Model-View-Controller (MVC) Design Pattern
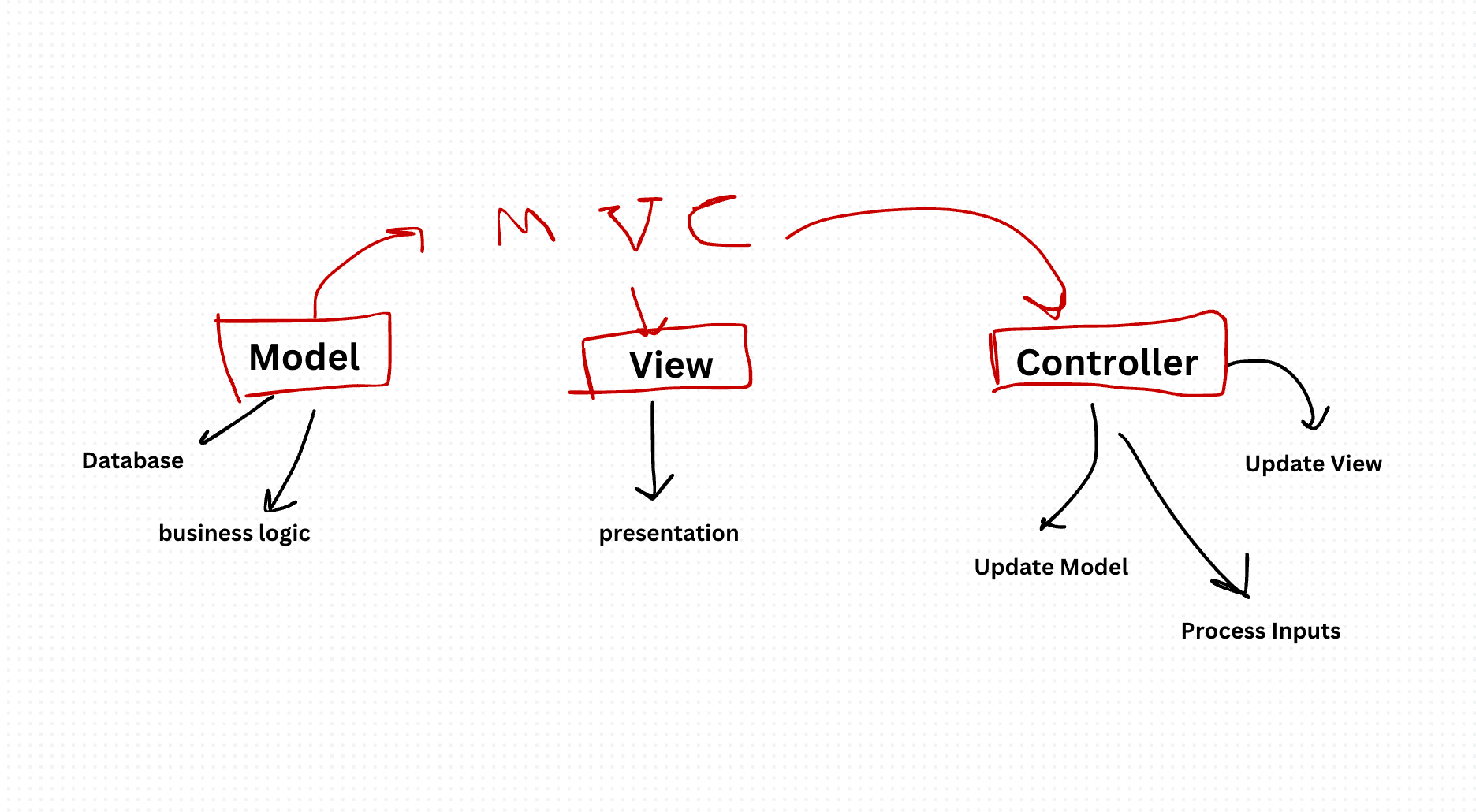
The Model-View-Controller (MVC) design pattern is a widely-used architectural pattern for designing and developing user interfaces in software applications. It divides an application into three interconnected components – Model, View, and Controller – each with distinct responsibilities. In this article, we'll explore the concepts behind the MVC pattern and demonstrate its implementation in JavaScript with practical examples.
The MVC pattern separates the concerns of an application into three main components:
- Model: The Model represents the application's data and business logic. It encapsulates the data and provides methods to manipulate and retrieve it. The Model notifies the View of any changes in the data.
// Model
class TaskModel {
constructor() {
this.tasks = [];
}
addTask(task) {
this.tasks.push(task);
this.notifyObservers();
}
notifyObservers() {
// Notify views of changes in the model
TaskView.renderTasks(this.tasks);
}
}
- View: The View represents the presentation layer of the application. It is responsible for rendering the user interface based on the data provided by the Model. The View observes changes in the Model and updates itself accordingly.
class TaskView {
static renderTasks(tasks) {
const taskList = document.getElementById('task-list');
taskList.innerHTML = '';
tasks.forEach(task => {
const listItem = document.createElement('li');
listItem.textContent = task;
taskList.appendChild(listItem);
});
}
}
- Controller: The Controller acts as an intermediary between the Model and the View. It receives user input from the View, processes it, and updates the Model accordingly. The Controller also updates the View in response to changes in the Model.
class TaskController {
constructor(model) {
this.model = model;
}
addTask(task) {
this.model.addTask(task);
}
}
The Model-View-Controller (MVC) design pattern is a powerful architectural pattern for developing user interfaces in JavaScript applications. By separating concerns into distinct components – Model, View, and Controller – the MVC pattern promotes modularity, maintainability, and testability. In this article, we explored the concepts behind the MVC pattern and demonstrated its implementation in JavaScript with a simple task management application.