Understanding Database Aggregates in TypeScript
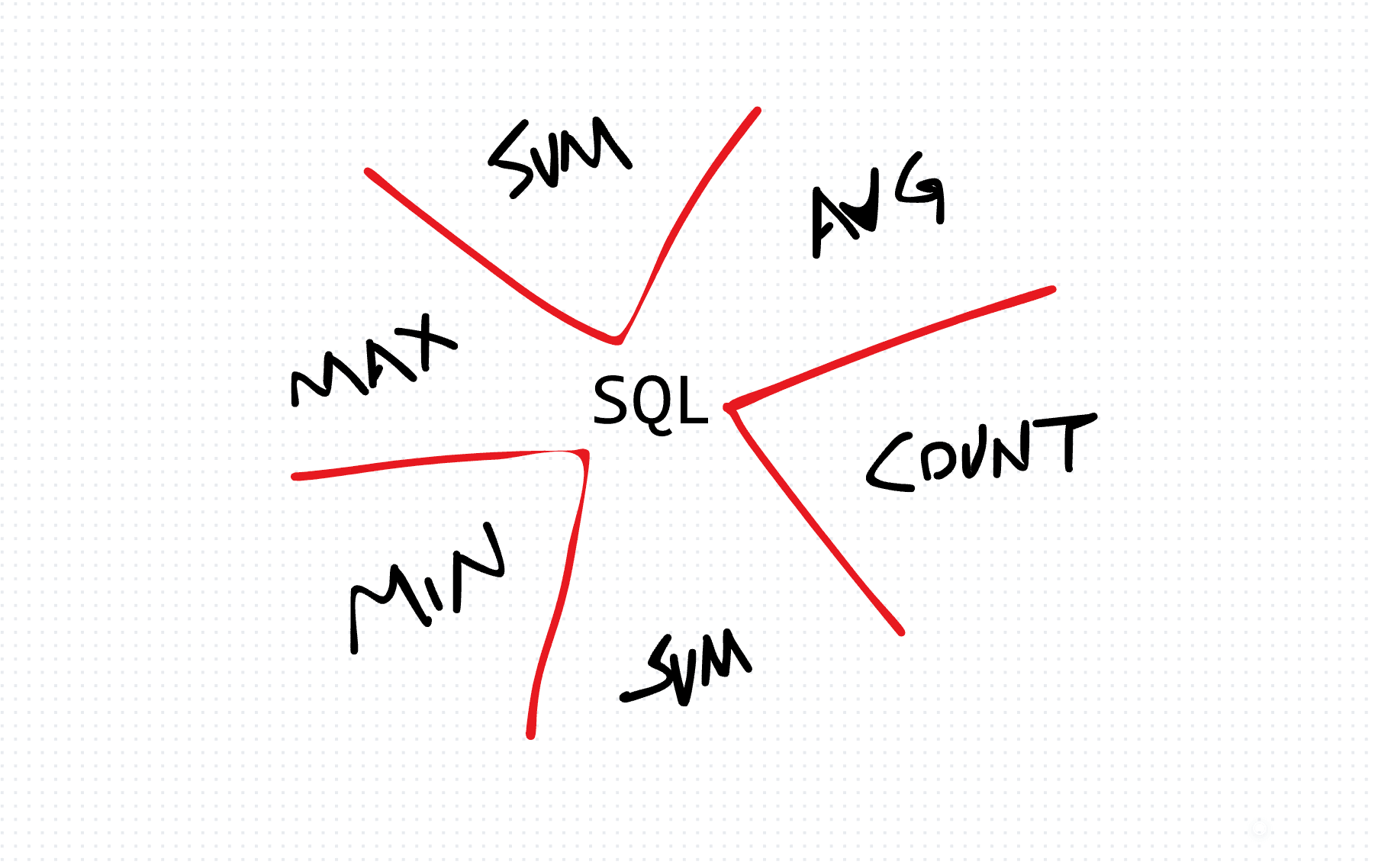
Database aggregates are functions that operate on sets of rows or values within a database table. Instead of returning individual rows, aggregate functions return a single value that summarizes the data within the specified set. These functions are commonly used for tasks such as calculating totals, averages, counts, minimum and maximum values, and other summary statistics.
COUNT:
Counts the number of rows or non-null values in a column.
const countQuery = `SELECT COUNT(*) AS total_users FROM users`;
SUM:
Calculates the sum of numeric values in a column.
const sumQuery = `SELECT SUM(sales_amount) AS total_sales FROM sales`;
AVG:
Computes the average value of numeric values in a column.
const avgQuery = `SELECT AVG(age) AS avg_age FROM employees`;
MIN:
Returns the minimum value in a column.
const minQuery = `SELECT MIN(price) AS min_price FROM products`;
MAX:
Returns the maximum value in a column.
const maxQuery = `SELECT MAX(score) AS max_score FROM exam_results`;
In TypeScript, we can execute SQL queries containing aggregate functions using database libraries such as TypeORM or Sequelize. Here's an example using TypeORM:
import { getConnection } from 'typeorm';
async function getTotalUsers(): Promise<number> {
const connection = getConnection();
const queryResult = await connection.query(`SELECT COUNT(*) AS total_users FROM users`);
return queryResult[0].total_users;
}
getTotalUsers().then((totalUsers) => {
console.log(`Total users: ${totalUsers}`);
});
Database aggregates are powerful tools for summarizing and analyzing data in TypeScript applications. By leveraging aggregate functions such as COUNT, SUM, AVG, MIN, and MAX, developers can gain valuable insights into their data and make informed decisions. Understanding how to use database aggregates effectively is essential for building robust and efficient database-driven applications in TypeScript.