Implementing Salt with Encryption in TypeScript
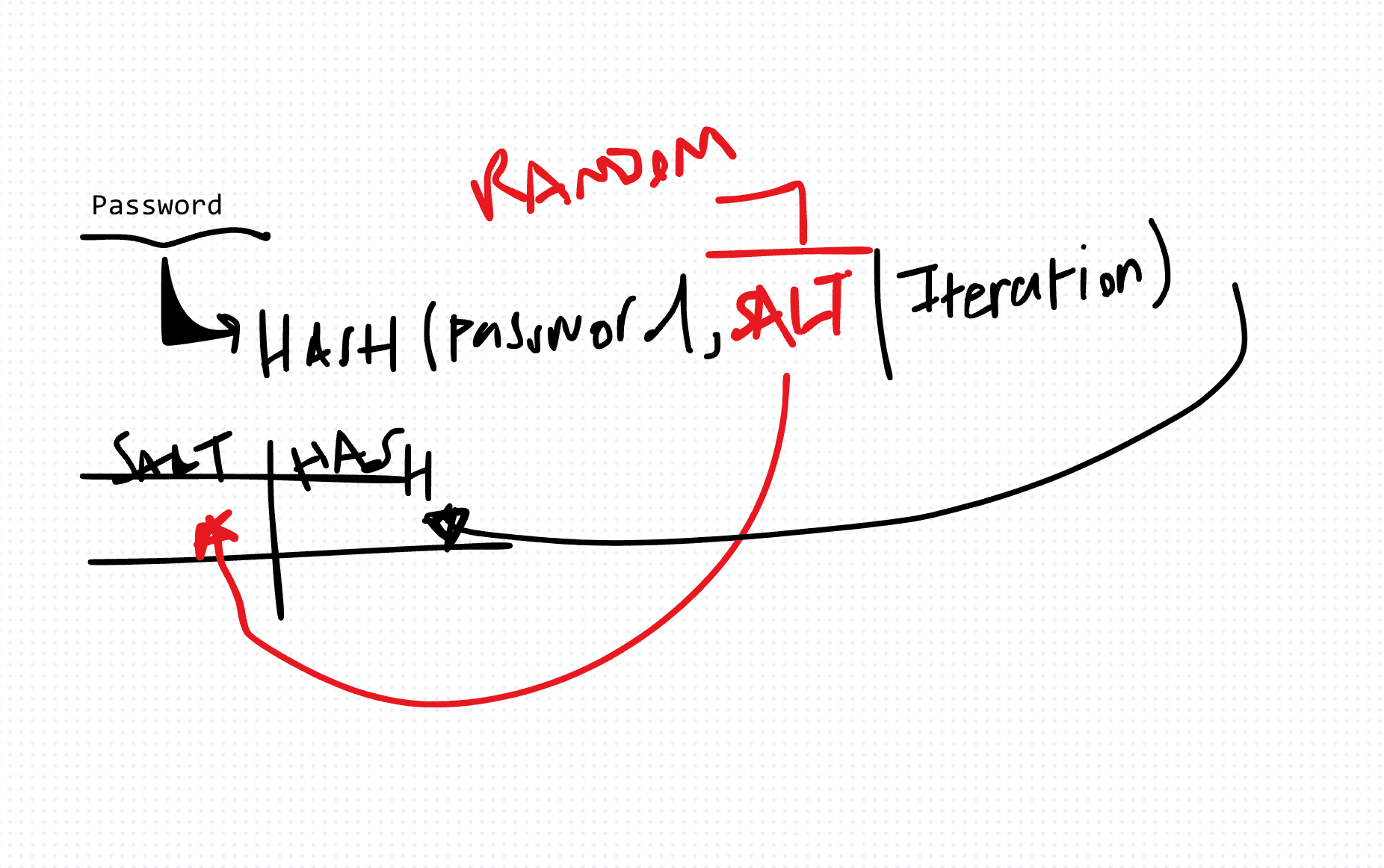
In the realm of cybersecurity, implementing salt with encryption is a fundamental practice to enhance the security of sensitive data. Salt adds an extra layer of protection by introducing randomness and uniqueness to encrypted data, making it significantly more challenging for attackers to decipher the original information. In this article, we'll delve into how to implement salt with encryption using TypeScript, along with practical examples to illustrate the process.
A salt is a randomly generated value that is added to the data before encryption. It serves as additional input to the encryption algorithm, ensuring that even identical plaintext inputs produce different ciphertext outputs.
import crypto from 'crypto';
const generateSalt = (length: number = 16): string => {
return crypto.randomBytes(Math.ceil(length / 2)).toString('hex').slice(0, length);
};
const encryptWithSalt = (data: string, salt: string): string => {
const hashedSalt = crypto.createHash('sha256').update(salt).digest('hex');
const cipher = crypto.createCipher('aes192', hashedSalt);
let encryptedData = cipher.update(data, 'utf8', 'hex');
encryptedData += cipher.final('hex');
return encryptedData;
};
const plaintextData = 'Sensitive information';
const salt = generateSalt();
const encryptedData = encryptWithSalt(plaintextData, salt);
console.log('Encrypted Data:', encryptedData);
console.log('Salt:', salt);
You can use a same salt, but best practice to save the salt next to hashed password on the salt in the database, it's pretty difficult to decrypt this then.
Implementing salt with encryption is essential for strengthening the security of sensitive data in applications. By incorporating random and unique salts into the encryption process, developers can mitigate the risk of brute-force and rainbow table attacks, thereby enhancing data protection. In TypeScript, leveraging cryptographic libraries like Node.js crypto module facilitates seamless integration of salted encryption into applications, ensuring robust security measures against potential threats. Experiment with the provided examples and explore further enhancements to fortify your data encryption practices in TypeScript-based projects.