Elusive Least Occurring Number in an Array
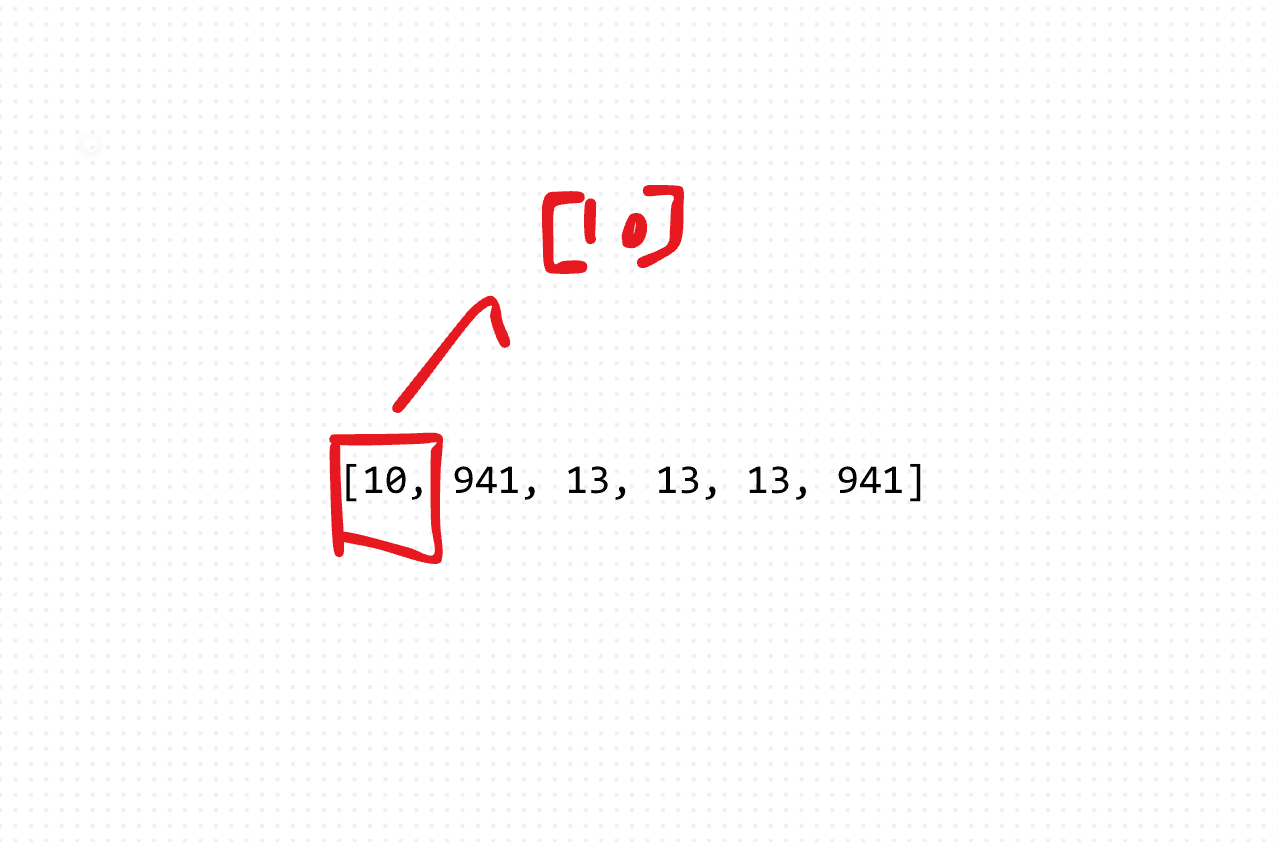
Consider an array of integers representing various data points. The objective is to identify the number that occurs the least number of times in the array. This least occurring number holds significance in scenarios such as anomaly detection, outlier analysis, or resource allocation optimization.
const solution = (numbers) => {
const counts = new Map();
for (const num of numbers) {
counts.set(num, (counts.get(num) || 0) + 1);
}
const minCount = Math.min(...counts.values());
const leastOccurringNumbers = [];
for (const [num, count] of counts) {
if (count === minCount) {
leastOccurringNumbers.push(num);
}
}
return leastOccurringNumbers;
};
console.log(solution([10, 941, 13, 13, 13, 941])); // Output: [10]
By employing a combination of data structures and iterative techniques, we've successfully addressed the problem of identifying the least occurring number in an array. This solution provides a versatile approach that can be applied in various data analysis and optimization scenarios, showcasing the power of algorithmic problem-solving techniques in real-world applications.