Node.js Scalability: Implementing a Node.js Cluster
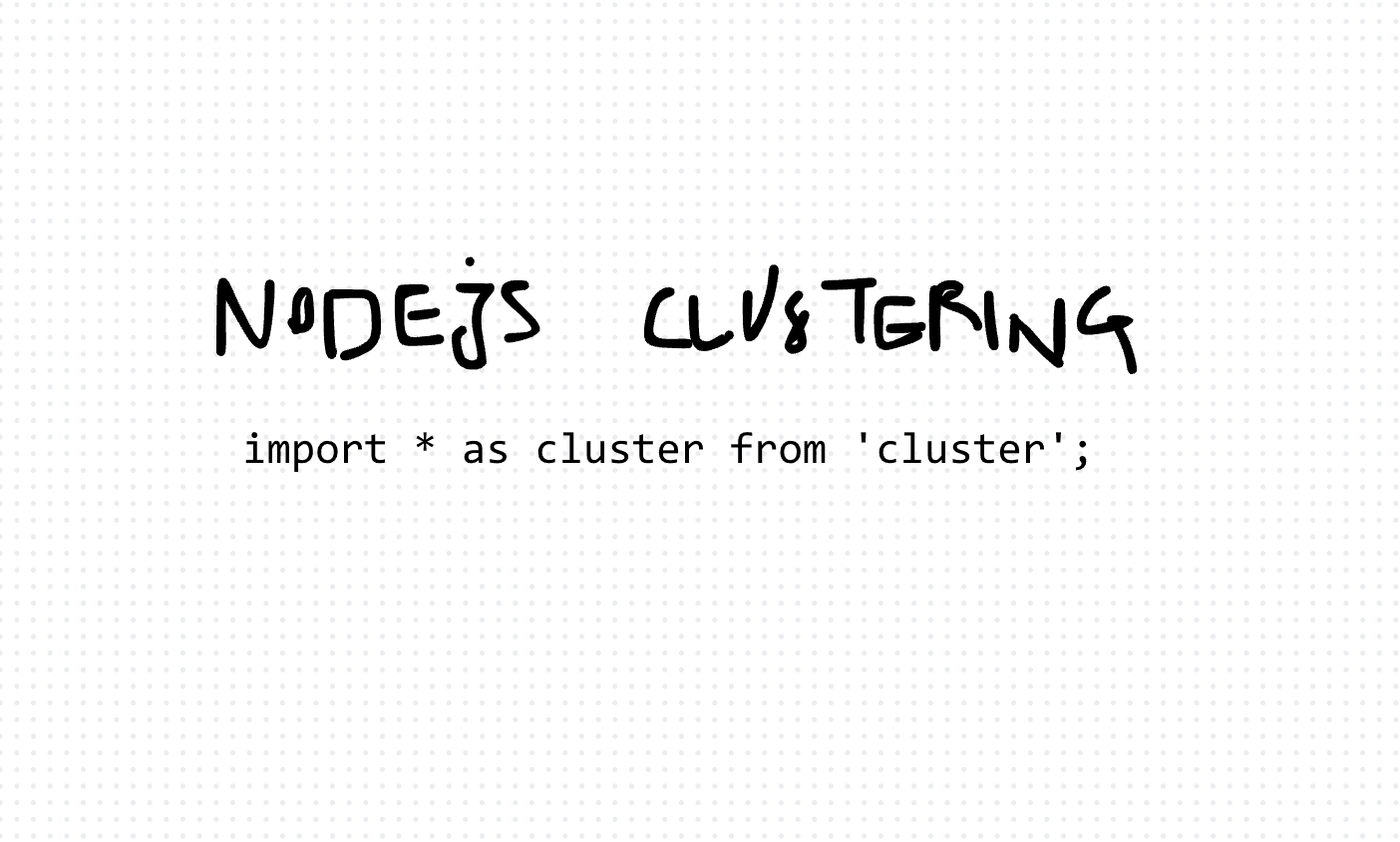
Node.js clusters are a built-in module that enables the creation of multiple instances of a Node.js process, known as worker processes, to handle incoming requests concurrently. By distributing the workload across multiple CPU cores, clusters leverage the full potential of modern multi-core systems, improving throughput and responsiveness.
Let's dive into a TypeScript example demonstrating how to implement a Node.js cluster in your application:
import * as cluster from 'cluster';
import * as os from 'os';
// Check if the current process is the master process
if (cluster.isMaster) {
// Retrieve the number of CPU cores
const numCores = os.cpus().length;
// Fork worker processes equal to the number of CPU cores
for (let i = 0; i < numCores; i++) {
cluster.fork();
}
// Listen for worker exit events
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} exited with code ${code} and signal ${signal}`);
// Restart the worker process
cluster.fork();
});
} else {
// Worker process code
console.log(`Worker ${process.pid} started`);
// Place your application logic here
// For example, start an HTTP server
// ...
}
In the above example, the master process determines the number of CPU cores available and forks worker processes accordingly. Each worker process operates independently, executing the application logic in parallel.
Node.js clusters offer a powerful mechanism for achieving parallelism and scalability in Node.js applications. By distributing the workload across multiple worker processes, clusters enable efficient utilization of CPU resources and enhance application performance and resilience. With the implementation examples and best practices outlined in this article, you're equipped to harness the full potential of Node.js clusters and take your applications to the next level of scalability.