OpenAI APIs: From ChatGPT to Image Generatio
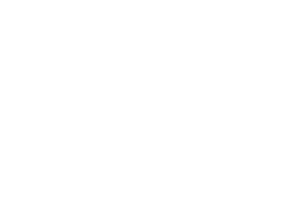
OpenAI's APIs have been a topic of much discussion, leaving me curious about the opportunities they present, their functionalities, and associated costs. Upon delving into their documentation, I found the initial setup to be straightforward. To begin, you can install the OpenAI package with the following npm commands:
npm init
npm install openai --save
Subsequently, a simple function like the one below can kickstart your exploration:
import OpenAI from "openai";
const openai = new OpenAI();
async function chat() {
const completion = await openai.chat.completions.create({
messages: [{ role: "system", content: "You are a helpful assistant." }],
model: "gpt-3.5-turbo",
});
console.log(completion.choices[0]);
}
chat();
However, acquiring an API key is essential. After obtaining one from the OpenAI platform (https://platform.openai.com/api-keys) and storing it in my ~/.zshrc file:
export OPENAI_API_KEY='VALUE'
encountered a new challenge when running node opeai-test.js in a different terminal, receiving an error code 429, indicating an exceeded quota. It turns out that using ChatGPT for over three months required me to add credit to my usage (https://platform.openai.com/usage), a process distinct from purchasing ChatGPT 4. Therefore, it's advisable not to commit to the $20 per month plan if your sole interest lies in using the APIs.
Despite this hiccup, running the code with a valid key yielded a response from ChatGPT. Notably, constructing an array of messages, such as:
