Higher-Order Component (HOC) React Design Pattern
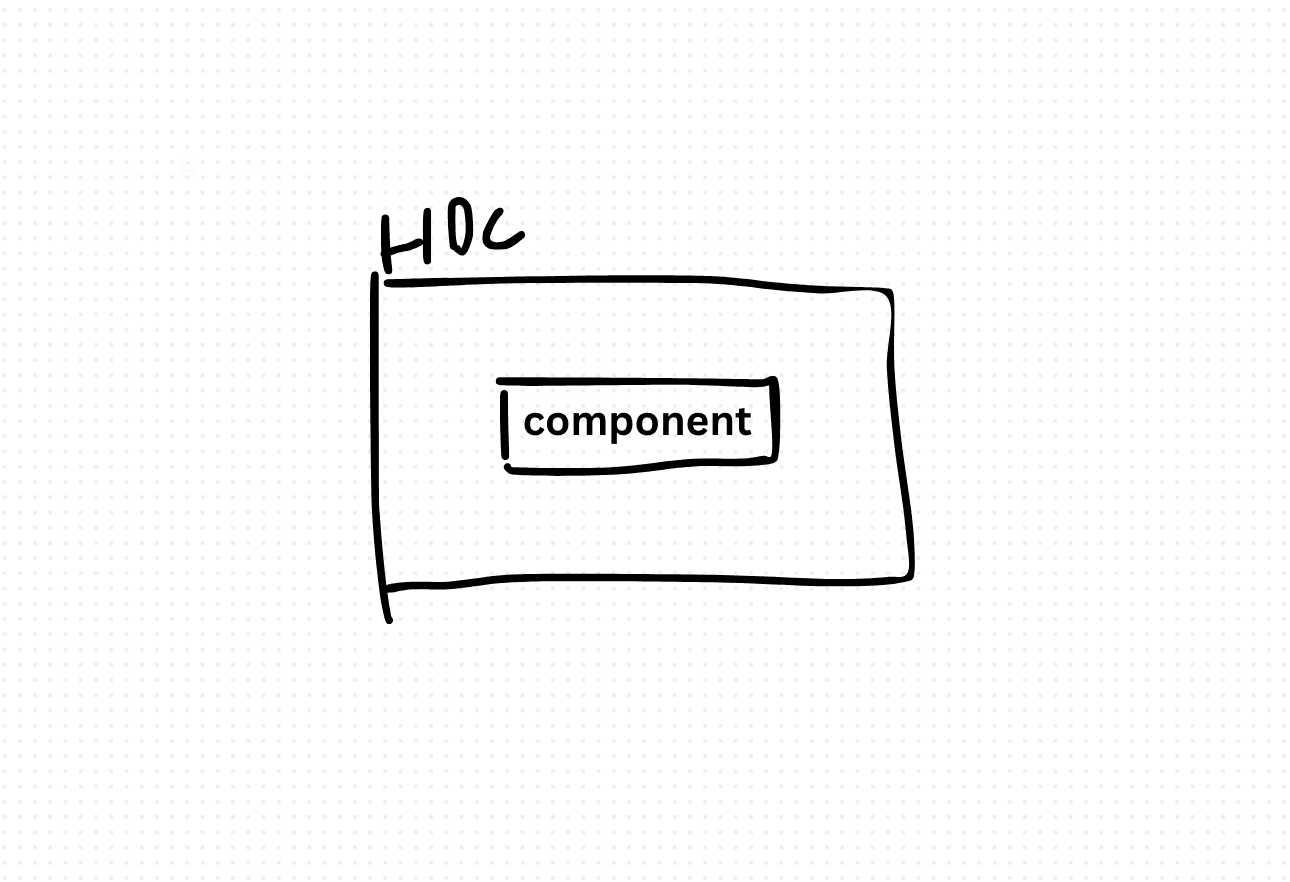
The Higher-Order Component (HOC) Pattern is like a magic wand for your React components. It's a function that takes a component as input and returns a new enhanced component with additional functionality. Think of it as giving your components a makeover without messing with their core essence.
The Secret Sauce:
So, what makes the HOC Pattern so special? It's all about enhancing your components with extra capabilities while keeping your codebase clean and modular. With HOCs, you can add features like logging, authentication, or data fetching to your components with ease.
Implementing the Higher-Order Component (HOC) Pattern:
Let's roll up our sleeves and see the HOC Pattern in action with a simple example:
import React, { useEffect } from 'react';
const withLogging = (WrappedComponent) => {
const EnhancedComponent = (props) => {
useEffect(() => {
console.log(`Component ${WrappedComponent.name || 'Anonymous'} mounted`);
}, []);
return <WrappedComponent {...props} />;
};
return EnhancedComponent;
};
export default withLogging;
In this example, withLogging is our HOC that adds logging functionality to any component it wraps. It logs a message when the wrapped component mounts, giving us insight into component lifecycle events.
import React from 'react';
import withLogging from './withLogging';
const MyComponent = ({ name }) => (
<div>
<h2>Hello, {name}!</h2>
</div>
);
export default withLogging(MyComponent);
Meanwhile, MyComponent is our humble component, unaware of its newfound logging capabilities. We enhance it with withLogging, and voila – instant logging magic!
- Reusability: HOCs are like Swiss Army knives for your components – versatile and reusable across your app.
- Separation of Concerns: HOCs keep your codebase tidy by separating cross-cutting concerns from your components.
- Flexibility: HOCs give you the flexibility to customize and compose components in various ways, empowering you to build complex UIs with ease.
Conclusion:
And there you have it – the Higher-Order Component (HOC) Pattern demystified! With HOCs in your toolkit, you'll wield the power to enhance your React apps with ease. So go ahead, sprinkle some HOC magic into your code, and watch your React apps shine brighter than ever!