Coding Principles for Better Code Quality
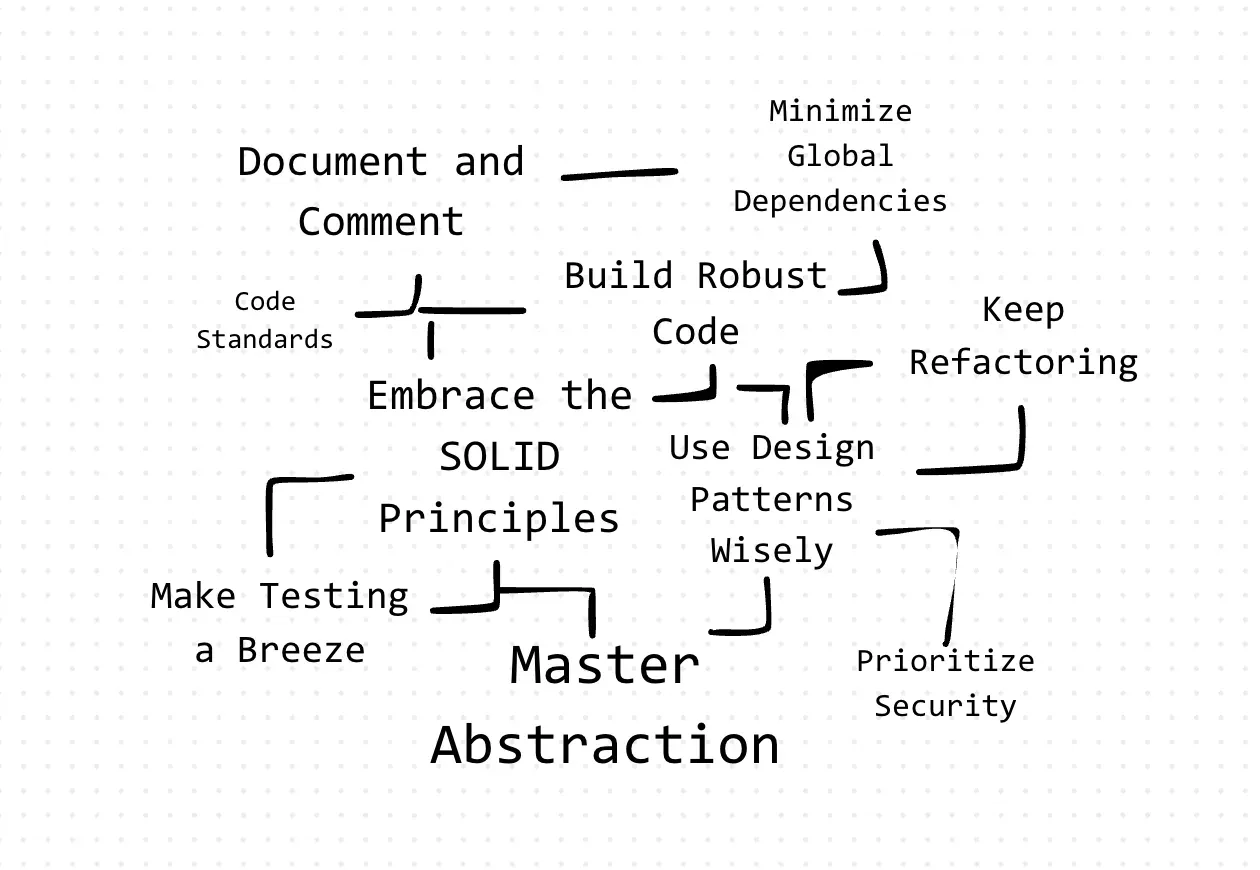
When it comes to software development, writing high-quality code is a must. Following good coding principles can make your code more robust, scalable, and easy to maintain. Here are ten key coding principles to keep in mind as you write your next masterpiece.
1. Stick to Code Standards
Every programming language has its own set of rules for writing clean code. For example, Python has PEP 8, and Java has the Google Java Style Guide. By following these guidelines, your code will be consistent and easier for others to read and maintain.
2. Document and Comment
Clear documentation and comments are lifesavers. They explain the tricky parts of your code and why you made certain decisions. Focus on the "why" rather than the "what" so others (and future you!) can understand your thought process. Keep your documentation up-to-date as your code evolves.
3. Build Robust Code
Robust code handles unexpected inputs and errors gracefully. Make sure your code doesn't crash under pressure by using exception handling and logging. This way, your software remains stable and provides useful feedback when something goes wrong.
4. Embrace the SOLID Principles
The SOLID principles—Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion—are your friends. They help you design software that's easy to scale and maintain. Following these principles makes your code flexible and adaptable.
5. Make Testing a Breeze
Testability is key to good code. Keep your code modular and straightforward to test, so you can catch bugs early and ensure everything works as expected. Automated tests are great for keeping your code in check as it grows and changes.
6. Master Abstraction
Abstraction is about simplifying complex systems by focusing on the essentials. It makes your code more flexible and easier to manage. Strike a balance—avoid over-complicating things, but don't ignore the importance of planning for the future.
7. Use Design Patterns Wisely
Design patterns can solve common problems, but don't overdo it. Each pattern has a specific use case, and overusing them can make your code unnecessarily complex. Use them where they fit best, but keep your code clean and simple.
8. Minimize Global Dependencies
Global variables and instances can lead to confusing state management. Instead, aim for localized state and parameter passing. Keep your functions side-effect-free to make your code more predictable and reliable.
9. Keep Refactoring
Refactoring is all about cleaning up your code as you go. Regularly revisiting and refining your code helps reduce technical debt and makes it more maintainable. Keep an eye out for areas that can be improved, and don't hesitate to make changes.
10. Prioritize Security
Security should always be top of mind. Protect against common vulnerabilities by validating inputs, managing data securely, and using encryption when necessary. Building security into your development process keeps your software and its users safe.
Incorporating these coding principles into your daily workflow can make a huge difference in the quality of your code. As you grow as a developer, remember that good coding practices are a continuous journey rather than a destination. Keep learning, adapting, and sharing your insights with others. By doing so, you'll not only improve your own skills but also contribute to a culture of quality and collaboration in the software development community.