messages: [{ role: "system", content: "You are a helpful assistant." }],
proved crucial for maintaining context in back-and-forth interactions. Handling thousands of related messages might pose a challenge.
Upon further investigation, I realized I wasn't using the specified model (gpt-3.5-turbo), prompting contemplation on the myriad possibilities the tool could offer. While ChatGPT's web interface (chat.openai.com) is user-friendly, envisioning it as a product might require a model trained on your specific data rather than relying solely on internet-sourced information.
The platform introduces additional features like embeddings, with a suggestion to explore the Assistant APIs currently in beta.
Another noteworthy feature is image generation. If you haven't experienced products like ArtFlow AI, I recommend giving it a try for its exquisite image generation capabilities.
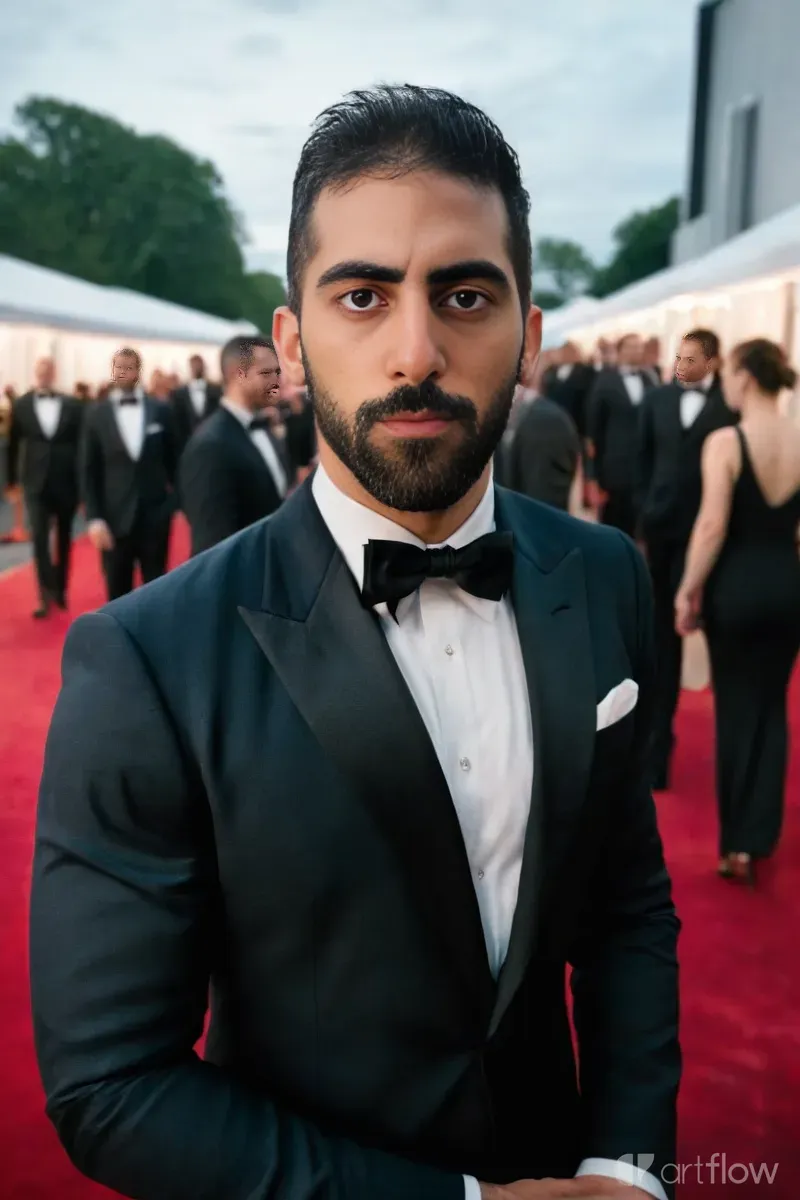
The following code snippet demonstrates using the endpoint for image generation:
async function generateImage() {
const image = await openai.images.generate({
model: "dall-e-3",
prompt: "A cute baby sea otter",
});
console.log(image.data);
}
generateImage();
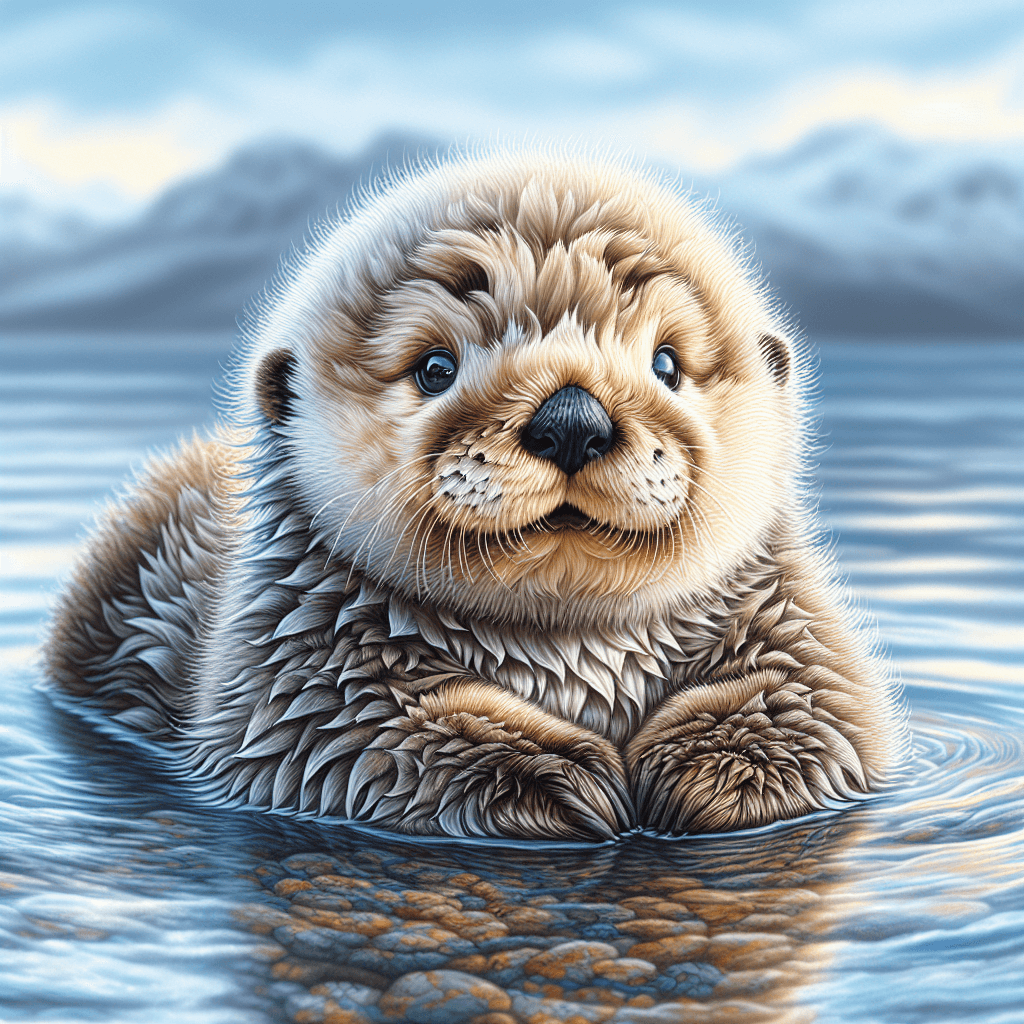
Remarkably easy! Now, turning to pricing, a single API call for chat and image generation costs 0.04 dollars each, with the latter being slightly more expensive. Notably, every 100 image generations incur a cost of 4 dollars, making it a bit on the pricier side.
