Reducing Input Variables in TypeScript Functions
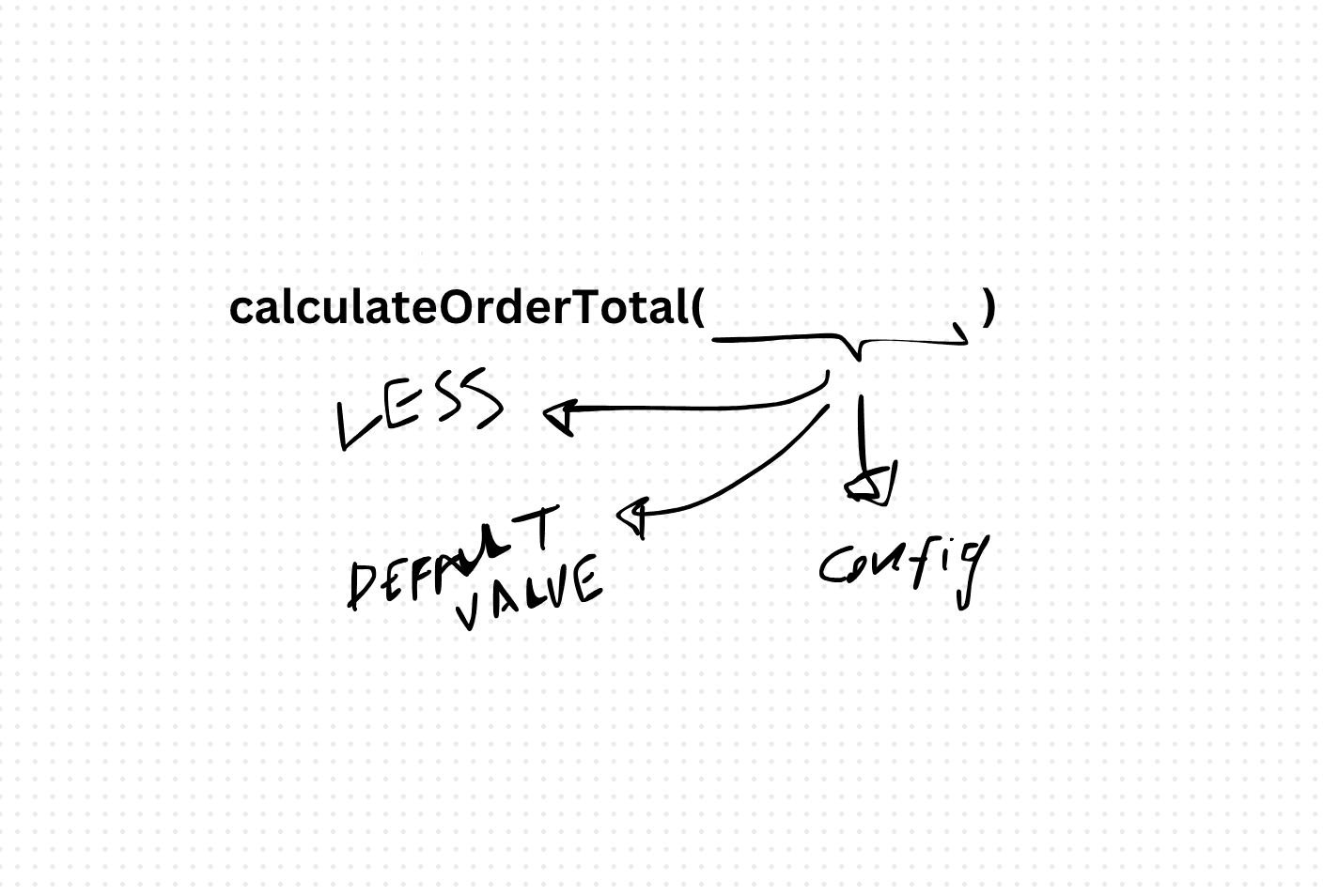
In TypeScript development, functions with a high number of input variables can become cumbersome to manage and maintain. Excessive input variables often indicate low cohesion, making functions less reusable and harder to test. In this article, we'll explore techniques for reducing the number of input variables in functions, improving cohesion, and enhancing code readability. With practical examples in TypeScript, we'll demonstrate how to refactor functions to maximize cohesion and minimize complexity.
Strategies:
- Group Related Data: If multiple input variables are closely related, consider grouping them into a single object or data structure. This approach encapsulates related data and reduces the number of parameters passed to the function.
function calculateOrderTotal(price: number, quantity: number, discount: number): number {
}
// After Refactoring
type OrderDetails = {
price: number;
quantity: number;
discount: number;
};
function calculateOrderTotal(order: OrderDetails): number {}
- Use Default Parameters: Use default parameter values to provide sensible defaults for optional inputs. This allows callers to omit unnecessary parameters, reducing the number of arguments required.
function greet(name: string, greeting: string): void {
console.log(`${greeting}, ${name}!`);
}
// After Refactoring
function greet(name: string = "Sir/Madam", greeting: string = 'Hello'): void {
console.log(`${greeting}, ${name}!`);
}
- Introduce Configuration Objects: Instead of passing individual parameters, consider passing a configuration object or options parameter containing relevant settings. This consolidates related inputs and simplifies function calls
function fetchData(url: string, method: string, headers: Record<string, string>, timeout: number): Promise<any> {
}
// After Refactoring
type FetchOptions = {
url: string;
method: string;
headers: Record<string, string>;
timeout: number;
};
function fetchData(options: FetchOptions): Promise<any> {}
By minimizing the number of input variables in functions, developers can enhance cohesion, improve code maintainability, and promote reusability. Through refactoring techniques such as grouping related data, using default parameters, and introducing configuration objects, functions can become more focused, concise, and easier to use. With these strategies and examples in TypeScript, developers can write cleaner, more cohesive codebases that are easier to understand and maintain over time.