Cookie Limitations in TypeScript
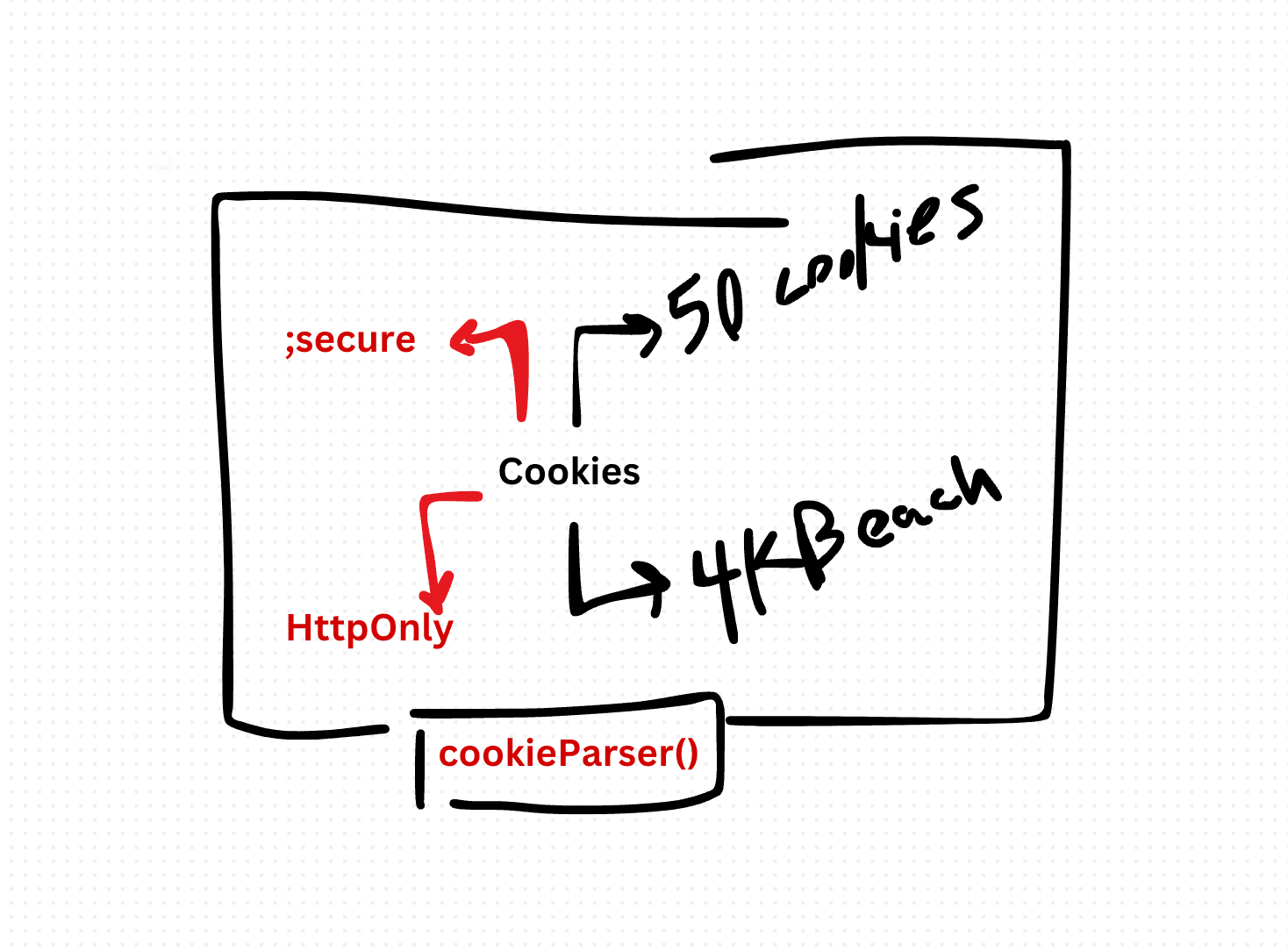
One of the primary limitations of cookies is their limited storage capacity. Browsers typically impose a maximum cookie size limit, which varies between browsers but is commonly around 4 KB per cookie and 50 cookies per domain. TypeScript developers must be mindful of these constraints when storing data in cookies:
document.cookie = 'exampleCookie=' + encodeURIComponent('Lorem ipsum dolor sit amet');
Cookies can only store data as strings, which means that complex data structures like objects or arrays must be serialized into strings before being stored. TypeScript developers can achieve this using methods such as JSON.stringify():
const complexData = { key: 'value', array: [1, 2, 3] };
document.cookie = 'complexData=' + encodeURIComponent(JSON.stringify(complexData));
Cookies are susceptible to various security vulnerabilities, including cross-site scripting (XSS) attacks and cross-site request forgery (CSRF) attacks. TypeScript developers must implement appropriate security measures to mitigate these risks, such as using secure and HTTP-only cookies:
document.cookie = 'secureCookie=value; secure';
document.cookie = 'httpOnlyCookie=value; HttpOnly';
Cookies are accessible to both the client-side and server-side code, making them vulnerable to tampering or exploitation. TypeScript developers should avoid storing sensitive information in cookies and implement server-side validation and verification mechanisms:
// Server-side code example (Node.js)
const cookieParser = require('cookie-parser');
app.use(cookieParser());
app.get('/', (req, res) => {
const cookieValue = req.cookies.exampleCookie;
// Perform validation and verification
});
Cookies remain a valuable tool for storing small amounts of data on the client-side, including in TypeScript applications. However, developers must be aware of their limitations and take appropriate precautions to mitigate potential risks. By understanding and addressing these limitations, TypeScript developers can leverage cookies effectively while ensuring the security and integrity of their applications.