How do you implement a React infinite scroll in a React application?
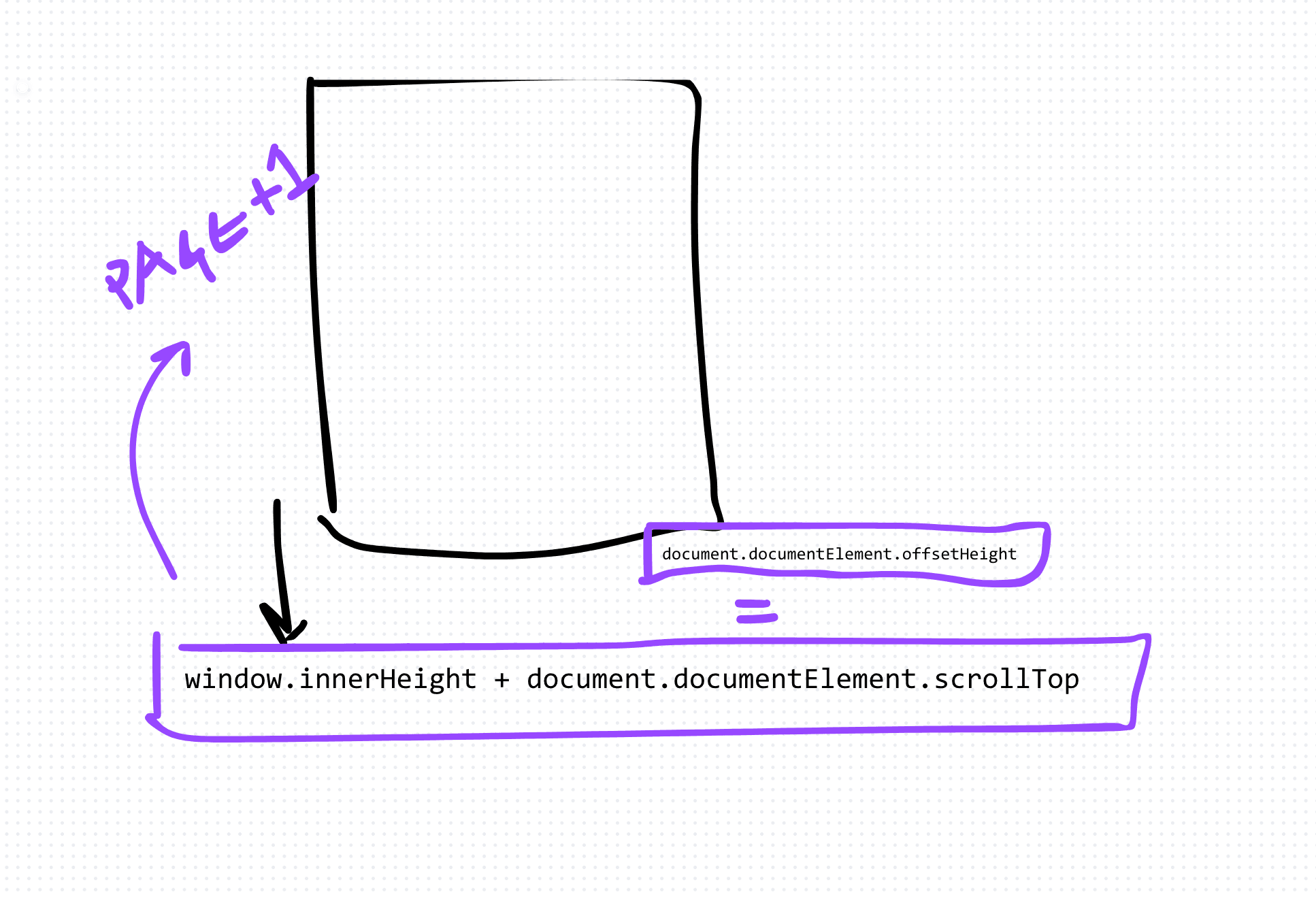
In TypeScript React applications, implementing infinite scroll functionality enhances user experience by dynamically loading content as the user scrolls down the page. React provides a straightforward way to achieve infinite scroll behavior using event listeners and state management. Implementing a React infinite scroll ensures smoother navigation and faster loading times, making it a valuable feature for applications with large datasets. Let's explore how to implement a React infinite scroll with examples to illustrate its usage and benefits.
React infinite scroll is a technique used to load content dynamically as the user scrolls down the page. Instead of loading all content upfront, infinite scroll fetches additional data as needed, providing a seamless browsing experience. This approach is particularly useful for applications with large datasets or paginated content.
import React, { useState, useEffect } from 'react';
const App: React.FC = () => {
const [data, setData] = useState<number[]>([]);
const [page, setPage] = useState(1);
useEffect(() => {
fetchData();
}, [page]);
const fetchData = async () => {
const newData = await fetch(`/api/data?page=${page}`);
const jsonData = await newData.json();
setData((prevData) => [...prevData, ...jsonData]);
};
const handleScroll = () => {
if (
window.innerHeight + document.documentElement.scrollTop ===
document.documentElement.offsetHeight
) {
setPage((prevPage) => prevPage + 1);
}
};
useEffect(() => {
window.addEventListener('scroll', handleScroll);
return () => window.removeEventListener('scroll', handleScroll);
}, []);
return (
<div>
{data.map((item, index) => (
<div key={index}>{item}</div>
))}
</div>
);
};
export default App;
In TypeScript React applications, implementing infinite scroll functionality provides a smoother and more efficient way to navigate through large datasets or paginated content. By dynamically loading data as the user scrolls, infinite scroll enhances user experience and reduces loading times. Understanding and implementing React infinite scroll effectively enables developers to create more responsive and user-friendly applications.