Monitor Object Design Pattern
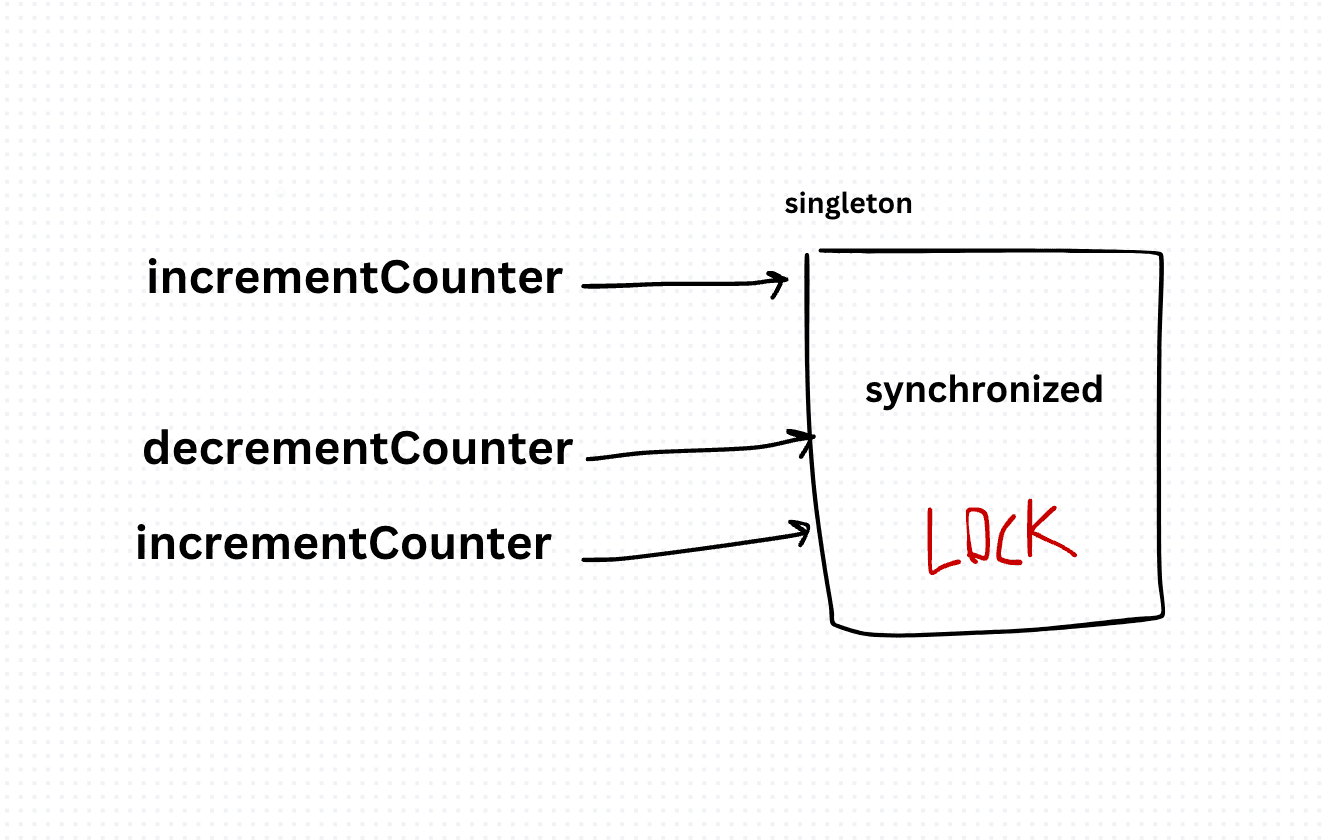
The Monitor Object Pattern is a synchronization pattern used to control access to shared resources in a multi-threaded environment. It encapsulates the shared resource along with synchronization mechanisms to ensure that only one thread can access the resource at a time. The monitor object acts as a gatekeeper, preventing concurrent access and maintaining consistency and integrity of shared data.
class Monitor {
constructor() {
this.counter = 0;
this.lock = false;
}
async synchronized(func) {
while (this.lock) {
await new Promise(resolve => setTimeout(resolve, 10)); // Wait until the lock is released
}
this.lock = true; // Acquire the lock
try {
await func();
} finally {
this.lock = false; // Release the lock
}
}
async increment() {
await this.synchronized(async () => {
this.counter++;
console.log("Counter incremented:", this.counter);
});
}
async decrement() {
await this.synchronized(async () => {
this.counter--;
console.log("Counter decremented:", this.counter);
});
}
getCount() {
return this.counter;
}
}
const monitor = new Monitor();
// Example usage
async function incrementCounter() {
await monitor.increment();
}
async function decrementCounter() {
await monitor.decrement();
}
incrementCounter();
decrementCounter();
incrementCounter();
incrementCounter();
The Monitor Object Pattern provides an effective solution for managing shared resources and synchronizing access in JavaScript applications. By encapsulating shared resources within monitor objects and providing synchronized methods for accessing them, this pattern ensures thread safety and prevents concurrency issues such as race conditions. In JavaScript, the Monitor Object pattern can be implemented using asynchronous programming techniques and a simple lock mechanism.