Sample Next.js application using Terraform in AWS
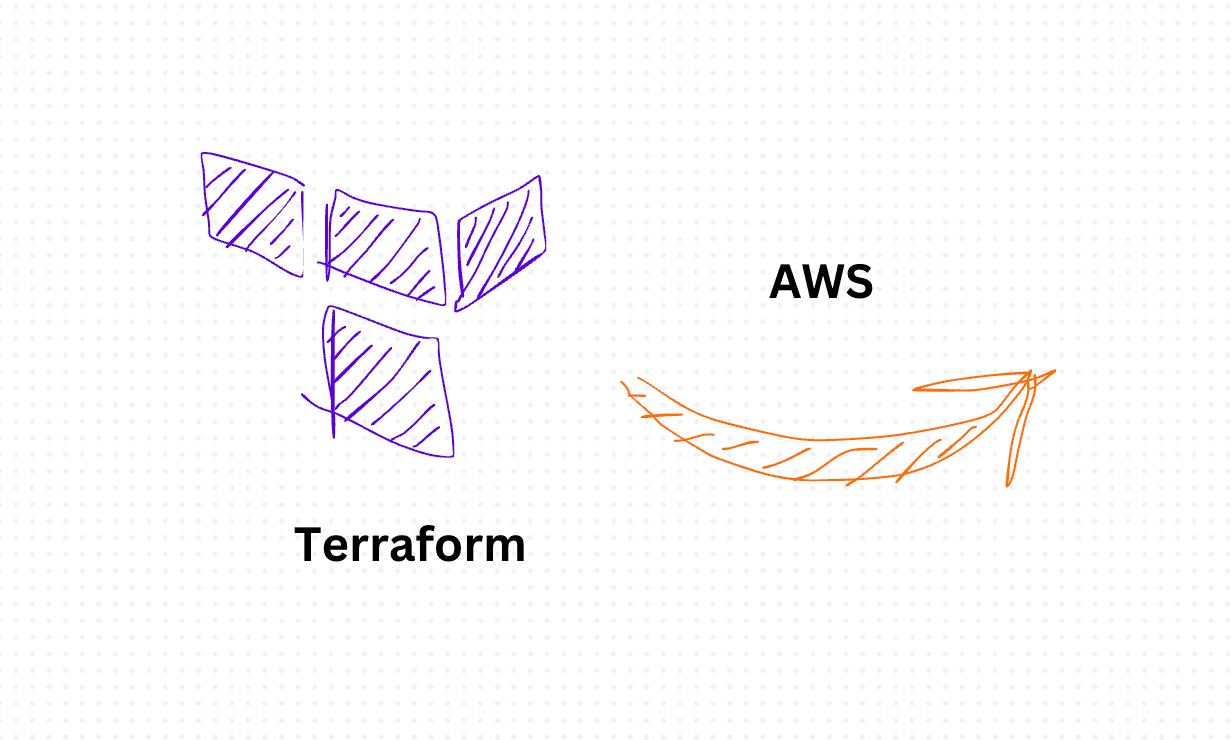
To deploy a sample Next.js application using Terraform in AWS, we'll go through the following steps:
- Set up an AWS account and configure AWS CLI.
- Write Terraform configuration to provision necessary resources.
- Deploy the Next.js application to an EC2 instance.
- Test the deployment.
Step 1: Set Up AWS Account and Configure AWS CLI
- Create an AWS account if you don't have one already.
- Install and configure AWS CLI on your local machine with your AWS credentials.
Step 2: Terraform
1. Install Terraform:
You can install Terraform on your local machine by following these steps:
Visit the Terraform download page.
Download the appropriate package for your operating system (e.g., Windows, macOS, Linux).
Extract the downloaded package and place the terraform binary in a directory included in your system's PATH.
Verify the installation by running terraform version in your terminal or command prompt. You should see the installed Terraform version displayed.
2. Configure AWS Credentials:
Before you can deploy infrastructure with Terraform on AWS, you need to configure your AWS credentials.
If you haven't done so already, create an IAM user in your AWS account with programmatic access and appropriate permissions for the resources you intend to provision. Make note of the Access Key ID and Secret Access Key for this IAM user.
Configure AWS CLI on your local machine by running aws configure in your terminal or command prompt. Enter the Access Key ID, Secret Access Key, preferred region, and default output format when prompted.
3. Write Terraform Configuration:
Create a directory for your Terraform configuration and create the following files:
- main.tf: Contains Terraform configuration for provisioning AWS resources.
# Define AWS provider
provider "aws" {
region = "us-east-1" # Choose your desired region
}
# Define EC2 instance
resource "aws_instance" "nextjs_instance" {
ami = "ami-xxxxxxxxx" # Replace with your desired AMI ID
instance_type = "t2.micro" # Choose your desired instance type
tags = {
Name = "Next.js Instance"
}
}
# Open port 80 for HTTP traffic
resource "aws_security_group" "http_sg" {
name = "http_sg"
description = "Allow HTTP inbound traffic"
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
}
# Associate security group with the EC2 instance
resource "aws_instance" "nextjs_instance" {
security_groups = [aws_security_group.http_sg.name]
}
Run the command `terraform init`. This initializes the directory and downloads any necessary providers and modules specified in your configuration.
4. Deploy:
Once Terraform is initialized and authenticated with AWS, you can deploy your infrastructure by running `terraform apply`
Step 3: Deploy Next.js Application to EC2 Instance with Docker
1. Dockerize the Next.js Application:
Create a Dockerfile in your Next.js application directory with the following content:
# Use official Node.js image as base
FROM node:latest
# Set working directory
WORKDIR /app
# Copy package.json and package-lock.json to the working directory
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy remaining application files to the working directory
COPY . .
# Build Next.js application
RUN npm run build
# Expose port 3000
EXPOSE 3000
# Command to run the application
CMD ["npm", "start"]
2. Build Docker Image:
Build the Docker image by running the following command in the directory containing your Dockerfile and application code:
docker build -t nextjs-app .
3. Push Docker Image to Docker Hub
docker tag nextjs-app your-docker-username/nextjs-app:latest
docker push your-docker-username/nextjs-app:latest
4. SSH into EC2 Instance:
ssh -i /path/to/your/key.pem ec2-user@ec2-instance-ip
5. Run Docker Container:
docker pull your-docker-username/nextjs-app:latest
6. Run the Docker container:
docker run -d -p 3000:3000 your-docker-username/nextjs-app:latest
Access your Next.js application through the public IP or DNS of your EC2 instance on port 80 (e.g., http://your-instance-ip).
In summary, Terraform revolutionizes infrastructure management by enabling developers to define and provision cloud resources using simple, declarative configuration files. This Infrastructure as Code (IaC) approach streamlines deployment processes, promotes consistency, and facilitates collaboration. With Terraform, developers can automate infrastructure provisioning, scale applications effortlessly, and focus on delivering value without the complexities of manual setup and maintenance.