Strategy Design Pattern
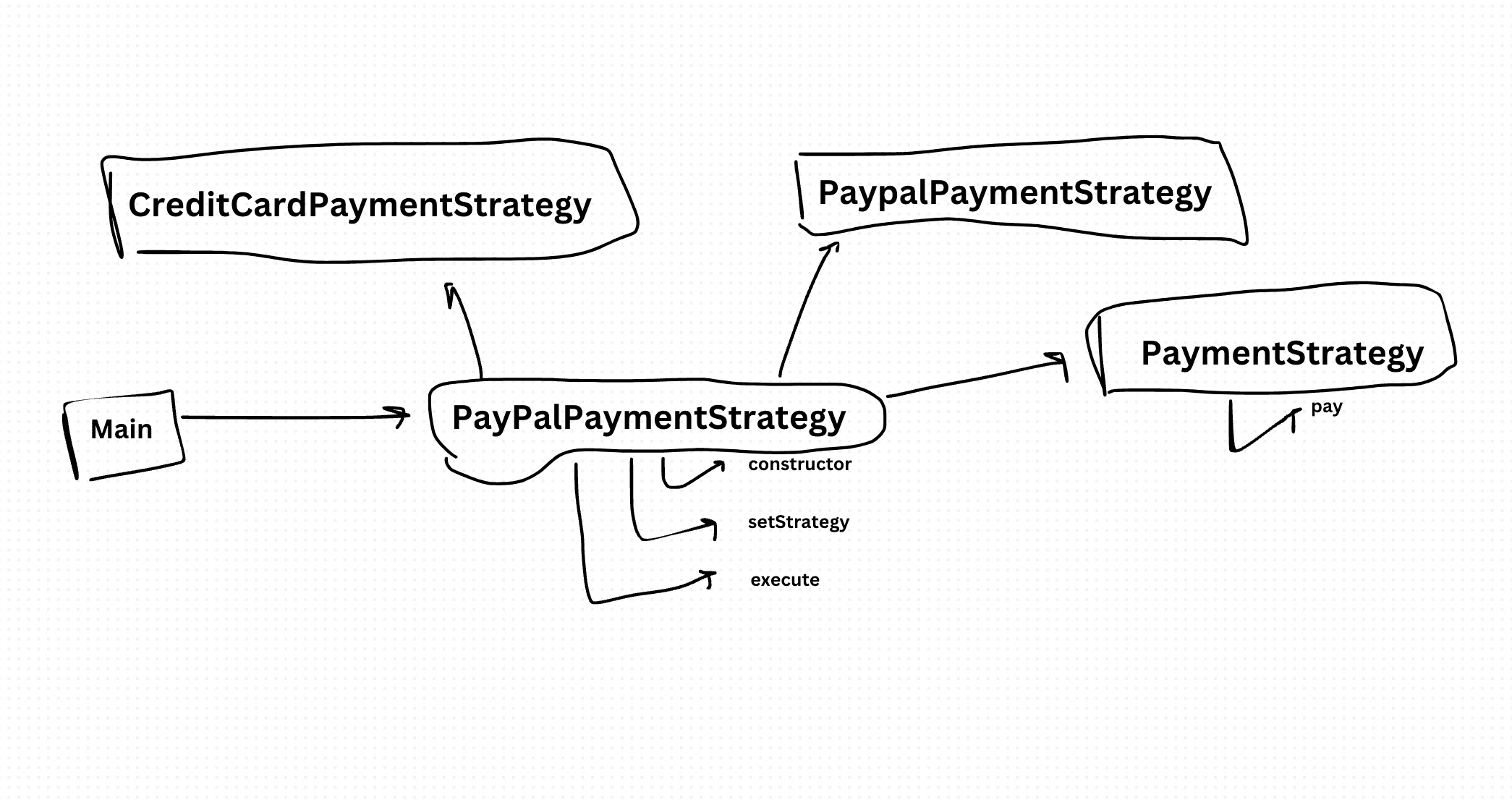
At its core, the Strategy Design Pattern consists of three main components: the Context, the Strategy Interface, and Concrete Strategies. The Context is the object that delegates the algorithmic responsibility to a Strategy object. The Strategy Interface defines a common interface for all supported algorithms, while Concrete Strategies implement specific algorithms. This pattern is particularly useful when different variations of an algorithm are needed, and the client should be able to choose between them dynamically.
// Strategy Interface
class PaymentStrategy {
pay(amount) {}
}
// Concrete Strategies
class CreditCardPaymentStrategy extends PaymentStrategy {
pay(amount) {
console.log(`Paid $${amount} via Credit Card`);
}
}
class PayPalPaymentStrategy extends PaymentStrategy {
pay(amount) {
console.log(`Paid $${amount} via PayPal`);
}
}
// Context
class PaymentContext {
constructor(strategy) {
this.strategy = strategy;
}
setStrategy(strategy) {
this.strategy = strategy;
}
executePayment(amount) {
this.strategy.pay(amount);
}
}
// Usage
const creditCardStrategy = new CreditCardPaymentStrategy();
const payPalStrategy = new PayPalPaymentStrategy();
const paymentContext = new PaymentContext(creditCardStrategy);
paymentContext.executePayment(100); // Paid $100 via Credit Card
paymentContext.setStrategy(payPalStrategy);
paymentContext.executePayment(50); // Paid $50 via PayPal
The Strategy Design Pattern offers a flexible and reusable solution for managing algorithms and behaviors in software systems. By encapsulating algorithms into separate strategy objects, developers can easily extend and modify the behavior of a system without affecting its clients. In JavaScript, the Strategy pattern is commonly used in scenarios such as payment processing, sorting algorithms, and validation strategies.