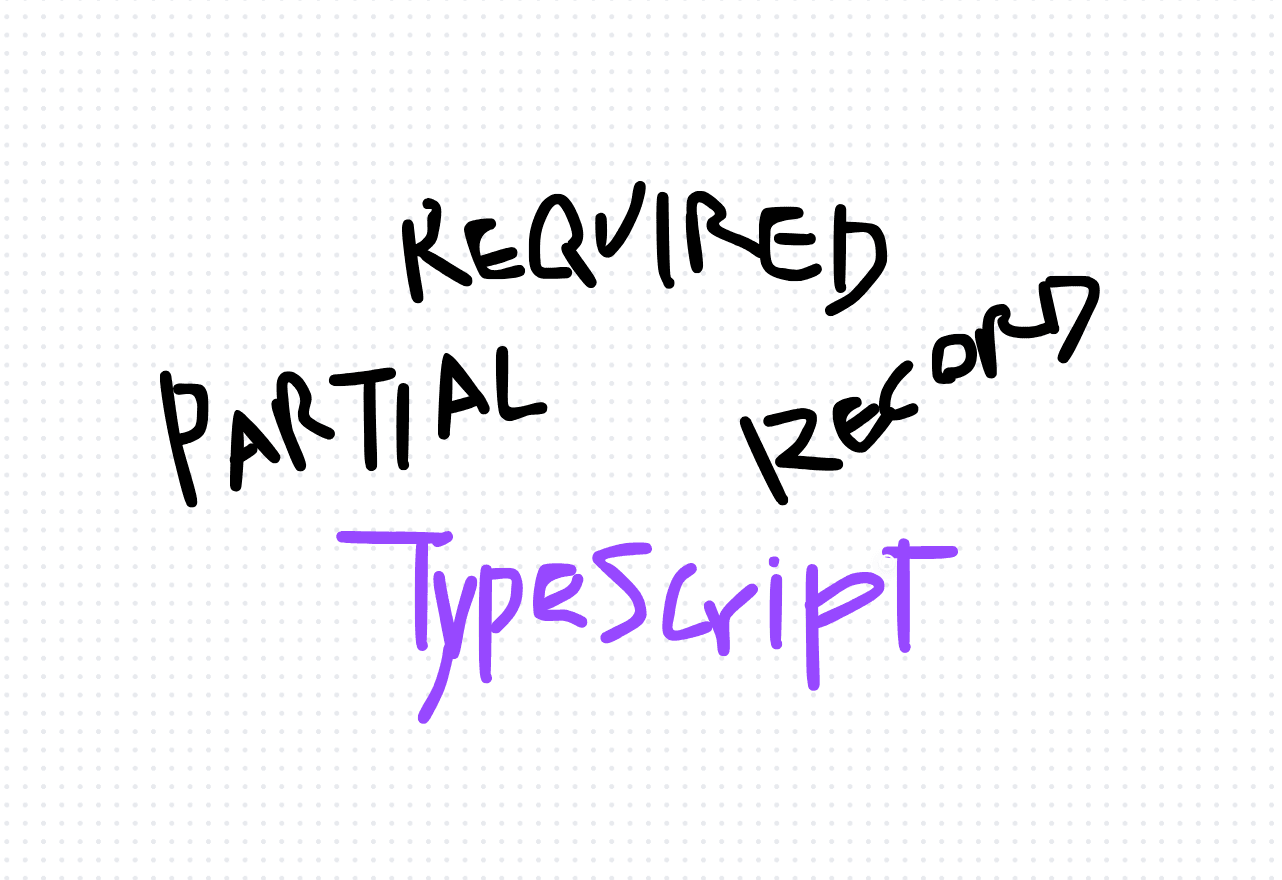
Partial is a type that represents a type that allows all properties to be optional. Required is a type that represents a type that requires all properties to be present. Record is a type that represents a type that is a mapping from string to any.
interface User {
name: string;
age: number;
email: string;
}
We can use Partial to create a type that represents a partially typed object:
type PartialUser = Partial<User>;
The PartialUser type will be a type that represents an object that has the same properties as the User interface, but all of which are optional.
type RequiredUser = Required<PartialUser>;
The RequiredUser type will be a type that represents an object that has the same properties as the PartialUser type, but all of which are required.
type NumberRecord = Record<string, number>;
const numberRecord: NumberRecord = {
'one': 1,
'two': 2,
'three': 3,
};
Partial, Required, and Record are powerful types in TypeScript that can be used to create powerful type guards that enforce the structure of an object. By using these types, you can improve the type safety, maintainability, and reusability of your code. In this article, we've seen how to use Partial, Required, and Record to create type guards that enforce the structure of an object. With these types, you can write more robust and maintainable code.