Minimum Size Subarray Sum Algorithm
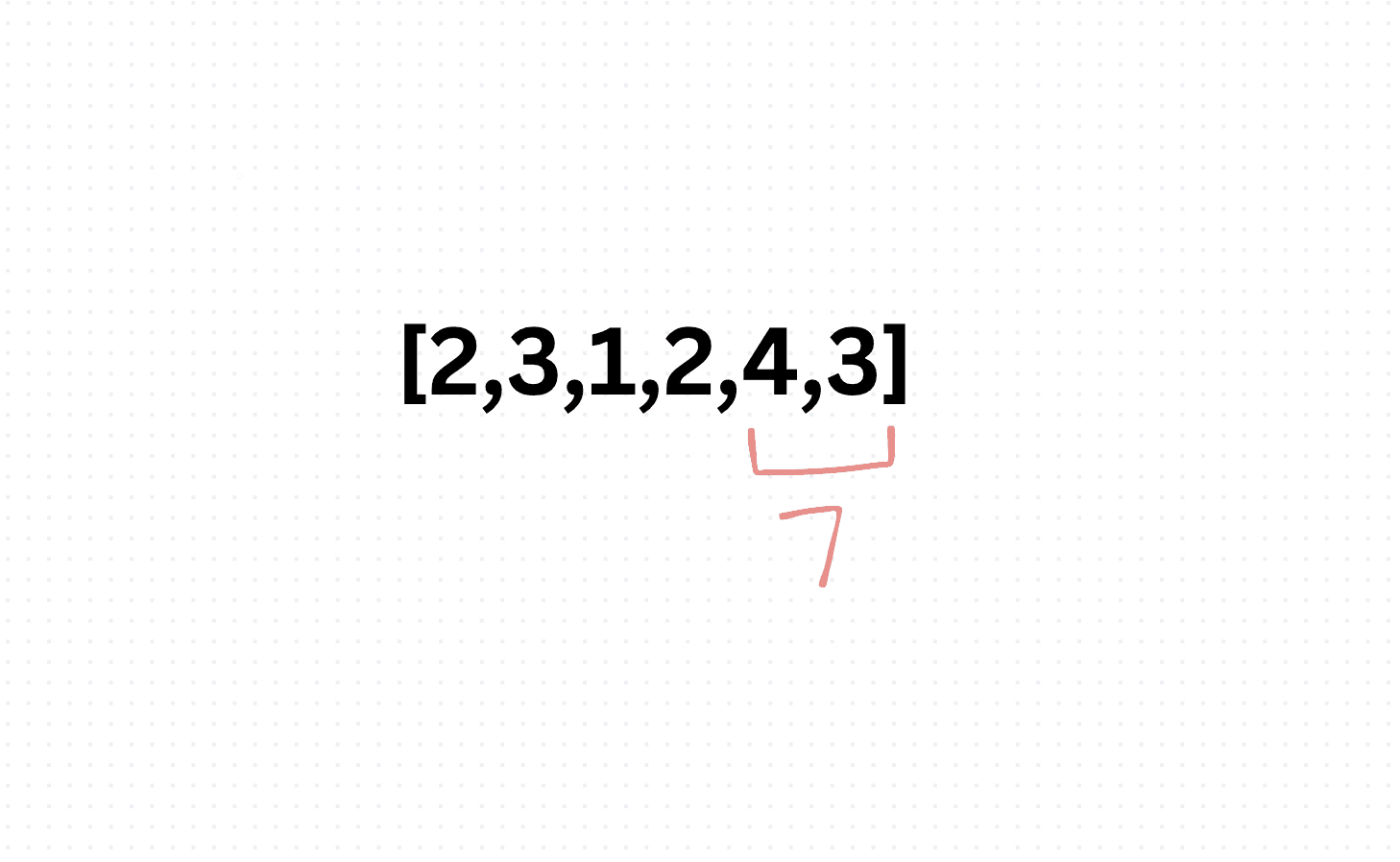
Given an array of positive integer nums and a positive integer target, return the minimal length of a
subarray whose sum is greater than or equal to the target. If there is no such subarray, return 0 instead.
Input: target = 7, nums = [2,3,1,2,4,3] Output: 2 Explanation: The subarray [4,3] has the minimal length under the problem constraint.
var minSubArrayLen = function(target, nums) {
const n = nums.length;
let left = 0;
let sum = 0;
let minLength = Infinity
for (let right = 0; right < nums.length; right++) {
sum += nums[right]
while (sum >= target) {
const currentLength = right - left + 1;
minLength = Math.min(minLength,currentLength)
sum -= nums[left]
left++;
}
}
if (minLength === Infinity) return 0;
return minLength;
};