Debugging TypeScript Applications: Lessons from the Trenches
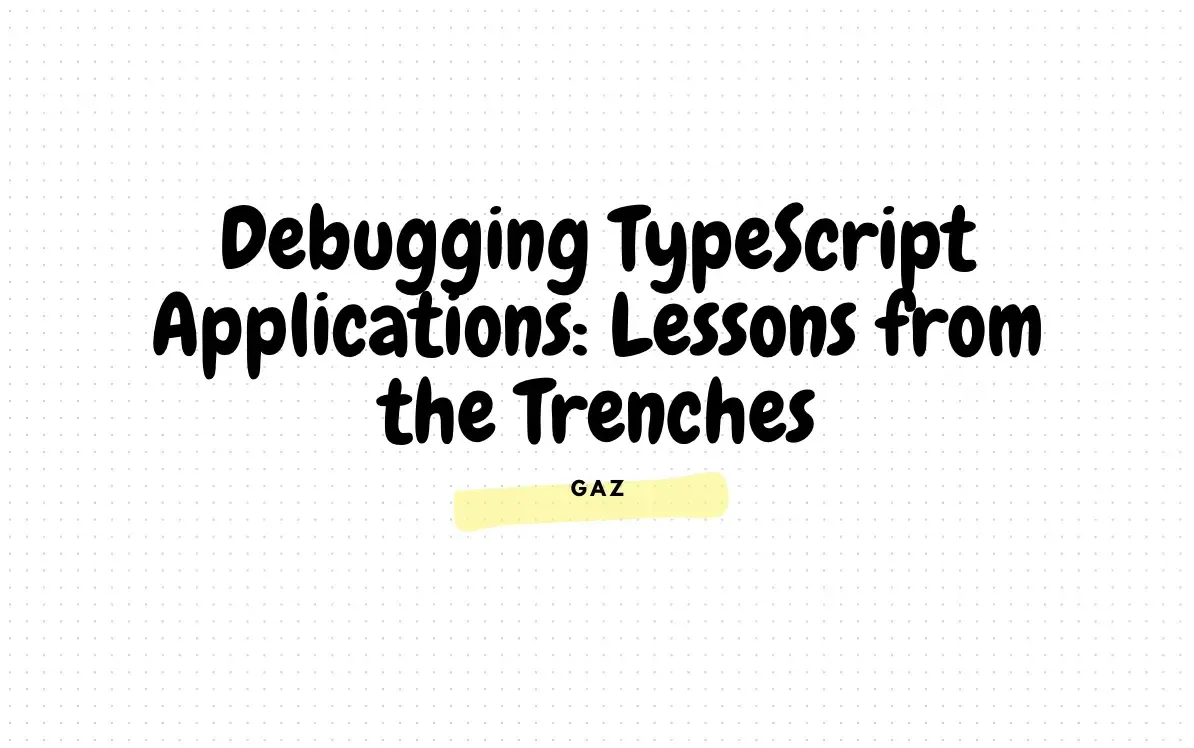
Debugging is an inevitable part of the development process. While TypeScript’s static typing helps catch many errors at compile time, bugs can still creep in, especially in larger applications. Drawing from my experience as a Principal Engineer, this article explores practical debugging techniques and tools to streamline your debugging workflow in TypeScript projects.
1. Type Errors in Complex Types
As your application grows, you might encounter situations where complex types fail unexpectedly. For example:
type User = {
id: number;
name: string;
};
type Admin = User & {
permissions: string[];
};
const getUser = (user: User) => {
console.log(user.name);
};
// Error: Argument of type 'Admin' is not assignable to parameter of type 'User'.
const admin: Admin = { id: 1, name: "Ehsan", permissions: ["read"] };
getUser(admin);
In this case, the intersection type caused subtle incompatibilities. Using TypeScript’s type guards or narrowing techniques can help:
const isUser = (input: any): input is User => {
return "id" in input && "name" in input;
};
if (isUser(admin)) {
getUser(admin);
}
2. Debugging undefined or null Values
TypeScript’s strict null checks (“strictNullChecks”) are invaluable for avoiding runtime issues. However, debugging nullish values can still be tricky.
const getUserName = (user: User | null): string => {
// Error: Cannot read properties of null
return user.name;
};
Solution: Use optional chaining and nullish coalescing operators.
const getUserName = (user: User | null): string => {
return user?.name ?? "Guest";
};
Debugging Tools and Techniques
1. Debugging with VS Code
VS Code provides excellent support for debugging TypeScript. Here’s how you can set it up:
Configure Source Maps
Ensure that your tsconfig.json includes the following:
{
"compilerOptions": {
"sourceMap": true
}
}
Launch Configuration
Add a debug configuration in .vscode/launch.json:
{
"version": "0.2.0",
"configurations": [
{
"type": "node",
"request": "launch",
"name": "Launch Program",
"skipFiles": ["<node_internals>/**"],
"program": "${workspaceFolder}/dist/index.js"
}
]
}
Set Breakpoints
Place breakpoints directly in your TypeScript files. VS Code will map them to the corresponding JavaScript during runtime.
2. Debugging with Chrome DevTools
For frontend TypeScript applications, Chrome DevTools can be a powerful ally.
Compile your TypeScript code with source maps enabled.
Open your application in Chrome.
Navigate to the “Sources” tab, and you’ll see your original TypeScript files listed under webpack:// or a similar namespace.
Add breakpoints and inspect variables directly in your TypeScript code.
3. Using console.log Wisely
While console.log may seem basic, it’s often the quickest way to debug. However, you can enhance its effectiveness:
Use console.table for logging arrays or objects:
const users: User[] = [
{ id: 1, name: "Ehsan" },
{ id: 2, name: "John" },
];
console.table(users);
Use string interpolation for clearer context:
console.log(`User ID: ${user.id}, Name: ${user.name}`);
4. Employing Linters and Static Analysis
Linters like ESLint with TypeScript plugins can catch potential issues before they even run. For instance:
Install ESLint:
npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
Configure .eslintrc.json:
{
"parser": "@typescript-eslint/parser",
"plugins": ["@typescript-eslint"],
"rules": {
"@typescript-eslint/no-unused-vars": "error",
"@typescript-eslint/explicit-function-return-type": "warn"
}
}
Run linting:
npx eslint src/**/*.ts
5. Analyzing Stack Traces
TypeScript’s stack traces can sometimes be verbose. Use tools like ts-stacktrace to simplify and map stack traces back to TypeScript files.
Debugging Best Practices
- Log Strategically: Remove or replace console.log with proper logging libraries like winston or pino in production.
- Write Unit Tests: Tests can help you catch bugs early and serve as a form of documentation.
- Divide and Conquer: Isolate problematic code and test in smaller units.
- Leverage Community Tools: Tools like TypeScript Hero and WebStorm offer additional debugging enhancements.
Final Thoughts
Debugging is both an art and a science. By combining the right tools, strategies, and best practices, you can make the process less daunting and more efficient. TypeScript’s robust type system is a great ally, but knowing how to debug effectively ensures that your applications are not only type-safe but also free from runtime issues.
Let me know if you’ve found these techniques helpful or if you’d like me to explore a specific debugging scenario in depth!