React Focus Trap in a TypeScript React Application
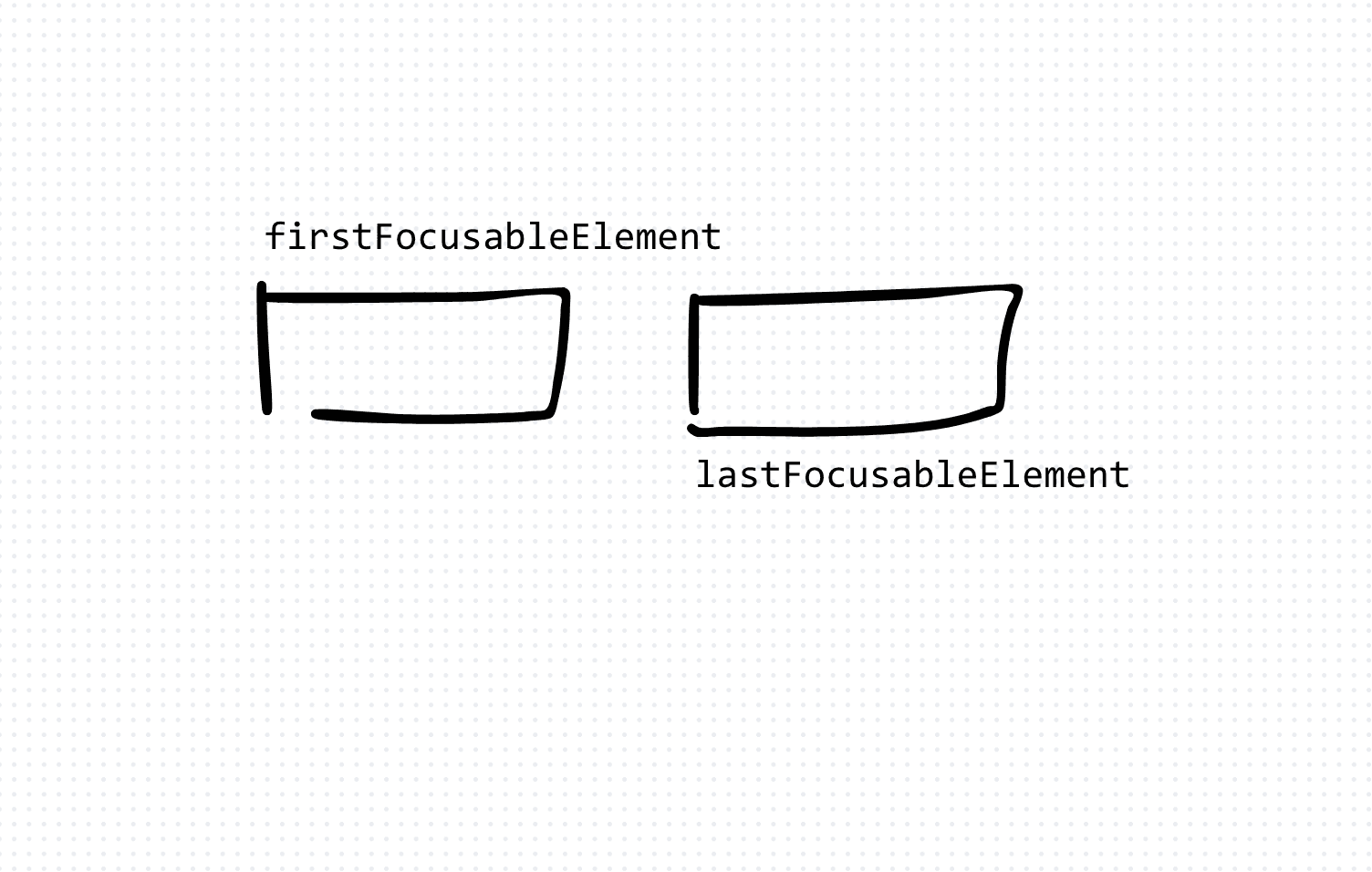
React focus trap is a technique used to confine keyboard focus within a specific area of the DOM, typically within a modal or popover. By trapping focus, it prevents users from accidentally tabbing out of the interactive element, improving accessibility and usability.
import React, { useEffect, useRef } from 'react';
const FocusTrap: React.FC = ({ children }) => {
const firstFocusableElement = useRef<HTMLButtonElement>(null);
const lastFocusableElement = useRef<HTMLButtonElement>(null);
useEffect(() => {
const handleTabKey = (event: KeyboardEvent) => {
if (event.key === 'Tab') {
if (document.activeElement === lastFocusableElement.current) {
event.preventDefault();
firstFocusableElement.current?.focus();
} else if (document.activeElement === firstFocusableElement.current && event.shiftKey) {
event.preventDefault();
lastFocusableElement.current?.focus();
}
}
};
document.addEventListener('keydown', handleTabKey);
return () => {
document.removeEventListener('keydown', handleTabKey);
};
}, []);
return (
<>
<button ref={firstFocusableElement}>First Focusable Element</button>
{children}
<button ref={lastFocusableElement}>Last Focusable Element</button>
</>
);
};
export default FocusTrap;
In TypeScript React applications, implementing a focus trap ensures that keyboard users can navigate through interactive elements within a defined region without getting lost or trapped. By confining focus within modal dialogs, popovers, and other interactive elements, focus traps enhance accessibility and improve the user experience for all users.
React TypeScript JavaScript Frontend Development Web Development Focus Trap Accessibility Keyboard Navigation Modal Popover