Avoiding Negative Conditions: Best Practices with TypeScript
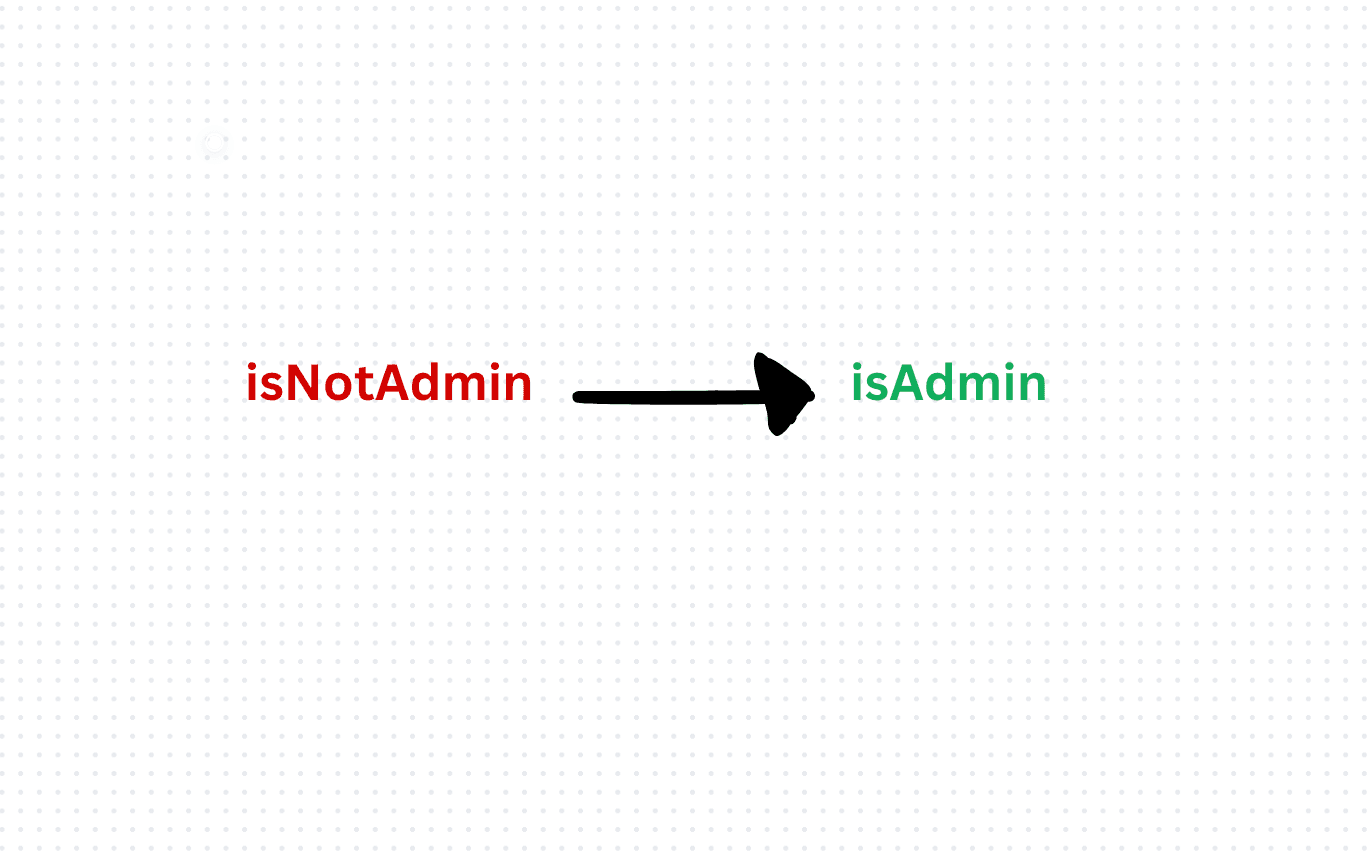
In TypeScript development, writing clear, readable, and maintainable code is essential for effective collaboration and long-term maintainability. One common pitfall that can hinder code readability is the use of negative conditions, which can make code harder to understand and reason about. In this article, we'll explore best practices for avoiding negative conditions in TypeScript code, along with examples to illustrate these concepts.
Negative conditions refer to conditional statements or expressions that check for the absence or negation of a certain condition. These conditions typically involve negating boolean expressions using keywords such as not, !, or !==. While negative conditions can be valid in some scenarios, they often introduce cognitive overhead and make code more challenging to comprehend.
// Negative Condition
function isNotAdmin(user: User): boolean {
return user.role !== 'admin';
}
// Positive Condition
function isAdmin(user: User): boolean {
return user.role === 'admin';
}
Avoiding negative conditions in TypeScript code promotes clarity, readability, and maintainability. By favoring positive conditionals, using descriptive variable names, refactoring complex conditions, and considering early returns, developers can write more understandable and maintainable codebases. Embracing these best practices contributes to better code quality and enhances the overall developer experience.