Facade Design Pattern
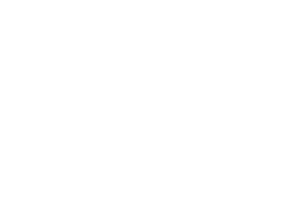
At its core, the Facade Design Pattern aims to simplify the usage of complex subsystems by providing a single, simplified interface. It acts as a "facade" or entry point to the subsystem, shielding clients from the details of individual components and providing a more intuitive and streamlined interface. This pattern promotes loose coupling between clients and subsystems, making it easier to maintain and extend the system over time.
// Subsystem 1
class VideoPlayer {
play(video) {
console.log(`Playing video: ${video}`);
}
pause() {
console.log("Pausing video");
}
}
// Subsystem 2
class SubtitleManager {
addSubtitle(subtitle) {
console.log(`Adding subtitle: ${subtitle}`);
}
}
// Facade
class MovieStreamingFacade {
constructor() {
this.videoPlayer = new VideoPlayer();
this.subtitleManager = new SubtitleManager();
}
watchMovie(movie, subtitle) {
console.log(`Streaming movie: ${movie}`);
this.videoPlayer.play(movie);
if (subtitle) {
this.subtitleManager.addSubtitle(subtitle);
}
}
pauseMovie() {
this.videoPlayer.pause();
}
}