Singleton Design Pattern
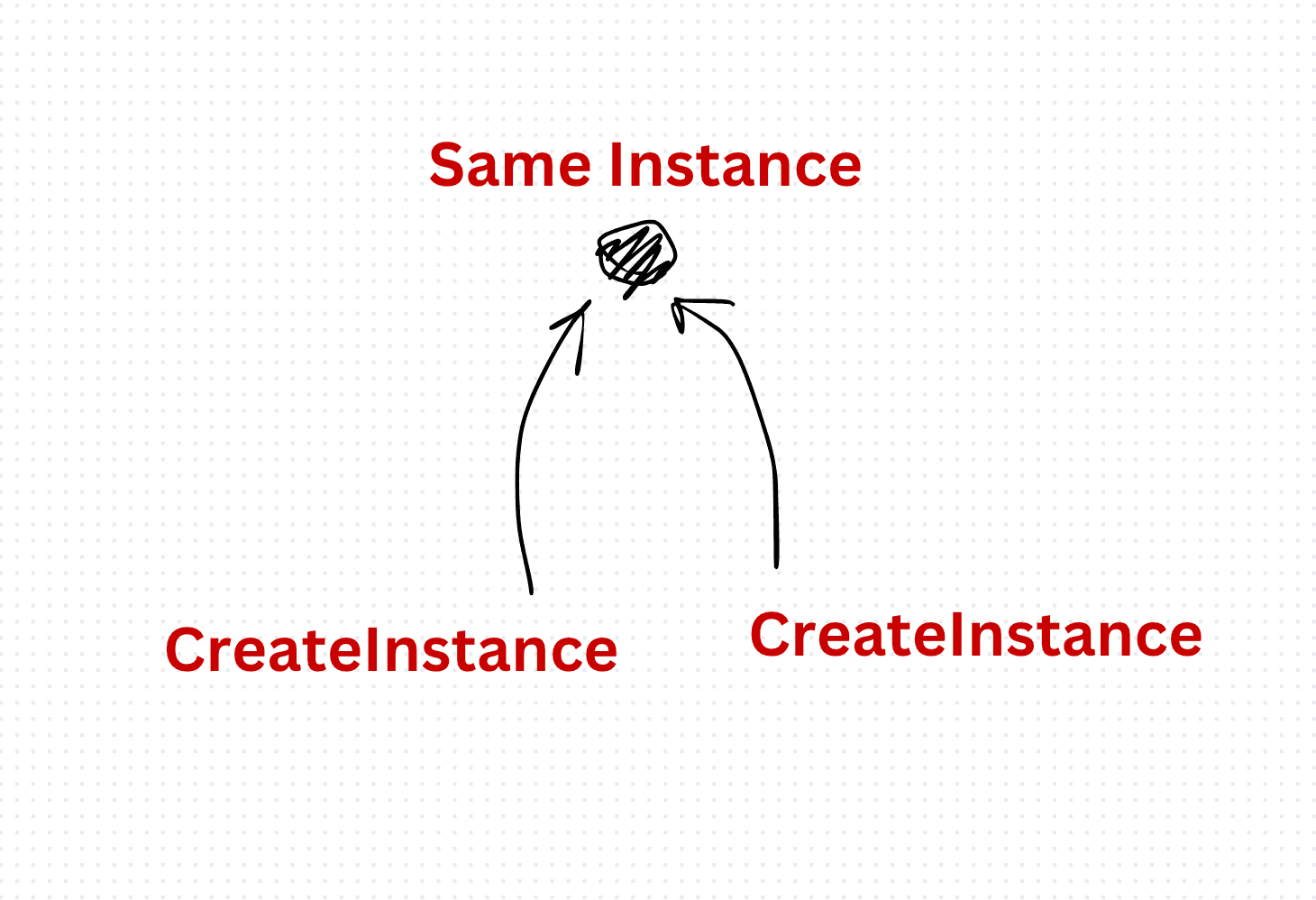
The Singleton Pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. In this article, we'll explore the Singleton Pattern in the context of JavaScript, along with a practical example.
Understanding the Singleton Pattern:
The Singleton Pattern is useful when exactly one object is needed to coordinate actions across the system. It is commonly used for logging, caching, thread pools, configuration settings, and more.
Implementation:
Let's dive into a simple implementation of the Singleton Pattern in JavaScript:
const Singleton = (function () {
let instance;
function createInstance() {
// Your singleton logic here
return {
method1: function () {
console.log("Method 1 called");
},
method2: function () {
console.log("Method 2 called");
},
};
}
return {
getInstance: function () {
if (!instance) {
instance = createInstance();
}
return instance;
},
};
})();
// Usage
const singletonInstance1 = Singleton.getInstance();
const singletonInstance2 = Singleton.getInstance();
console.log(singletonInstance1 === singletonInstance2);
The Singleton Pattern is a powerful tool for ensuring that only one instance of a class exists within an application. While it has its use cases, it's essential to use it judiciously to avoid potential drawbacks such as tight coupling and global state.