Iterator Design Pattern
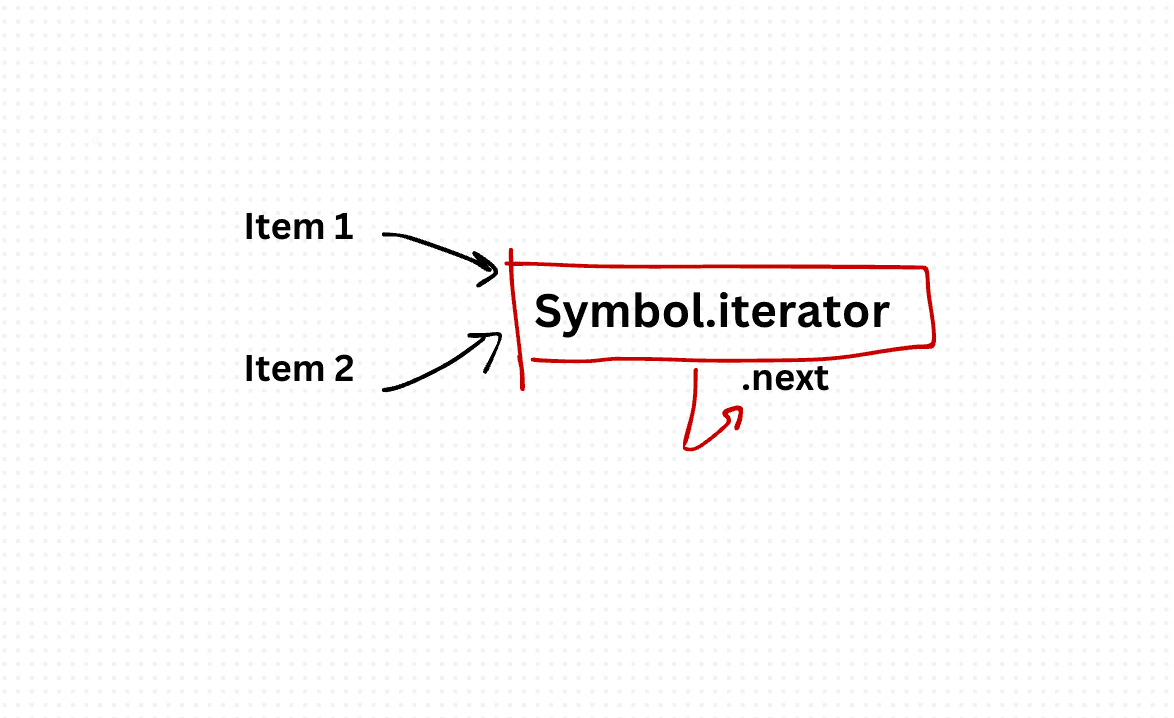
At its core, the Iterator Design Pattern consists of two main components: the Iterator and the Iterable. The Iterator defines methods for accessing elements of a collection sequentially, while the Iterable provides a way to obtain an Iterator instance for traversing the collection. This pattern promotes separation of concerns by encapsulating traversal logic within Iterator objects, making collections iterable without exposing their internal structure.
// Iterable
class List {
constructor() {
this.items = [];
}
add(item) {
this.items.push(item);
}
[Symbol.iterator]() {
let index = 0;
const items = this.items;
return {
next: function() {
return index < items.length
? { value: items[index++], done: false }
: { done: true };
}
};
}
}
// Usage
const list = new List();
list.add("Item 1");
list.add("Item 2");
list.add("Item 3");
const iterator = list[Symbol.iterator]();
let result = iterator.next();
while (!result.done) {
console.log(result.value);
result = iterator.next();
}
The Iterator Design Pattern provides a convenient and standardized way to traverse collections of various types without exposing their internal structure. By encapsulating traversal logic within iterator objects, this pattern promotes separation of concerns and enables the creation of reusable traversal algorithms. In JavaScript, the Iterator pattern is commonly used in scenarios such as iterating over arrays, sets, maps, and custom data structures.