Testing setTimeout Functions in React Components
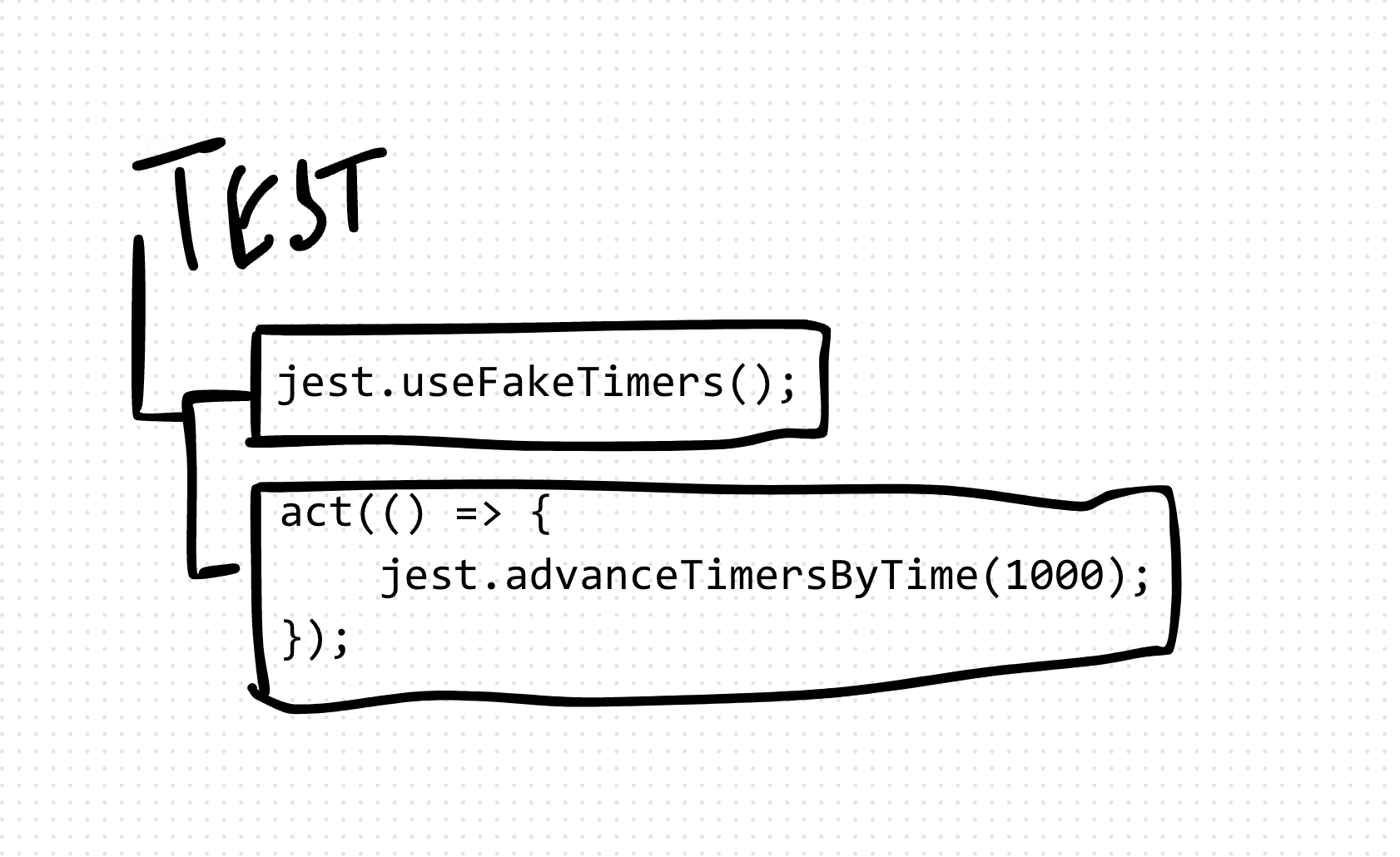
Testing React components involves not only verifying their rendered output but also ensuring that their methods and functionalities behave as expected. In this article, we'll explore how to test methods of React components using TypeScript, along with examples.
Writing Tests for setTimeout:
- Mocking setTimeout: Use Jest's jest.useFakeTimers() function to mock the setTimeout function. This allows you to control the passage of time in your tests.
- Invoke the Component Method: Invoke the component method that contains the setTimeout function you want to test.
- Advance Timers: Use Jest's jest.advanceTimersByTime() function to advance the timers by the desired amount of time. This simulates the passage of time and triggers the setTimeout callback.
- Assert Expected Behavior: Use Jest's assertions to verify that the setTimeout callback was called and executed as expected.
import React, { useState } from 'react';
const DelayedMessage: React.FC = () => {
const [message, setMessage] = useState('');
const showMessage = () => {
setTimeout(() => {
setMessage('Hello, World!');
}, 1000);
};
return (
<div>
<button onClick={showMessage}>Show Message</button>
<p>{message}</p>
</div>
);
};
export default DelayedMessage;
And Testing:
import React from 'react';
import { render, fireEvent, act } from '@testing-library/react';
import DelayedMessage from './DelayedMessage';
describe('DelayedMessage Component', () => {
it('displays the message after a delay', async () => {
jest.useFakeTimers();
const { getByText } = render(<DelayedMessage />);
const button = getByText('Show Message');
fireEvent.click(button);
// Advance timers by 1 second
act(() => {
jest.advanceTimersByTime(1000);
});
expect(getByText('Hello, World!')).toBeInTheDocument();
});
});
Testing setTimeout functions in React components requires careful handling of asynchronous behavior and timer manipulation. By using Jest's timer functions and async testing utilities, you can effectively test the behavior of components that rely on setTimeout for delayed actions. Remember to mock timers, advance timers in your tests, and assert the expected behavior to ensure the reliability of your tests.