Task Scheduling with AgendaJS in Node.js
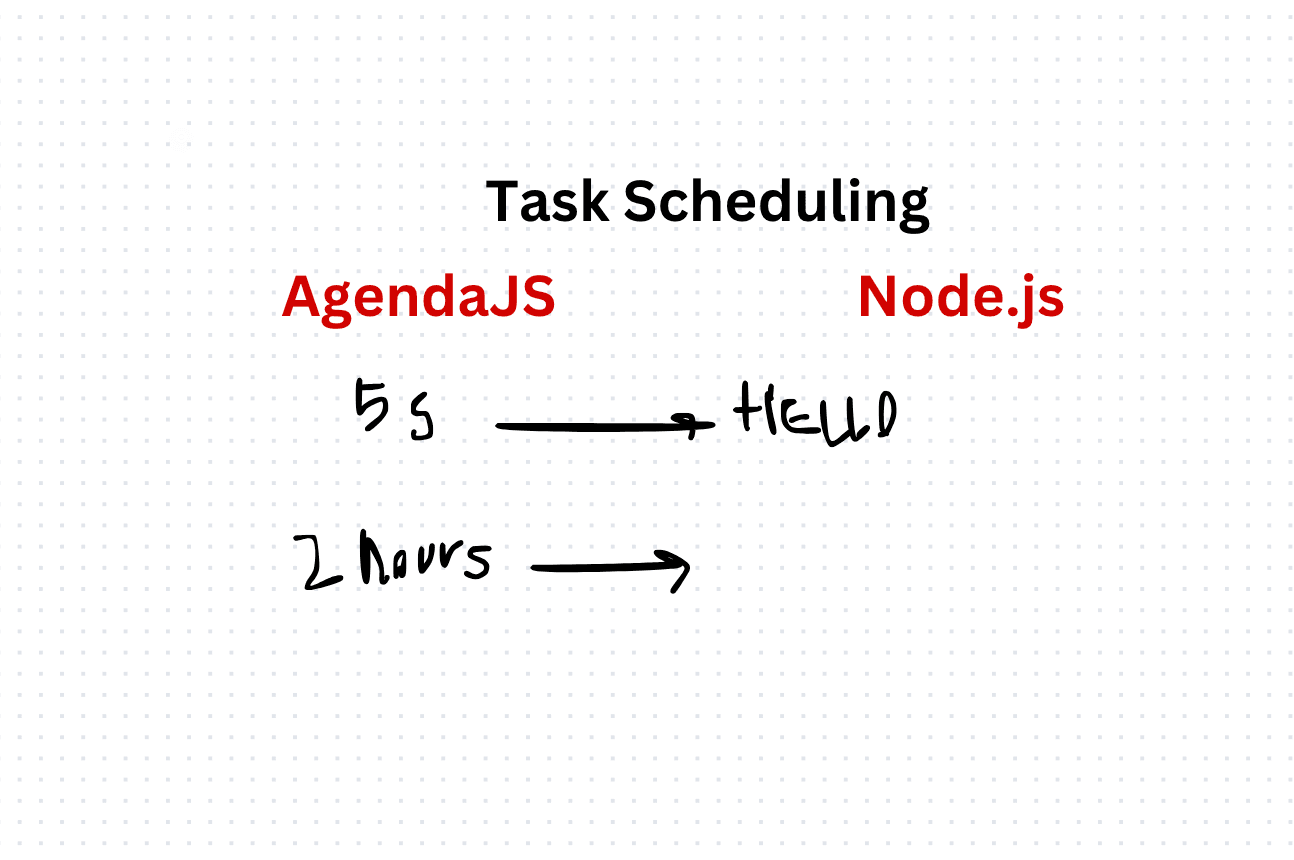
Task scheduling is a critical aspect of many Node.js applications, allowing developers to automate repetitive tasks, process background jobs, and manage asynchronous operations effectively. In this comprehensive guide, we'll explore how to implement task scheduling in Node.js using AgendaJS, a powerful job scheduling library built on top of MongoDB. We'll cover the installation process, basic usage, advanced features, and best practices for integrating AgendaJS into your Node.js projects.
import { MongoClient } from "mongodb";
import { Agenda } from "agenda";
const mongoURI = "mongodb+srv://agendajs:ua59A40I4KbrGcng@agendajs.chfsrry.mongodb.net/?retryWrites=true&w=majority&appName=agendajs";
const agenda = new Agenda({
db: {
address: mongoURI,
collection: `agendaJobs-${Math.random()}`,
},
});
const time = () => {
const date = new Date();
return `${date.getHours()}:${date.getMinutes()}:${date.getSeconds()}`;
};
agenda.define("hello", async (job, done) => {
console.log(time(), "Hello, World!");
done();
});
const server = async () => {
agenda.processEvery("1 second");
await agenda.start();
agenda.every("10 second", "hello");
agenda.on("start", (job) => {
console.log(time(), `Job <${job.attrs.name}> starting`);
});
agenda.on("success", (job) => {
console.log(time(), `Job <${job.attrs.name}> succeeded`);
});
agenda.on("fail", (error, job) => {
console.log(time(), `Job <${job.attrs.name}> failed:`, error);
});
};
server();
And To Test it write this
import server from "./server";
import { Agenda } from "agenda";
jest.mock("mongodb", () => {
const MongoClient = {
connect: jest.fn(),
};
return { MongoClient };
});
jest.mock("agenda");
describe("server", () => {
it("should call agenda.define", async () => {
await server();
expect(Agenda).toHaveBeenCalled();
expect(Agenda).toHaveBeenCalledWith({
db: {
address: expect.any(String),
collection: expect.any(String),
},
});
expect(Agenda.prototype.define).toHaveBeenCalled();
expect(Agenda.prototype.define).toHaveBeenCalledWith(
"hello",
expect.any(Function)
);
});
it('should call agenda.processEvery("1 second")', async () => {
await server();
expect(Agenda.prototype.processEvery).toHaveBeenCalled();
expect(Agenda.prototype.processEvery).toHaveBeenCalledWith("1 second");
});
it("should call agenda.start", async () => {
await server();
expect(Agenda.prototype.start).toHaveBeenCalled();
});
it('should call agenda.every("10 second", "hello")', async () => {
await server();
expect(Agenda.prototype.every).toHaveBeenCalled();
expect(Agenda.prototype.every).toHaveBeenCalledWith("10 second", "hello");
});
});
Node.js Task Scheduling AgendaJS MongoDB Job Scheduling Background Jobs Asynchronous Operations Automation Best Practices