Lazy Loading in HTML and CSS: Enhancing Performance and User Experience
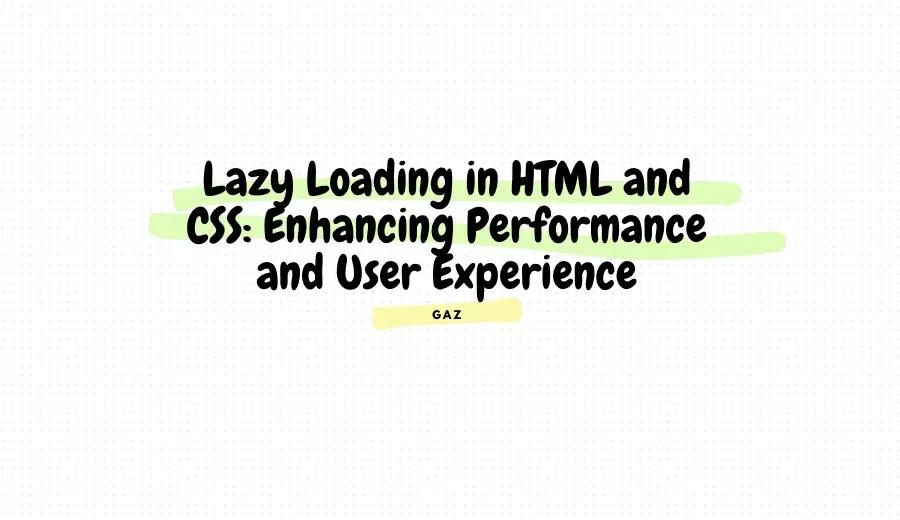
Lazy loading is a powerful technique to improve web performance by deferring the loading of non-critical resources until they are needed. Over the years, I’ve implemented lazy loading in various projects, and it has proven to be a game-changer for both performance and user experience. In this article, I’ll share my experience with lazy loading in HTML and CSS, along with practical examples.
Why Use Lazy Loading?
Lazy loading offers several benefits:
- Improved Page Load Speed: By loading only critical resources initially, the page becomes interactive faster.
- Reduced Bandwidth Usage: Only necessary assets are downloaded, saving data for users.
- Enhanced User Experience: By prioritizing visible content, users get a smoother experience.
Lazy Loading in HTML
Images with the loading Attribute
One of the simplest ways to implement lazy loading is by using the loading="lazy" attribute on images and iframes. Here’s an example:
<!DOCTYPE html>
<html lang="en">
<head>
<title>Lazy Loading Example</title>
</head>
<body>
<h1>Lazy Loading Images</h1>
<img src="image1.jpg" alt="Image 1" loading="lazy">
<img src="image2.jpg" alt="Image 2" loading="lazy">
</body>
</html>
Lazy Loading Iframes
For embedded content, such as YouTube videos, lazy loading can significantly reduce the initial load time:
<IFRAMETAG
width="560"
height="315"
src="https://www.youtube.com/embed/dQw4w9WgXcQ"
title="YouTube video player"
frameborder="0"
allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture"
allowfullscreen
loading="lazy">
</IFRAMETAG>
JavaScript-Based Lazy Loading
For more advanced use cases, such as lazy loading background images or custom components, JavaScript provides greater control:
const lazyImages = document.querySelectorAll('img[data-src]');
const loadImages = (image) => {
image.src = image.dataset.src;
};
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
loadImages(entry.target);
observer.unobserve(entry.target);
}
});
});
lazyImages.forEach(image => {
observer.observe(image);
});
HTML:
<img data-src="image3.jpg" alt="Lazy Loaded Image">
Lazy Loading in CSS
Background Images
CSS alone does not have built-in support for lazy loading background images. However, combining CSS with JavaScript achieves the desired effect.
.lazy-bg {
background-color: #f3f3f3;
background-image: none;
transition: background-image 0.3s ease-in-out;
}
.lazy-bg.loaded {
background-image: url('background.jpg');
}
JavaScript:
const lazyBackground = document.querySelector('.lazy-bg');
const loadBackground = (element) => {
element.classList.add('loaded');
};
const bgObserver = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
loadBackground(entry.target);
observer.unobserve(entry.target);
}
});
});
bgObserver.observe(lazyBackground);
HTML:
<div class="lazy-bg"></div>
Lazy Loading Fonts
Fonts can also be lazy-loaded using the font-display property:
@font-face {
font-family: 'CustomFont';
src: url('custom-font.woff2') format('woff2');
font-display: swap;
}
body {
font-family: 'CustomFont', sans-serif;
}
This ensures text is displayed with a fallback font while the custom font is loading.
Best Practices for Lazy Loading
- Test Thoroughly: Ensure lazy loading works across different devices and browsers.
- Prioritize Critical Content: Always load above-the-fold content eagerly.
- Monitor Performance: Use tools like Lighthouse to analyze the impact of lazy loading.
- Fallbacks: Provide fallbacks for browsers that don’t support native lazy loading.
Conclusion
Lazy loading is an essential technique for modern web development, offering tangible benefits in performance and user experience. By combining native HTML attributes, CSS tricks, and JavaScript, you can implement lazy loading effectively across various scenarios.
Have you implemented lazy loading in your projects? Share your thoughts or experiences below!