Optimizing Script Loading in Web Development: Strategies and Techniques
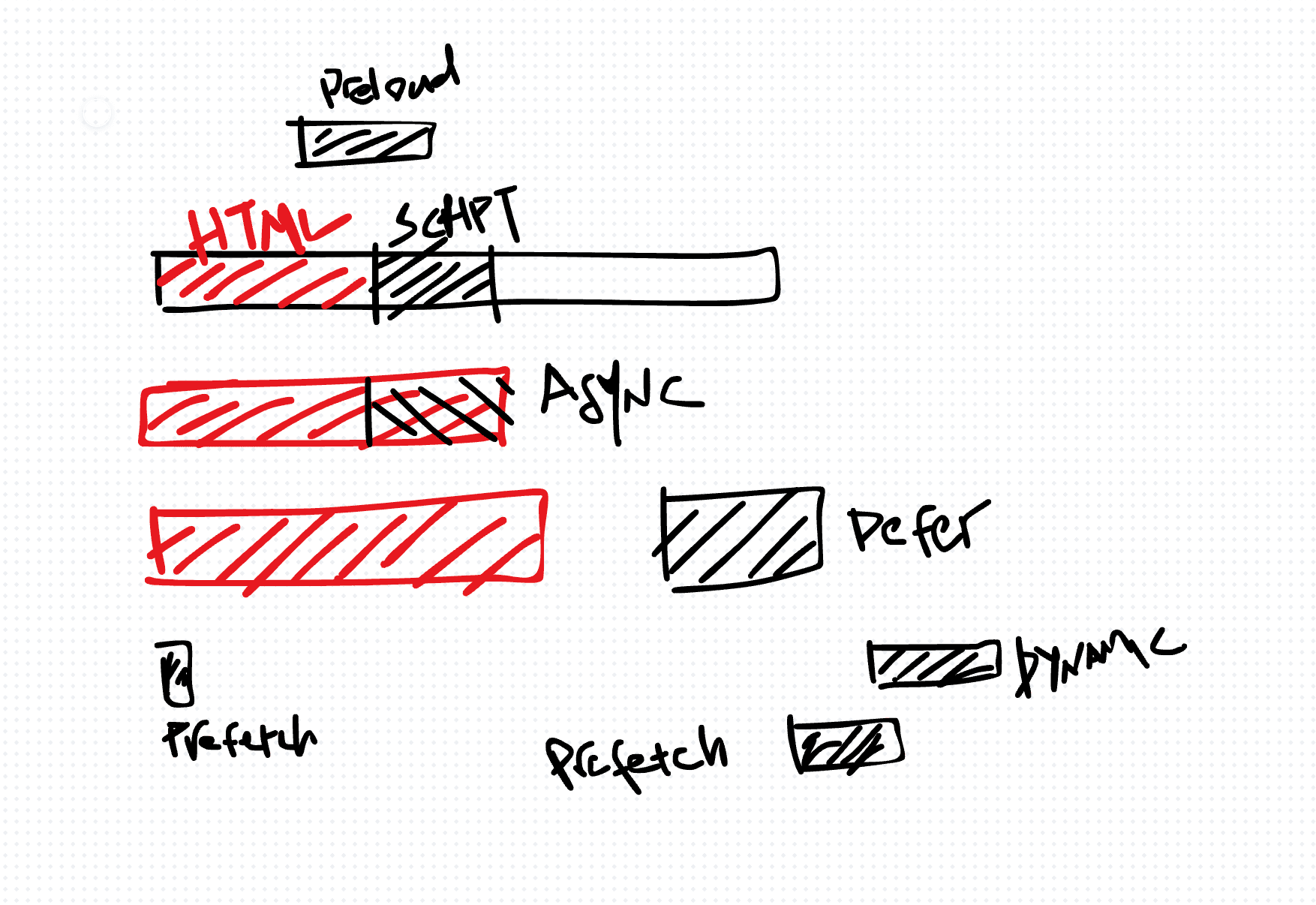
In web development, optimizing script loading is crucial for enhancing web performance and delivering a seamless user experience. By carefully controlling how and when scripts are added to a page, developers can minimize load times and improve overall performance. In this article, we'll explore different strategies for optimizing script loading and provide an example of lazy loading using TypeScript.
1. Inline Scripts:
Inline scripts are directly embedded within HTML documents using the <script> tag. While convenient, they can hinder performance as they block HTML parsing and rendering. It's best to reserve inline scripts for small, non-blocking tasks.
<script>
// Inline script code here
</script>
2. External Scripts with async Attribute:
External scripts with the async attribute are downloaded asynchronously, allowing them to execute independently of HTML parsing. This can improve page load times, especially for non-essential scripts.
<script src="script.js" async></script>
3. External Scripts with defer Attribute:
Scripts with the defer attribute are also downloaded asynchronously but are executed only after the HTML document has been fully parsed, ensuring they do not block rendering.
<script src="script.js" defer></script>
4. Dynamic Script Loading:
Dynamic script loading using JavaScript gives developers more control over when and how scripts are added to the page. This approach allows for conditional loading based on user interactions or other factors.
const script = document.createElement('script');
script.src = 'script.js';
document.body.appendChild(script);
5. Preloading and Prefetching:
The <link> tag can be used to preload or prefetch resources, including scripts, improving performance by initiating early downloading.
<link rel="preload" href="script.js" as="script"
<link rel="prefetch" href="script.js">
Preload is used to specify resources that are crucial for the current page and should be fetched as soon as possible, even before they are needed. It hints to the browser that the specified resource is required for rendering the current page.
Prefetch is used to specify resources that are likely to be needed for future navigation or interaction, such as resources for subsequent pages or user actions. It suggests to the browser that the specified resource may be needed in the near future.
6. Lazy Loading with TypeScript:
Lazy loading involves deferring the loading of non-essential scripts until they are needed. Below is an example of lazy loading using TypeScript.
const loadScript = (url: string) => {
return new Promise((resolve, reject) => {
const script = document.createElement('script');
script.src = url;
script.async = true;
script.onload = resolve;
script.onerror = reject;
document.body.appendChild(script);
});
};
loadScript('lazy-loaded-script.js')
.then(() => {
console.log('Lazy-loaded script loaded successfully');
})
.catch((error) => {
console.error('Error loading lazy-loaded script:', error);
});
7. Link rel preconenct
The rel="preconnect" attribute in HTML is used to instruct the browser to initiate an early connection to a specified resource's origin server. This helps to reduce the connection latency when fetching resources from external domains, such as fonts, scripts, stylesheets, or APIs.
<link rel="preconnect" href="https://example.com">
Of course you can use `dns-prefetch` to just check for dns as well
<link rel="dns-prefetch" href="https://example.com">
By employing various strategies such as async loading, defer attributes, and lazy loading, developers can optimize script loading to improve web performance significantly. By carefully considering how and when scripts are added to a page, developers can create faster and more efficient web experiences for users. Experiment with different approaches and monitor performance metrics to find the best solution for your specific use case.