String Reversal Algorithm
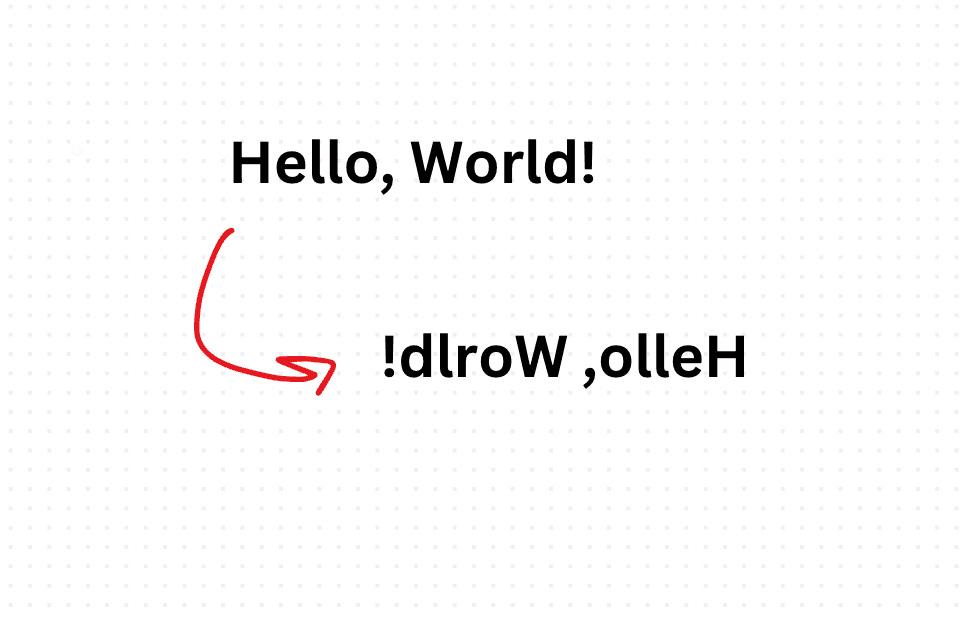
String reversal is a fundamental operation in computer science used in various applications, including text processing, cryptography, and data compression
String reversal involves reversing the order of characters in a given string. It is a simple yet essential operation with applications in many areas of computer science, ranging from basic text manipulation to advanced data processing tasks.
- Iterative Reversal: Iterate through the string and build the reversed string character by character.
// Iterative Reversal
function reverseStringIterative(str) {
let reversed = '';
for (let i = str.length - 1; i >= 0; i--) {
reversed += str[i];
}
return reversed;
}
- Recursive Reversal: Recursively reverse substrings of the input string.
// Recursive Reversal
function reverseStringRecursive(str) {
if (str === '') {
return '';
} else {
return reverseStringRecursive(str.substr(1)) + str.charAt(0);
}
}
- Using Built-in Functions: Utilize built-in JavaScript functions like split, reverse, and join to reverse the string efficiently.
// Using Built-in Functions
function reverseStringBuiltIn(str) {
return str.split('').reverse().join('');
}
The time complexity of all three approaches to string reversal is O(n), where n is the length of the input string. Each character of the string is processed once during the reversal operation.