Meaningful Test Concepts: Best Practices for Writing Tests in TypeScript
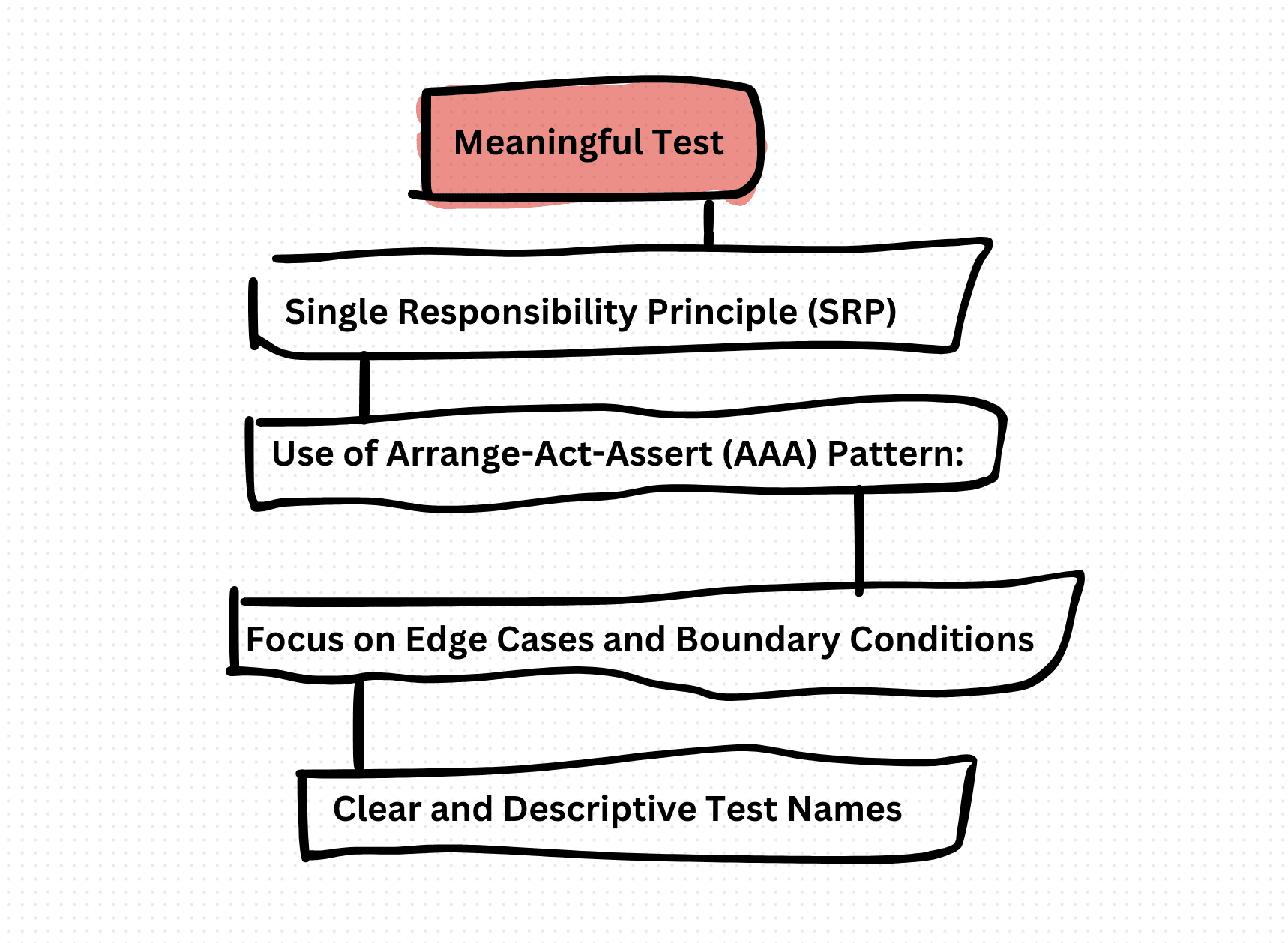
When writing tests, it's crucial to ensure that each test case has a clear and meaningful concept, focusing on a specific behavior or scenario of the code under test. Meaningful test concepts improve test readability, maintainability, and effectiveness, making it easier to diagnose failures and understand the intent of the test suite. This article explores best practices for crafting meaningful test concepts in TypeScript and provides examples to illustrate these principles.
- Single Responsibility Principle (SRP) for Tests:
Just as code adheres to the SRP, each test should focus on a single logical concept or behavior. Avoid combining multiple assertions or scenarios within a single test case, as it can make the test less readable and harder to diagnose when failures occur.
describe('Calculator', () => {
it('should add two numbers correctly', () => {
const calculator = new Calculator();
const result = calculator.add(2, 3);
expect(result).toBe(5);
});
});
- Clear and Descriptive Test Names:
Use descriptive test names that clearly communicate the intent and behavior being tested. A well-named test serves as documentation and helps developers understand the purpose of the test without diving into the implementation details.
describe('Calculator', () => {
it('should correctly add two positive numbers', () => {
});
it('should return zero when adding zero to any number', () => {
});
});
- Focus on Edge Cases and Boundary Conditions:
Ensure that tests cover edge cases, boundary conditions, and corner cases relevant to the functionality being tested. These tests help uncover potential bugs and ensure that the code behaves correctly under various scenarios.Example:
describe('ArrayUtils', () => {
it('should correctly handle an empty array', () => {
const result = ArrayUtils.getLastElement([]);
expect(result).toBeUndefined();
});
it('should return the last element of the array', () => {
const array = [1, 2, 3, 4, 5];
const result = ArrayUtils.getLastElement(array);
expect(result).toBe(5);
});
});
- Use of Arrange-Act-Assert (AAA) Pattern:
Structure tests using the AAA pattern, where each test case consists of three distinct sections: arranging the preconditions, acting on the code under test, and asserting the expected outcomes. This pattern enhances test readability and clarity.
describe('UserService', () => {
it('should retrieve a user by ID from the database', () => {
// Arrange
const userId = '123';
const databaseMock = createDatabaseMock();
// Act
const user = UserService.getUserById(userId, databaseMock);
// Assert
expect(user.id).toBe(userId);
});
});
By following best practices for crafting meaningful test concepts in TypeScript, developers can create test suites that are easy to understand, maintain, and extend. By focusing on single responsibilities, using clear and descriptive test names, covering edge cases, and adhering to the AAA pattern, tests become powerful tools for ensuring code quality and reliability.