Proxy Design Pattern
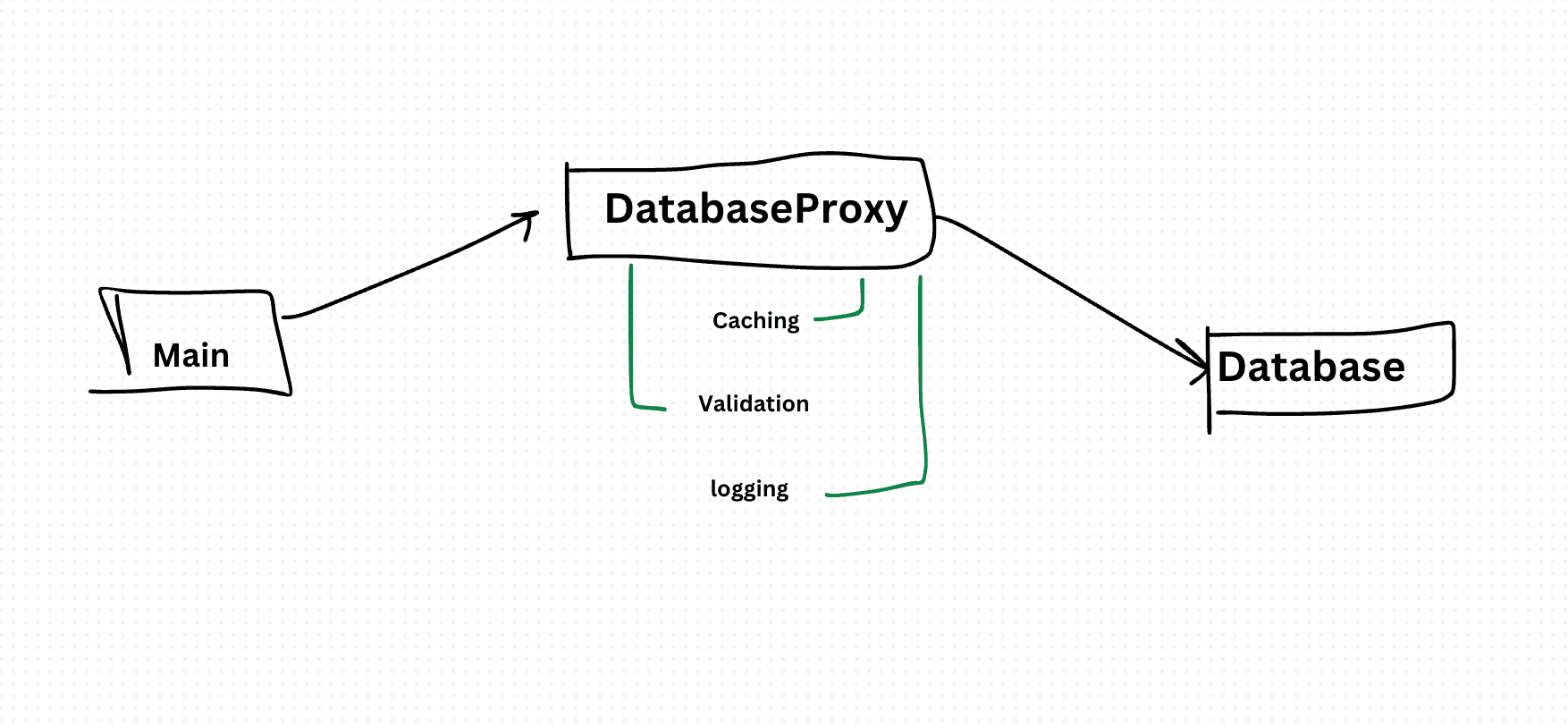
At its core, the Proxy Design Pattern enables the creation of a representative object that controls access to another object. It adds a layer of indirection, allowing for various operations such as validation, logging, caching, and lazy initialization to be performed before or after the target object's operations are executed. This pattern is particularly useful in scenarios where direct access to the target object needs to be restricted or augmented with additional functionality.
class Database {
constructor() {
this.data = { /* sensitive data */ };
}
fetchData() {
console.log("Fetching data...");
return this.data;
}
}
// Proxy
class DatabaseProxy {
constructor() {
this.database = new Database();
}
fetchData(user) {
if (user === "admin") {
console.log("Admin access granted.");
return this.database.fetchData();
} else {
console.log("Unauthorized access.");
return null;
}
}
}
The Proxy Design Pattern provides a powerful mechanism for controlling access to objects and augmenting their behavior with additional functionalities. By introducing a proxy between the client and the target object, developers can enforce security measures, implement caching, or perform other tasks without modifying the core functionality of the target object. In JavaScript, proxies offer a flexible and elegant solution for implementing cross-cutting concerns in a non-intrusive manner.