Decorators in TypeScript: Code Reusability
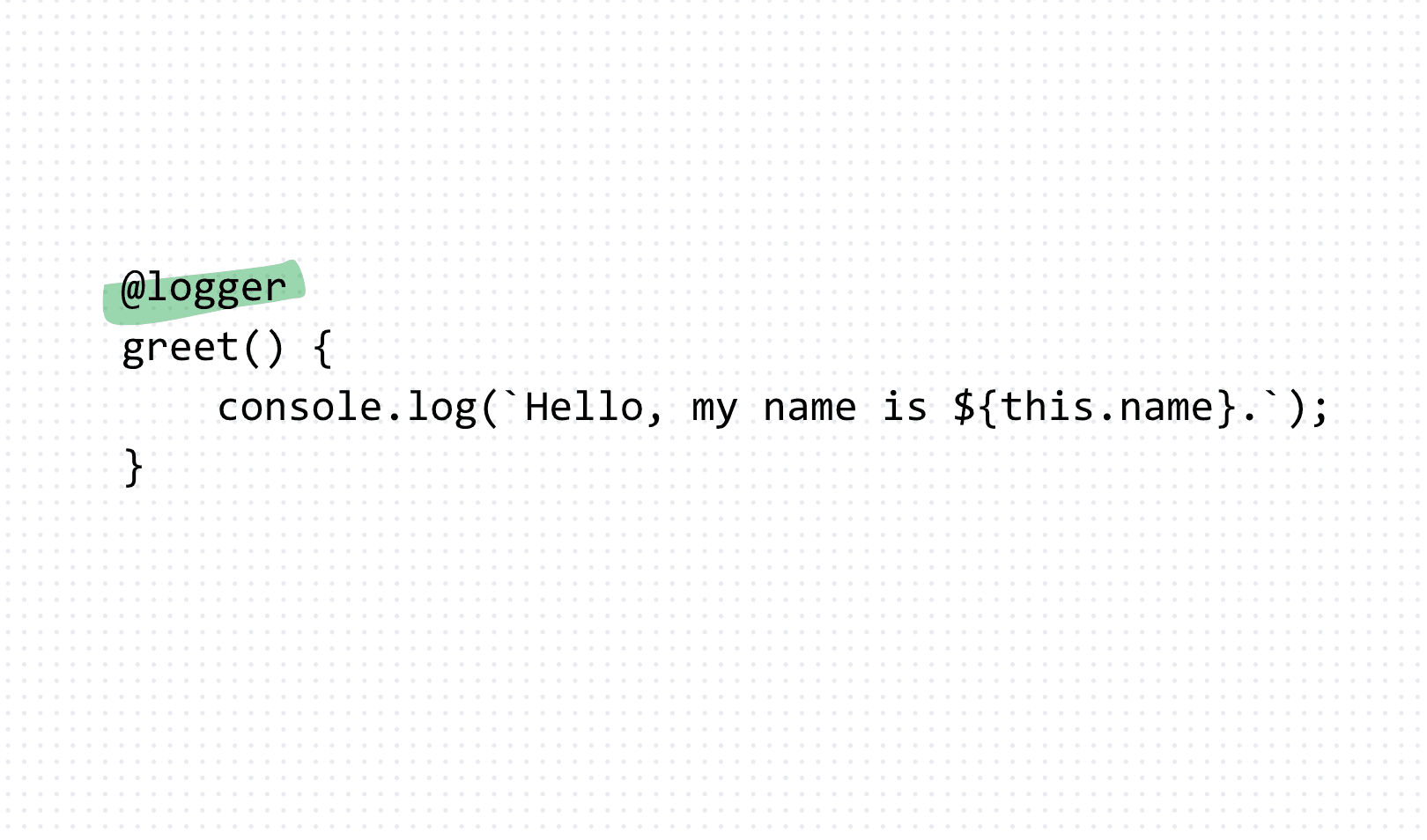
Decorators are a special kind of declaration that can be attached to a class declaration, method, accessor, property, or parameter. They provide a way to modify or extend the behavior of a class or method. Decorators are often used to add metadata to a class or method, such as logging or caching.
class User {
constructor(private name: string, private age: number) {}
greet() {
console.log('start: greet')
console.log(`Hello, my name is ${this.name}.`);
console.log('end: greet')
}
printAge() {
console.log('start: printAge')
console.log(`I am ${this.age} years old`);
console.log('end: printAge')
}
}
const user = new User("Ron", 25);
user.greet();
user.printAge();
So we could write a logger funciton like this:
function logger(originalMethod: any, _context: any) {
function replacementMethod(this: any, ...args: any[]) {
console.log("start:", originalMethod.name);
const result = originalMethod.call(this, ...args);
console.log("end:", originalMethod.name);
return result;
}
return replacementMethod;
}
Then convert our class to be like:
class User {
constructor(private name: string, private age: number) {}
@logger
greet() {
console.log(`Hello, my name is ${this.name}.`);
}
@logger
printAge() {
console.log(`I am ${this.age} years old`);
}
}
const user = new User("Ron", 25);
user.greet();
user.printAge();
Decorators in TypeScript provide a powerful mechanism for metaprogramming, allowing developers to add metadata and modify the behavior of classes, methods, and properties. By leveraging decorators, you can enhance the readability, maintainability, and functionality of your codebase. Understanding decorators and incorporating them into your projects can significantly improve your development workflow and the quality of your applications.