Enums in TypeScript: A Comprehensive Guide
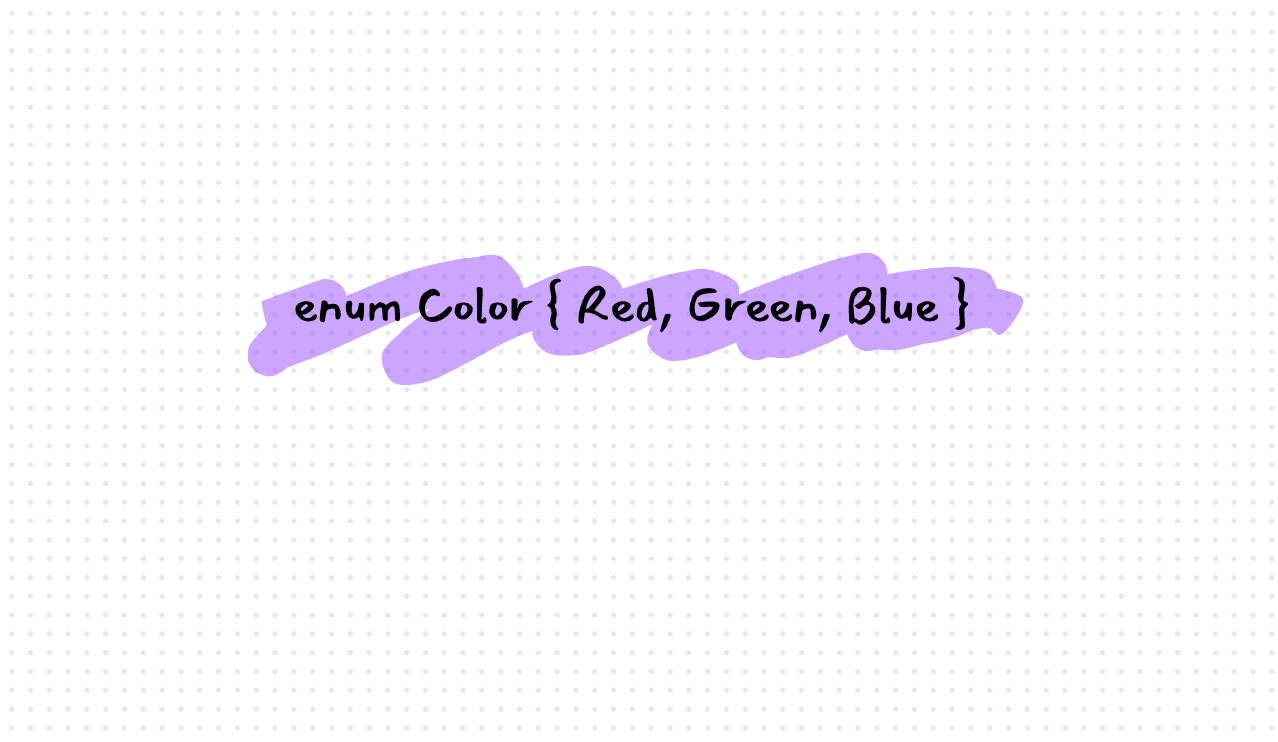
An enum is a way to define a set of named values. Enums are a way to define a set of constants that can be used throughout an application. Enums are useful when you need to define a set of values that can be used in your code.
enum Color {
Red,
Green,
Blue
}
function getColorName(color: Color) {
switch (color) {
case Color.Red:
return 'Red';
case Color.Green:
return 'Green';
case Color.Blue:
return 'Blue';
}
}
console.log(getColorName(Color.Red)));
In this article, we have explored the concept of enums in TypeScript and provided examples to illustrate how they work. Enums are a powerful tool that can be used to define a set of named values. By using enums, you can write more readable, maintainable, and reusable code that is easier to debug and maintain.