Error Boundary React Design Pattern
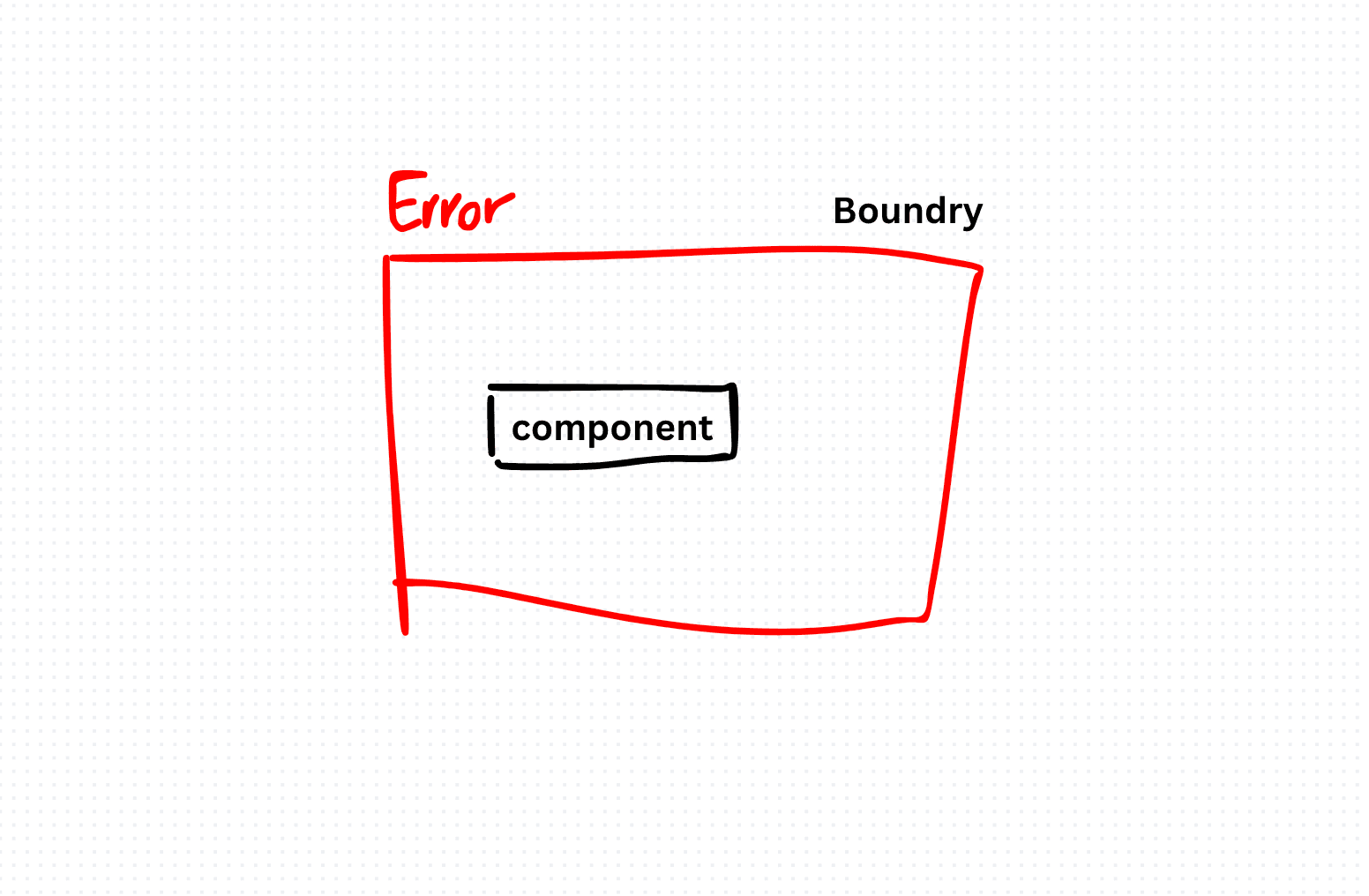
What makes the Error Boundary Design Pattern so powerful? It's all about gracefully handling errors and preventing them from disrupting the user experience. With Error Boundaries, you can isolate errors to specific parts of your UI, keeping the rest of your app functioning smoothly.
Implementing the Error Boundary Design Pattern:
Let's dive into a practical example to see the Error Boundary in action:
import React, { useState } from 'react';
const ErrorBoundary = ({ children }) => {
const [hasError, setHasError] = useState(false);
const handleOnError = () => {
setHasError(true);
};
if (hasError) {
return <h1>Oops! Something went wrong.</h1>;
}
return (
<div onError={handleOnError}>
{children}
</div>
);
};
export default ErrorBoundary;
In this example, we create an ErrorBoundary component using a functional component and the useState hook. It catches errors within its children and displays a fallback UI when an error occurs.
import React from 'react';
import ErrorBoundary from './ErrorBoundary';
import MyComponent from './MyComponent';
const App = () => (
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
);
export default App;
Meanwhile, in our App component, we wrap our MyComponent with the ErrorBoundary to handle any errors that may occur within it.
And there you have it – the Error Boundary Design Pattern demystified! With Error Boundaries in your toolkit, you can build more resilient React applications that gracefully handle errors and provide a smoother user experience. So go ahead, implement Error Boundaries in your projects, and elevate your React game to new heights! 🚀