Command Query Responsibility Segregation (CQRS) Design Pattern
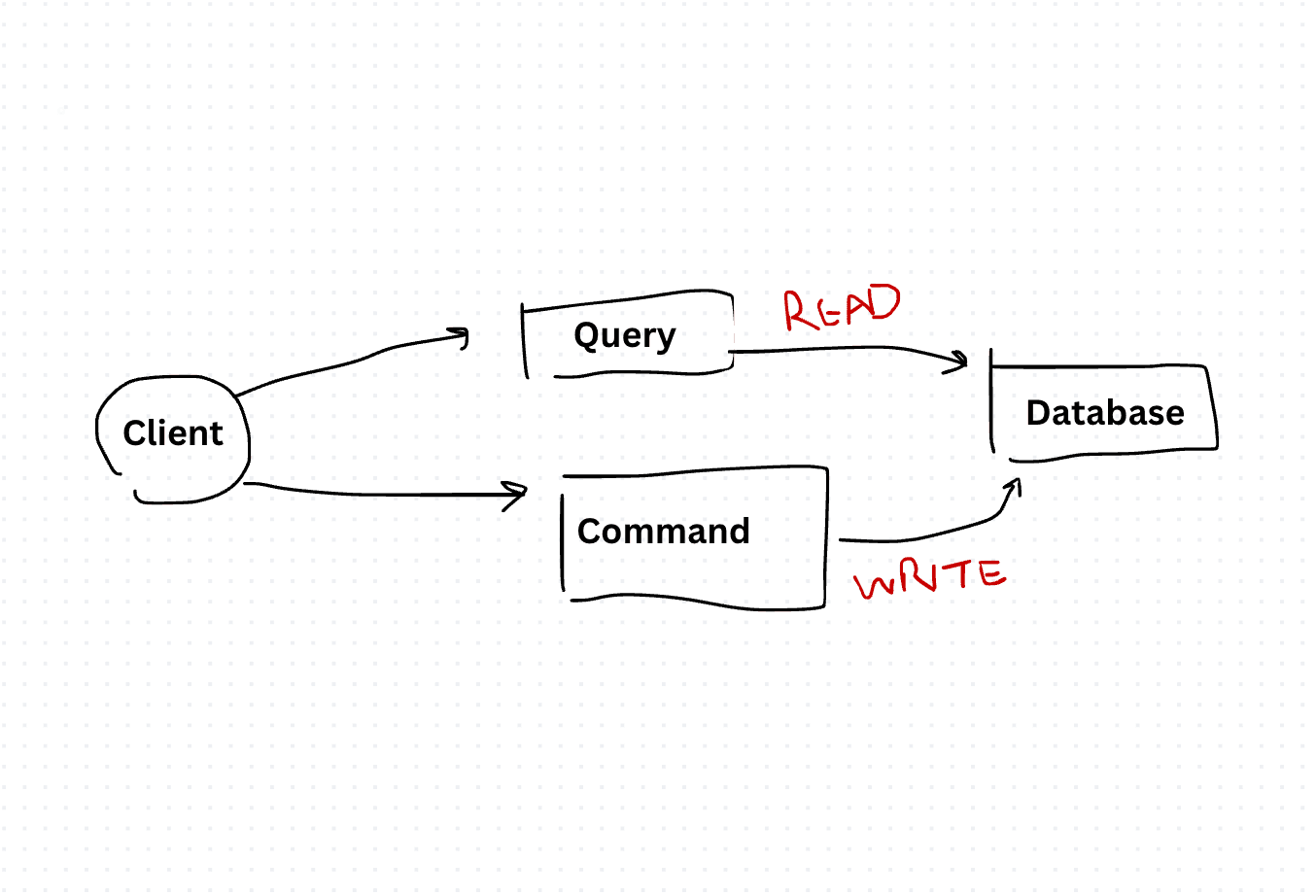
CQRS separates the responsibility of handling commands (write operations) from that of handling queries (read operations) in a software application. By segregating these concerns, CQRS allows for independent optimization and scaling of each side of the application.
- Commands: Represent actions that change the state of the application.
- Queries: Represent read operations that retrieve data from the application's state.
- Command Model: Handles commands and updates the application's state.
- Query Model: Optimized for efficient data retrieval and querying.
Simple Task:
class TaskCommandService {
constructor() {
this.tasks = [];
}
createTask(task) {
this.tasks.push(task);
}
updateTask(id, updatedTask) {
const index = this.tasks.findIndex(task => task.id === id);
if (index !== -1) {
this.tasks[index] = { ...this.tasks[index], ...updatedTask };
}
}
}
// Query Model
class TaskQueryService {
constructor(taskCommandService) {
this.taskCommandService = taskCommandService;
}
getTasks() {
return this.taskCommandService.tasks;
}
getTaskById(id) {
return this.taskCommandService.tasks.find(task => task.id === id);
}
}
// Usage
const taskCommandService = new TaskCommandService();
const taskQueryService = new TaskQueryService(taskCommandService);
// Create a new task
taskCommandService.createTask({ id: 1, title: 'Task 1', description: 'Description 1' });
// Update an existing task
taskCommandService.updateTask(1, { title: 'Updated Task 1' });
// Query tasks
const tasks = taskQueryService.getTasks();
console.log(tasks);
// Query a task by ID
const taskById = taskQueryService.getTaskById(1);
console.log(taskById);
Implementing CQRS in Practice:
Implementing CQRS requires careful consideration of the application's domain and requirements. This typically involves:
- Defining clear boundaries between commands and queries.
- Designing separate models and components for handling commands and queries.
- Implementing mechanisms for asynchronous communication and eventual consistency between the command and query sides.
- Testing and validating the behavior of the system under different scenarios, including concurrent access and failure conditions.
Command Query Responsibility Segregation (CQRS) is a powerful design pattern that offers numerous benefits for building complex and scalable software applications. By separating the concerns of handling commands and queries, CQRS enables developers to optimize each side independently, resulting in improved performance, flexibility, and maintainability. While implementing CQRS requires careful planning and consideration, the benefits it offers can greatly enhance the quality and scalability of modern software systems.