Mediator Design Pattern
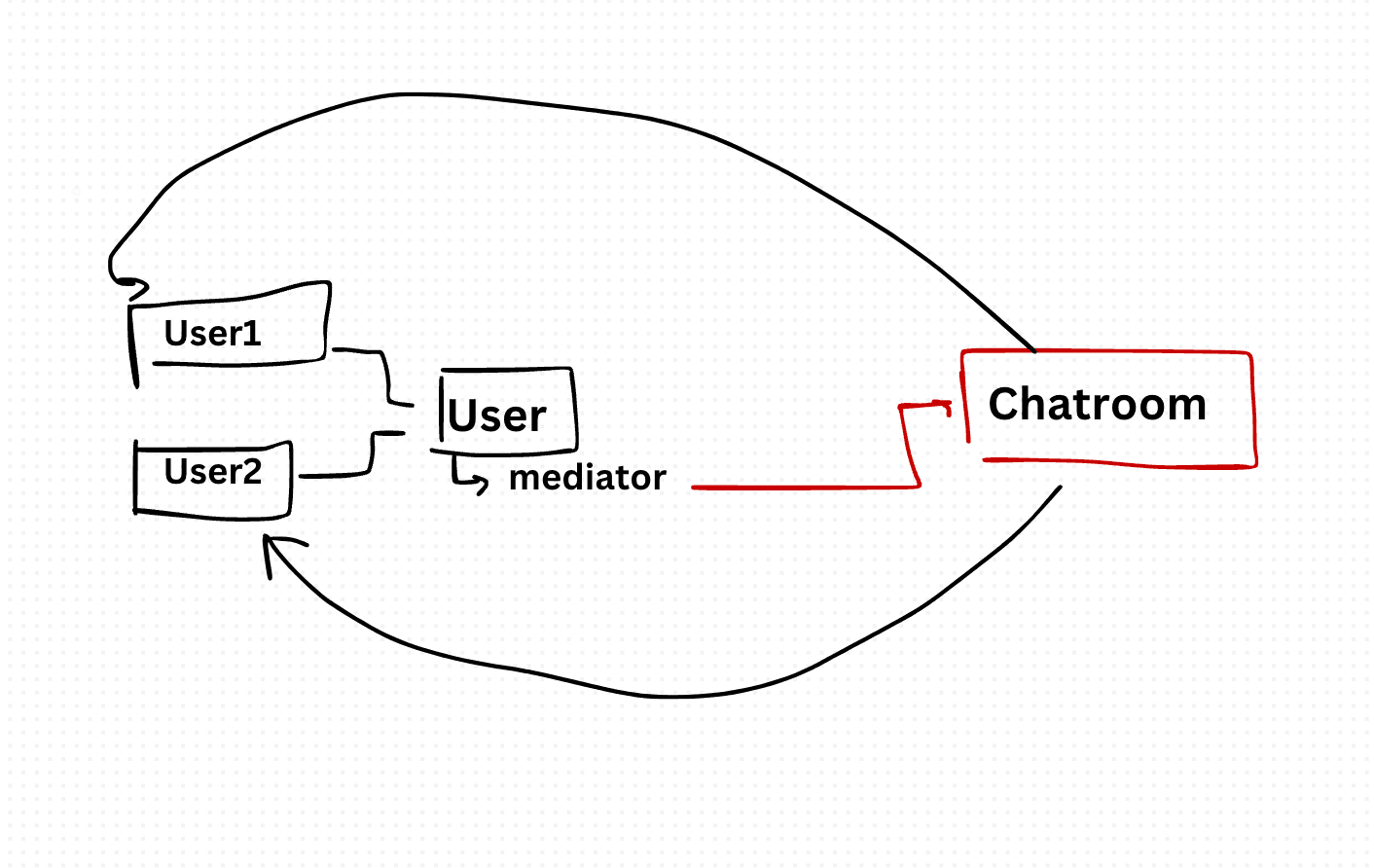
At its core, the Mediator Design Pattern consists of three main components: the Mediator, Colleagues, and Concrete Colleagues. The Mediator defines an interface for communicating with Colleagues, while Colleagues are objects that need to communicate with each other. Concrete Colleagues implement specific behavior and interact with the Mediator to facilitate communication. This pattern promotes decoupling of objects and simplifies complex communication scenarios by centralizing control and coordination.
// Mediator
class ChatRoom {
sendMessage(user, message) {
console.log(`[${user.getName()}]: ${message}`);
}
}
// Colleague
class User {
constructor(name, mediator) {
this.name = name;
this.mediator = mediator;
}
getName() {
return this.name;
}
sendMessage(message) {
this.mediator.sendMessage(this, message);
}
}
// Usage
const mediator = new ChatRoom();
const user1 = new User("Alice", mediator);
const user2 = new User("Bob", mediator);
user1.sendMessage("Hello, Bob!"); // [Alice]: Hello, Bob!
user2.sendMessage("Hi, Alice!"); // [Bob]: Hi, Alice!
The Mediator Design Pattern provides an elegant solution for managing complex communication scenarios and promoting loose coupling between objects. By centralizing communication logic within a mediator object, this pattern simplifies interactions and enhances flexibility and maintainability in software systems. In JavaScript, the Mediator pattern is commonly used in scenarios such as event handling, UI frameworks, and distributed systems.