Edge Rendering with Next.js and Cloudflare Workers
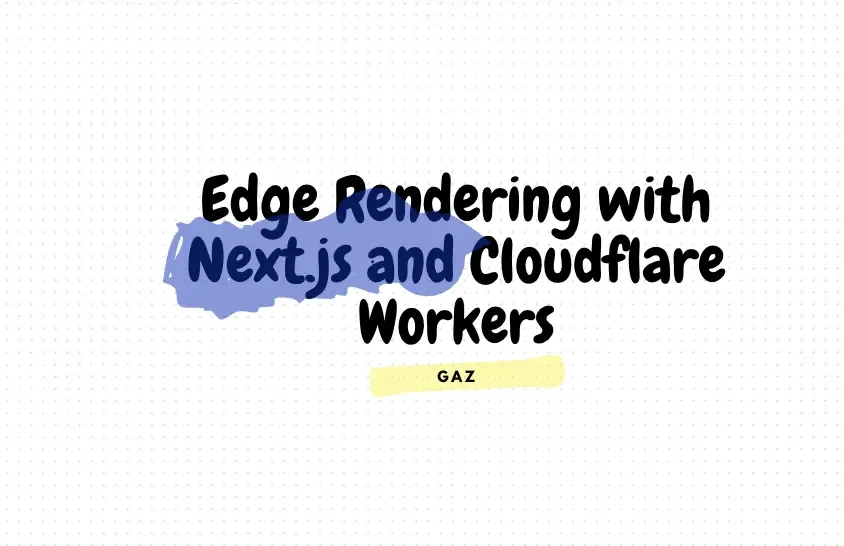
In modern web development, edge rendering is gaining momentum due to its ability to deliver lightning-fast experiences by bringing server-side logic closer to the user. Having recently implemented edge rendering using Next.js and Cloudflare Workers, I want to share my experience and provide a practical guide with TypeScript examples.
Why Edge Rendering?
Edge rendering enables:
- Improved Performance: By processing requests at the edge, latency is reduced significantly.
- Dynamic Personalization: Tailor content for users based on geolocation or other parameters in real-time.
- Reduced Server Load: Offload tasks to edge nodes, freeing up central servers.
Getting Started with Next.js
To implement edge rendering, you need Next.js 12 or later, as it introduced support for middleware, which can be deployed to the edge.
Step 1: Create a New Next.js Project
Start by creating a new Next.js application if you don’t have one:
npx create-next-app@latest next-edge-example
cd next-edge-example
npm install
Step 2: Add Middleware for Edge Processing
Middleware in Next.js allows you to run code before a request is completed. Let’s create a middleware file:
import { NextRequest, NextResponse } from 'next/server';
export function middleware(req: NextRequest) {
const url = req.nextUrl.clone();
// Example: Redirect based on geolocation
const country = req.geo?.country || 'US';
if (country !== 'US') {
url.pathname = `/international${url.pathname}`;
return NextResponse.redirect(url);
}
return NextResponse.next();
}
export const config = {
matcher: '/:path*', // Apply middleware to all routes
};
Step 3: Deploy Middleware to the Edge
In your next.config.js, enable experimental edge runtime:
module.exports = {
experimental: {
runtime: 'edge',
},
};
Next.js middleware can now run on platforms supporting edge runtimes, such as Cloudflare Workers.
Deploying to Cloudflare Workers
Cloudflare Workers is an ideal platform for edge rendering due to its global distribution and performance.
Step 1: Install Wrangler CLI
Wrangler is Cloudflare’s CLI for managing Workers.
npm install -g wrangler
Step 2: Configure Cloudflare Project
Initialize a Cloudflare Workers project:
wrangler init
Modify the wrangler.toml file to configure your project:
name = "next-edge-example"
main = "middleware.ts"
compatibility_date = "2024-01-01"
Step 3: Build and Deploy
Compile your project and deploy it to Cloudflare Workers:
wrangler build
wrangler publish
Step 4: Configure DNS
Point your domain or subdomain to Cloudflare and route traffic to your deployed Worker.
Example: Dynamic Rendering with TypeScript
Here’s how you can use edge rendering to deliver dynamic content based on geolocation:
import { useEffect, useState } from 'react';
interface GeoData {
country: string;
city?: string;
}
export default function Home() {
const [geo, setGeo] = useState<GeoData | null>(null);
useEffect(() => {
fetch('/api/geo')
.then((res) => res.json())
.then((data) => setGeo(data));
}, []);
return (
<div>
<h1>Welcome to Edge Rendering with Next.js!</h1>
{geo && (
<p>
You are visiting from {geo.city || 'an unknown city'}, {geo.country}.
</p>
)}
</div>
);
}
pages/api/geo.ts
import { NextApiRequest, NextApiResponse } from 'next';
export default function handler(req: NextApiRequest, res: NextApiResponse) {
const geo = {
country: req.headers['x-country'] || 'US',
city: req.headers['x-city'],
};
res.status(200).json(geo);
}
Conclusion
Edge rendering with Next.js and Cloudflare Workers is a powerful combination for delivering fast, dynamic, and scalable web applications. By leveraging middleware, TypeScript, and edge computing platforms, you can enhance the user experience while optimizing backend performance.
I hope this guide helps you get started with edge rendering. Feel free to share your thoughts or ask questions below!