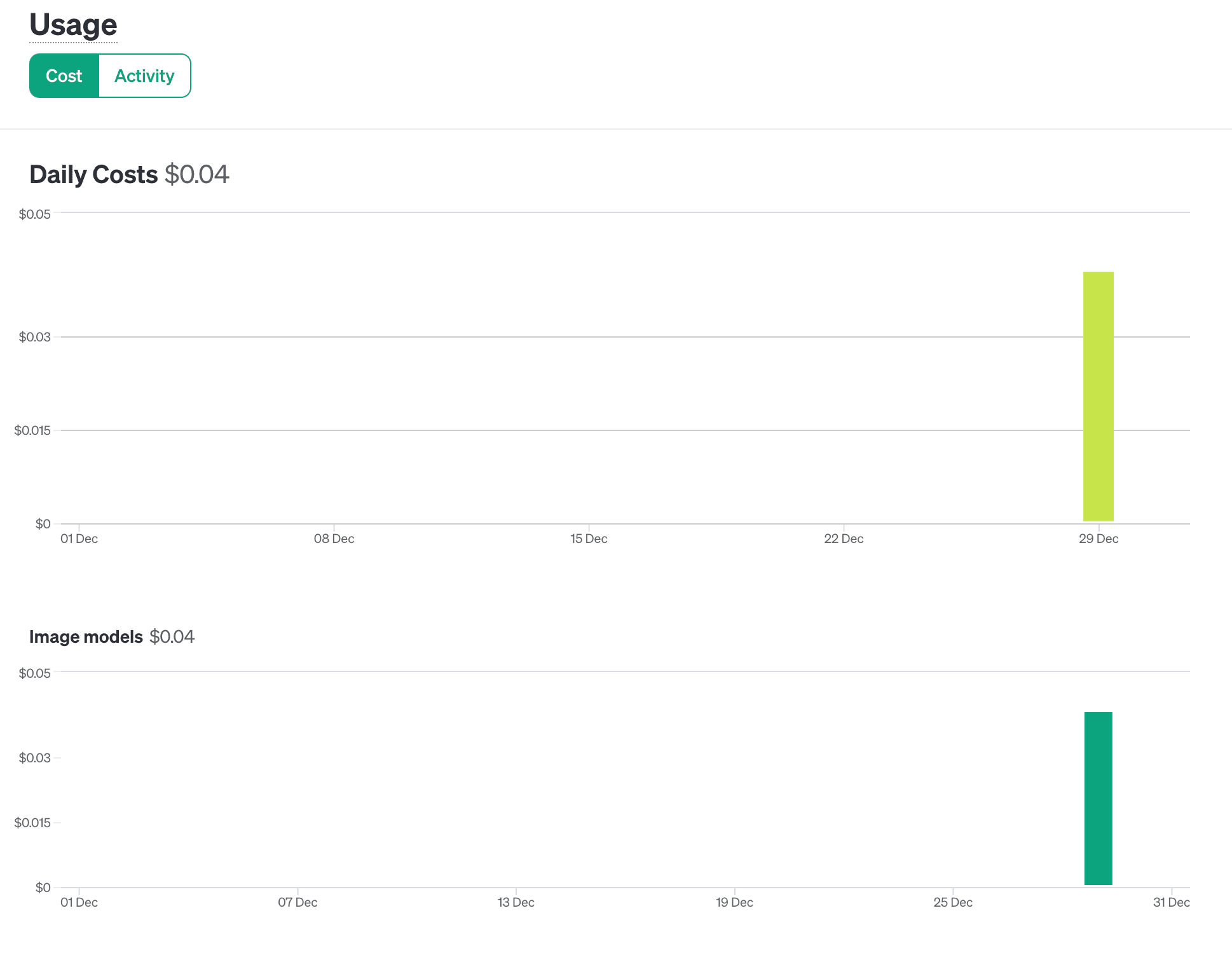
In addition to these, OpenAI offers GPT-4 Vision, where you can link or upload an image for ChatGPT to answer questions, along with Text to Speech and Speech to Text functionalities."
In summary, OpenAI's APIs offer developers an accessible pathway to integrate advanced language models into their applications. The setup is straightforward, involving the installation of the OpenAI package and obtaining an API key. The provided code snippets demonstrate easy interaction with the Chat API and image generation.
Despite minor challenges like usage quotas, the Chat API's versatility in constructing conversation arrays allows nuanced interactions. The platform introduces features like embeddings and the beta Assistant API, broadening its application scope. Image generation showcases creative potential, with considerations for associated costs.
Looking ahead, envisioning ChatGPT as a product emphasizes the importance of using a model trained on custom data. The addition of GPT-4 Vision, Text to Speech, and Speech to Text functionalities further enhances OpenAI's offerings.
In essence, OpenAI's APIs empower developers to infuse cutting-edge natural language processing capabilities into their projects, promising continued innovation in AI-driven application development.